Fibonacci Series Program in C
Write a C Program to find Fibonacci series up to n
The sequence is a Fibonacci series in C where the next number is the sum of the previous two numbers. The first two terms of the Fibonacci sequence is started from 0, 1.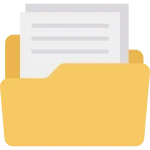
What is Fibonacci Series
It’s a unique sequence where the next number is the sum of previous two numbers.
Where the first two terms are always 0 and 1
Where the first two terms are always 0 and 1
In mathematical terms :
Fn = Fn-1 + Fn-2
Where,
F0 : 0
F1 : 1
Where,
F0 : 0
F1 : 1
Example Series
The series Looks like : 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144 …
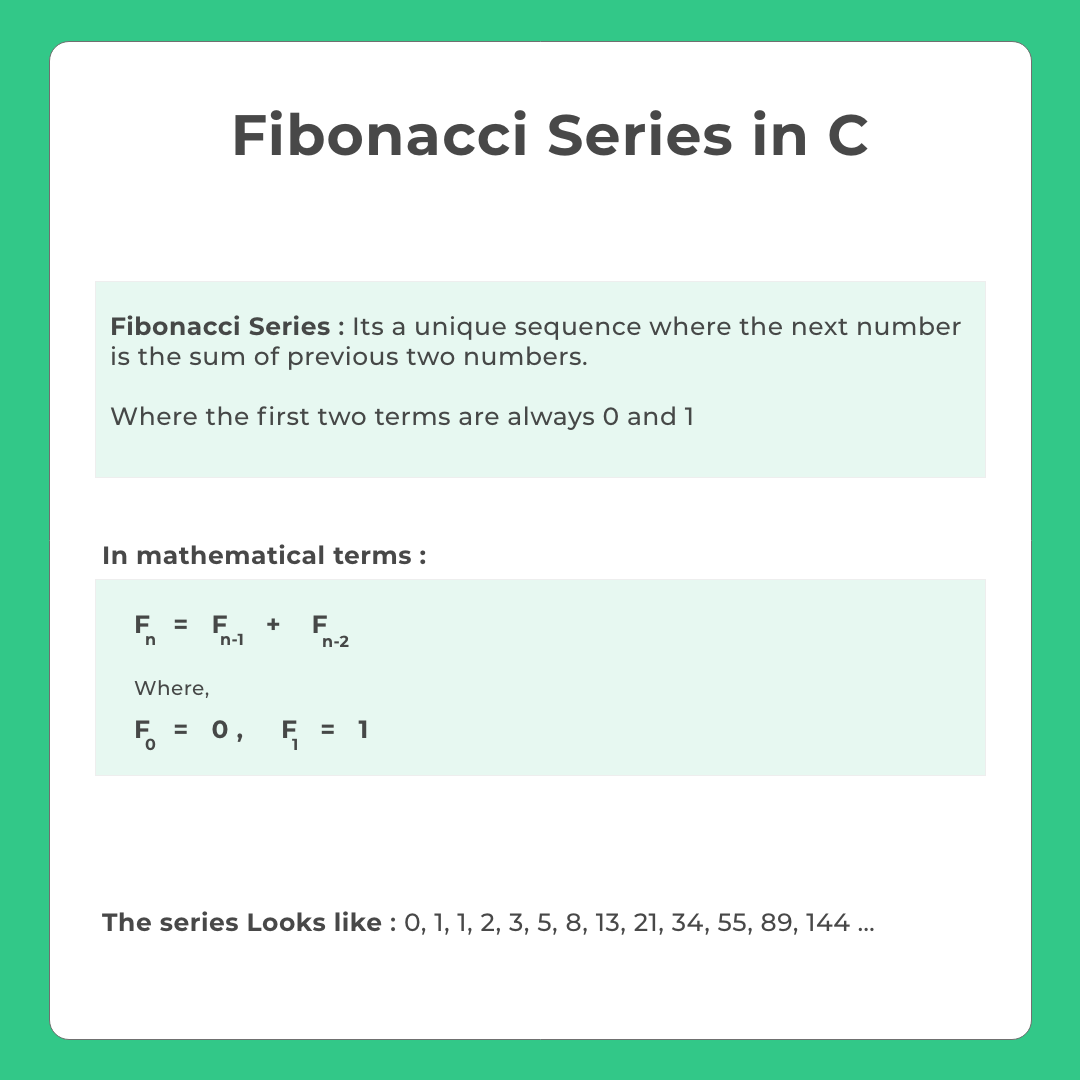
Method 1
Write a program to print Fibonacci series method 1 below –
Run
#include<stdio.h> int main() { int n = 10; int a = 0, b = 1; // printing the 0th and 1st term printf("%d, %d",a,b); int nextTerm; // printing the rest of the terms here for(int i = 2; i < n; i++){ nextTerm = a + b; a = b; b = nextTerm; printf("%d, ",nextTerm); } return 0; }
Output
0, 1, 1, 2, 3, 5, 8, 13, 21, 34,
Method 2 (Recursion/static variable)
This method uses recursion in C, also uses static variables in C
Run
#include<stdio.h> int printFib(int n){ static int a = 0, b = 1, nextTerm; if(n > 0) { nextTerm = a + b; a = b; b = nextTerm; printf("%d, ",nextTerm); printFib(n-1); } } int main() { int n = 10; printf("0, 1, "); // n-2 as 2 terms already printed printFib(n-2); return 0; }
