Fibonacci Series in Python
Find the Fibonacci Series up to Nth Term in Python
Given an integer as an input, the objective is to find the Fibonacci series until the number input as the Nth term. Therefore, we write a program to Find the Fibonacci Series up to Nth Term in Python Language.
Example Input : 4 Output : 0 1 1 2
Lets have a look at write a program to print fibonacci series in python
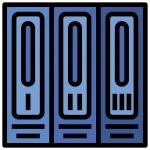
Where the first two terms are always 0 and 1
Where,
F0 : 0
F1 : 1
Find the Fibonacci Series up to Nth Term in Python Language
Given an integer input as the Nth value, the objective is to Find the Fibonacci Series up to the Nth Term using Loops and Recursion. The objective is to print all the number of the Fibonacci series until the Nth term given as an input. Here are some of the methods to solve the above mentioned problem
- Method 1: Using Simple Iteration
- Method 2: Using Recursive Function
- Method 3: Using direct formulae
We’ll discuss the above mentioned methods in detail in the sections below. Do check out the blue box below for better understanding of fibonacci series program in python.
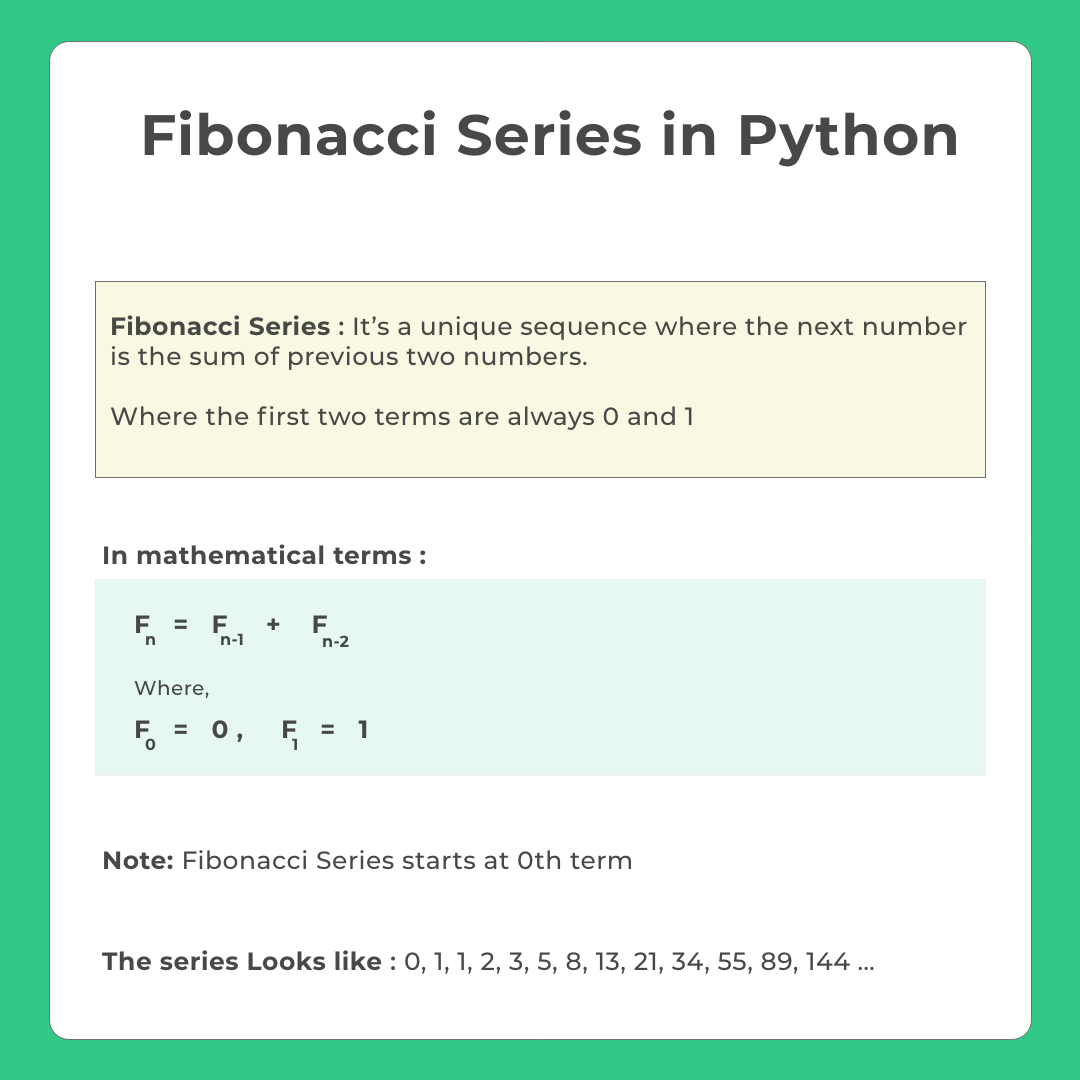
Method 1: Using Simple Iteration
In this method we’ll use loops to iterate through and form the series up to the integer input N as the range. To print the series up to the Nth term we start a loop from 2 to the Nth term as 0 and 1 are the seed values for forming the series.
