Automorphic Number or Not | C Program
Automorphic Number in C
In this program, we have to find the number is an Automorphic number or not using C programming. Basically, automorphic number is a number whose square ends with the same digits as number itself.
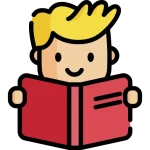
Automorphic Number in C Programming
Example:
5 =(5)2 = 25 6 = (6)2 = 36 25 = (25)2 = 625 76=(76)2 = 5776 376 = (376)2 = 141376 890625 = (890625)2 = 793212890625
These numbers are the automorphic numbers.
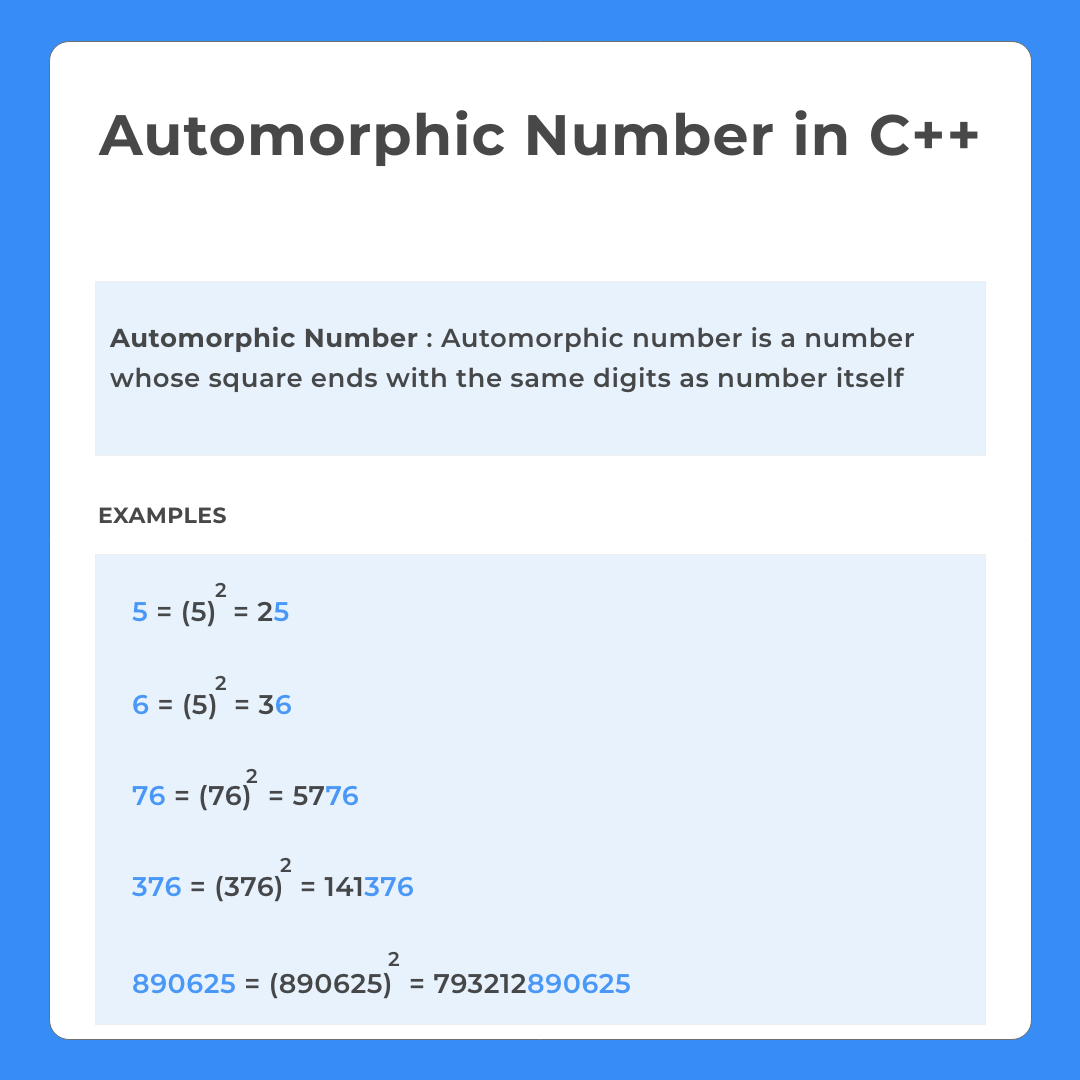
C Program:-
Run
#include <stdio.h> int checkAutomorphic(int num){ int square = num * num; while(num != 0) { // means not automorphic number if(num % 10 != square % 10){ return 0; } // reduce down numbers num /= 10; square /= 10; } // if reaches here means automorphic number return 1; } int main () { int num = 376, square = num * num ; if(checkAutomorphic(num)) printf("Num : %d, Square: %d - Automorphic Number",num, square); else printf("Num : %d, Square: %d - Not Automorphic Number",num, square); } // Time complexity: O(N) // Space complexity: O(1)
Output:-
Num : 376, Square: 141376 - Automorphic Number
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
- Positive or Negative number: C | C++ | Java | Python
- Even or Odd number: C | C++ | Java | Python
- Sum of First N Natural numbers: C | C++ | Java | Python
- Sum of N natural numbers: C | C++ | Java | Python
- Sum of numbers in a given range: C | C++ | Java | Python
- Greatest of two numbers: C | C++ | Java | Python
- Greatest of the Three numbers: C | C++ | Java | Python
- Leap year or not: C | C++ | Java | Python
- Prime number: C | C++ | Java | Python
- Prime number within a given range: C | C++ | Java | Python
- Sum of digits of a number: C | C++ | Java | Python
- Reverse of a number : C | C++ | Java | Python
- Palindrome number: C | C++ | Java | Python
- Armstrong number : C | C++ | Java | Python
- Armstrong number in a given range : C | C++ | Java | Python
- Fibonacci Series upto nth term : C | C++ | Java | Python
- Find the Nth Term of the Fibonacci Series : C | C++ | Java | Python
- Factorial of a number : C | C++ | Java | Python
- Power of a number : C | C++ | Java | Python
- Factor of a number : C | C++ | Java | Python
- Strong number : C | C++ | Java | Python
- Perfect number : C | C++ | Java | Python
- Automorphic number : C | C++ | Java | Python
- Harshad number : C | C++ | Java | Python
- Abundant number : C| C++ | Java | Python
- Friendly pair : C | C++ | Java | Python
#include
#include
int main()
{
int number = 25;
int count=0;
int ans = number * number; // 625
int orig = number;
int result=0;
while(number>0)
{
count++;
number = number /10;
}
for(int i=0;i<count;i++)
{
int remainder = ans%10;
result = remainder * pow(10, i) + result;
ans=ans/10;
}
printf("%d",result);
if(orig == result)
{
printf("It is an automorphic number");
}
else{
printf("Not an automorphic number");
}
}
Kindly join our discord community for all the technical support.
#PYTHON is cool
n = input()
print(“YES” if int(str(n**2)[-len(str(n))::]) == n else “No”)