Output
0, 1, 1, 2, 3, 5, 8, 13, 21, 34,
Method 3 (Recursion)
This method uses recursion in C, rather than static variables, we use pure recursion here.
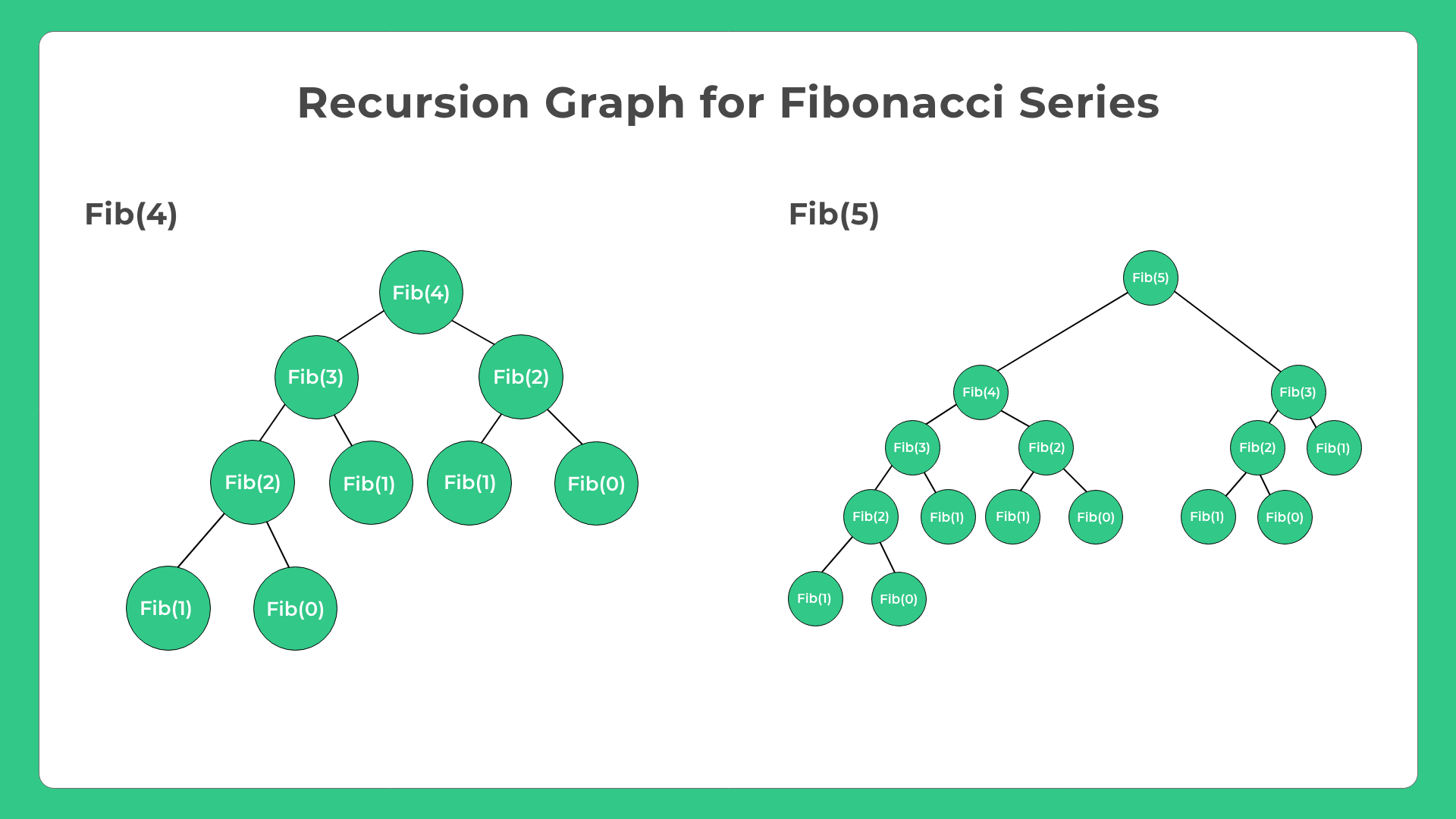
#include<stdio.h> int getFib(int n){ if(n <= 1) return n; return getFib(n-1) + getFib(n-2); } int main() { int n = 10; for(int i = 0; i < n; i++) printf("%d, ",getFib(i)); return 0; }
Output
0, 1, 1, 2, 3, 5, 8, 13, 21, 34,
Method 4 (Direct Formula)
We can use direct formula to find nth term of Fibonacci Series as –
Fn = {[(√5 + 1)/2] ^ n} / √5
#include<stdio.h> #include<math.h> int getFibo(double phi, int n) { for(int i = 0; i <= n; i++) { // setting .0 precision so all leading decimals are not printed // else would have printed 1.000000, 1.000000, 2.000000 so on.... printf("%.0lf, ",round(pow(phi, i) / sqrt(5))); } } int main () { int n = 15; double phi = (1 + sqrt(5)) / 2; getFibo(phi, n); return 0; }
Output
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610,
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
- Positive or Negative number: C | C++ | Java | Python
- Even or Odd number: C | C++ | Java | Python
- Sum of First N Natural numbers: C | C++ | Java | Python
- Sum of N natural numbers: C | C++ | Java | Python
- Sum of numbers in a given range: C | C++ | Java | Python
- Greatest of two numbers: C | C++ | Java | Python
- Greatest of the Three numbers: C | C++ | Java | Python
