Find the height of binary tree in C
Height of binary tree
A binary tree is a tree-type non-linear data structure with a maximum of two children for each parent. The height of a binary tree is defined as the number of edges between the root node and the farthest leaf node.The height of an empty tree is 0.
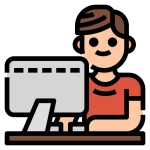
Find Height of Binary Tree In C Language
Algorithm :
- If root is NULL, return 0.
- Otherwise Recursively call the height function on its left and right child.
- Return the value which is greater out of height of left child and bight of right child
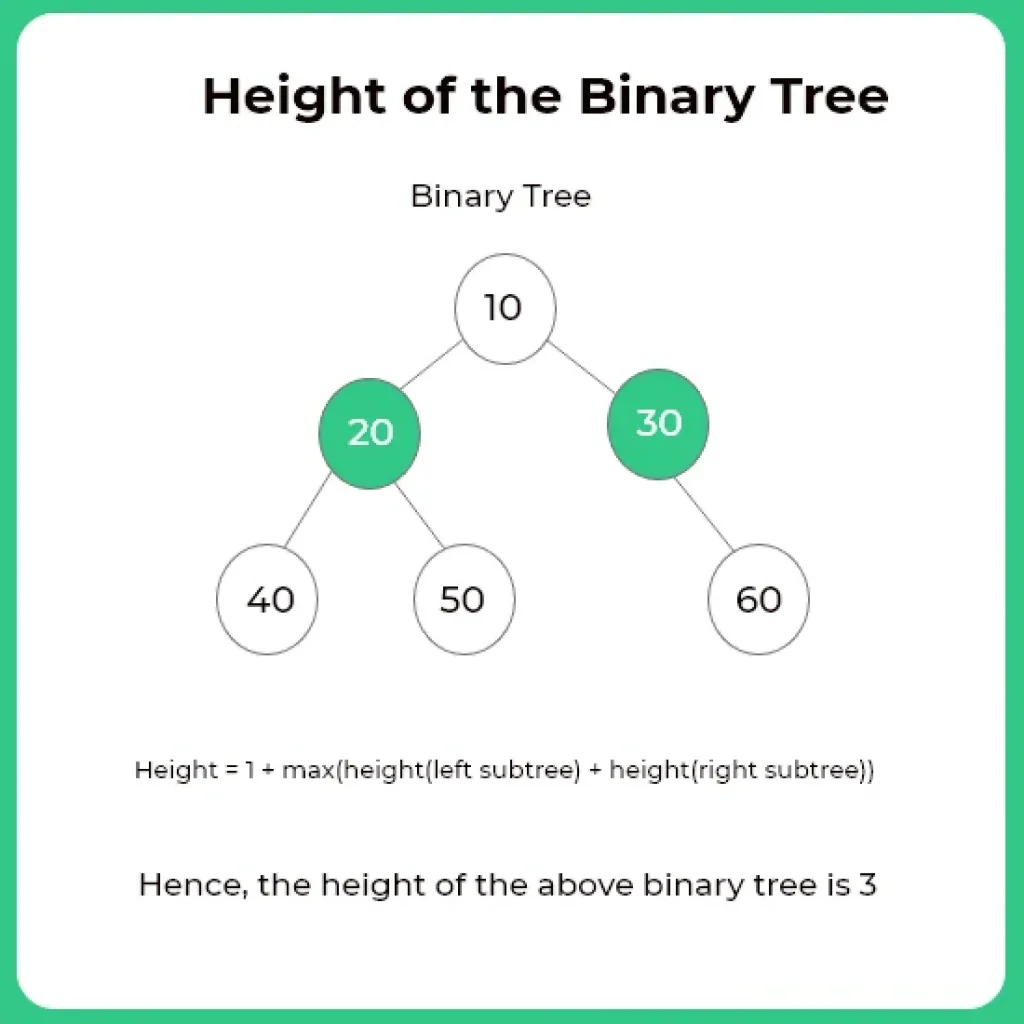
Code For Find The Height of Binary Tree In C
Run
#include <stdio.h> #include <stdlib.h> struct node { int data; struct node *left; struct node *right; }; int height (struct node *node) { if (node == NULL) return 0; else { int leftHeight = height (node->left); int rightHeight = height (node->right); if (leftHeight > rightHeight) return (leftHeight + 1); else return (rightHeight + 1); } } struct node *newNode (int data) { struct node *node = (struct node *) malloc (sizeof (struct node)); node->data = data; node->left = NULL; node->right = NULL; return (node); } int main () { struct node *root = newNode (10); root->left = newNode (20); root->right = newNode (30); root->left->left = newNode (40); root->left->right = newNode (50); printf ("Height of tree is %d", height (root)); return 0; }
Output:
Height of tree is 3
Login/Signup to comment