Let’s implement the logic in Python Language.
# Write a program to print fibonacci series upto n terms in python num = 10 n1, n2 = 0, 1 print("Fibonacci Series:", n1, n2, end=" ") for i in range(2, num): n3 = n1 + n2 n1 = n2 n2 = n3 print(n3, end=" ") print()
Python Code
Output
Fibonacci Series: 0 1 1 2 3 5 8 13 21 34
Method 2: Using Recursive Function
In this method we’ll use recursion to find the Fibonacci Series up to the given integer input as the Nth range. To do so we take three variables and call the recursive function twice in return statement forming a recursion tree. To know more about recursion, Check out our page, Recursion in Python.
Let’s implement the above mentioned logic in Python Language.
Python Code
# Python program to print Fibonacci Series def fibonacciSeries(i): if i <= 1: return i else: return (fibonacciSeries(i - 1) + fibonacciSeries(i - 2)) num=10 if num <=0: print("Please enter a Positive Number") else: print("Fibonacci Series:", end=" ") for i in range(num): print(fibonacciSeries(i), end=" ")
Output
Fibonacci Series: 0 1 1 2 3 5 8 13 21 34
Method 3
We can use direct formula to find nth term of Fibonacci Series as –
Fn = {[(√5 + 1)/2] ^ n} / √5
# write a program to print fibonacci series in python import math def fibonacciSeries(phi, n): for i in range(0, n + 1): result = round(pow(phi, i) / math.sqrt(5)) print(result, end=" ") num = 10 phi = (1 + math.sqrt(5)) / 2 fibonacciSeries(phi, num)
Output
Fibonacci Series:0 1 1 2 3 5 8 13 21 34 55
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
- Positive or Negative number: C | C++ | Java | Python
- Even or Odd number: C | C++ | Java | Python
- Sum of First N Natural numbers: C | C++ | Java | Python
- Sum of N natural numbers: C | C++ | Java | Python
- Sum of numbers in a given range: C | C++ | Java | Python
- Greatest of two numbers: C | C++ | Java | Python
- Greatest of the Three numbers: C | C++ | Java | Python
- Leap year or not: C | C++ | Java | Python
- Prime number: C | C++ | Java | Python
- Prime number within a given range: C | C++ | Java | Python
- Sum of digits of a number: C | C++ | Java | Python
- Reverse of a number : C | C++ | Java | Python
- Palindrome number: C | C++ | Java | Python
- Armstrong number : C | C++ | Java | Python
- Armstrong number in a given range : C | C++ | Java | Python
- Fibonacci Series upto nth term : C | C++ | Java | Python
- Find the Nth Term of the Fibonacci Series : C | C++ | Java | Python
- Factorial of a number : C | C++ | Java | Python
- Power of a number : C | C++ | Java | Python
- Factor of a number : C | C++ | Java | Python
- Strong number : C | C++ | Java | Python
- Perfect number : C | C++ | Java | Python
- Automorphic number : C | C++ | Java | Python
- Harshad number : C | C++ | Java | Python
- Abundant number : C| C++ | Java | Python
- Friendly pair : C | C++ | Java | Python
n = int(input(“enter the number : “))
temp = n
m = [0, 1]
for i in range (1, temp+1):
m.append(m[-1] + m[-2])
print(m)
Dynamic approach for fibonacci series in python:
memo=[] #create an empty list
def fibo(n):
for j in range(n+1):
memo.append(-1)
#append items to list
for i in range(len(memo)):
#looping through the index of the list
if i==0:
memo[0]=1
if i==1:
memo[1]=1
#assign first 2 values
for i in range(2, n+1):
memo[i]= memo[i-1] + memo[i – 2]
return memo
print(fibo(6))
m = [0, 1]
for j in range (1, 10+1):
m.append(m[-2] + m[-1])
print(m)
number=10
n1=0
n2=1
print(“Fibonacci Series”,n1,n2,end=” “)
for i in range(number-2):
n3=n1+n2
n1,n2=n2,n3
print(n3,end=” “)
if number<=0:
print("Please enter the number greater than 0")
else:
for i in range(0,number):
print(sum, end=" ")
n1 = n2
n2 = sum
sum = n1 + n2
n=int(input())
list=[]
def fib(n):
if n==0 or n==1:
return n
else:
return (fib(n-1)+fib(n-2))
for i in range(n):
result=fib(i)
list.append(result)
print(list)
n1=int(input(“enter index number:”))
print(list[n1])
def fibonacciSeries(i):
if i <= 1:
return i
else:
return (fibonacciSeries(i – 1) + fibonacciSeries(i – 2))
num=int(input("Enter a number:"))
if num <= 0:
print("Please enter a Positive Number")
else:
print("Fibonacci Series:", end=" ")
for i in range(num):
print(fibonacciSeries(i), end=" ")
num1=int(input(“enter the value”))
fib1=int(0)
fib2=int(1)
for i in range(num1):
print(fib1,end=” “)
fibn=fib1+fib2
fib1=fib2
fib2=fibn