- Leap year or not: C | C++ | Java | Python
- Prime number: C | C++ | Java | Python
- Prime number within a given range: C | C++ | Java | Python
- Sum of digits of a number: C | C++ | Java | Python
- Reverse of a number : C | C++ | Java | Python
- Palindrome number: C | C++ | Java | Python
- Armstrong number : C | C++ | Java | Python
- Armstrong number in a given range : C | C++ | Java | Python
- Fibonacci Series upto nth term : C | C++ | Java | Python
- Find the Nth Term of the Fibonacci Series : C | C++ | Java | Python
- Factorial of a number : C | C++ | Java | Python
- Power of a number : C | C++ | Java | Python
- Factor of a number : C | C++ | Java | Python
- Strong number : C | C++ | Java | Python
- Perfect number : C | C++ | Java | Python
- Automorphic number : C | C++ | Java | Python
- Harshad number : C | C++ | Java | Python
- Abundant number : C| C++ | Java | Python
- Friendly pair : C | C++ | Java | Python
#include
int main()
{
int n2,a=0,b=1,sum=0,i=3;
printf(“Enter length of fibonacci series required :”);
scanf(“%d”,&n2);
printf(“%d %d “,a,b);
while(i<=n2)
{
sum = a+b;
printf("%d ",sum);
a = b;
b = sum;
i++;
};
return 0;
}
Kindly join our Discord channel for all the course related or technical queries.
#include
int main(){
int n,i,a=0,b=1,c;
printf(“Enter a number: “);
scanf(“%d”,&n);
if(n<0){
printf("Number of terms should be always greater than 0\n");
}
else{
for(i=1;i<=n;i++){
printf("%d ",a);
c=a+b;
a=b;
b=c;
}
}
}