OS Menu
- OS Home
- Introduction
- CPU Scheduling
- What is Process?
- Process Lifecyle
- Process Control Block
- Process Scheduling
- Context Switching
- CPU Scheduling
- FCFS Scheduling
- SJF (non-preemptive)
- SJF (Preemptive - SRTF)
- Round Robin
- Priority Scheduling
- Convoy Effect
- Scheduler Vs Dispatcher
- Preemptive Vs non
- Preemptive scheduling
- Non preemptive scheduling
- Process Synchronization
- Deadlock
- Popular Algorithms
- Memory Management
- Memory Management Introduction
- Partition Allocation Method
- First Fit
- First Fit (Intro)
- First Fit in C
- First Fit in C++
- First Fit in Python
- First Fit in Java
- Best Fit
- Best Fit (Intro)
- Best Fit in C
- Best Fit in C++
- Best Fit in Java
- Worst Fit
- Worst Fit (Intro)
- Worst Fit in C++
- Worst Fit in C
- Worst Fit in Java
- Worst Fit in Python
- Next Fit
- First fit best fit worst fit (Example)
- Memory Management 2
- Memory Management 3
- Page Replacement Algorithms
- LRU (Intro)
- LRU in C++
- LRU in Java
- LRU in Python
- FIFO
- Optimal Page Replacement algorithm
- Optimal Page Replacement (Intro)
- Optimal Page Replacement Algo in C
- Optimal Page Replacement Algo in C++
- Optimal Page Replacement Algo in Java
- Optimal Page Replacement Algo in Python
- Thrashing
- Belady’s Anomaly
- Static vs Dynamic Loading
- Static vs Dynamic Linking
- Swapping
- Translational Look Aside Buffer
- Process Address Space
- Difference between Segmentation and Paging
- File System
- Off-campus Drive Updates
- Get Hiring Updates
- Contact us
PREPINSTA PRIME
Worst Fit Program In Python
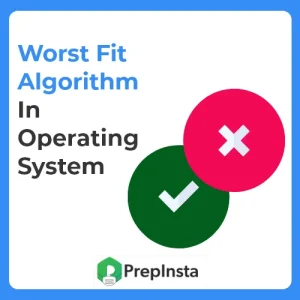
Worst Fit Program in Python
The operating system is responsible to allocate memory to the different processes under execution. The empty memory is allocated to these processes at dynamic time on the basis of different memory management schemes. The most common schemes among them are the best fit, worst fit, and the first fit.
The operating system is responsible to allocate memory to the different processes under execution. The empty memory is allocated to these processes at dynamic time on the basis of different memory management schemes. The most common schemes among them are the best fit, worst fit, and the first fit.
In the case of worst fit memory allocation method, the CPU searches for the empty memory block which is equal or greater than the memory size demanded by the process. The empty memory block is allocated to the process as soon as it is found. The allocation scheme is said to be the worst fit method as it sometimes causes maximum wastage of valuable memory space. An aerating system allocates this memory based using an algorithm also known as the scheduling algorithm.
- Read Also – Worst Fit Algorithm in JAVA
Program code for Worst Fit Memory Management Scheme using Python:
# Method to assign empty memory to processes using the worst fit algorithm def worstfit(b_size, m, p_size, n): #code to store the memory block id during the allocation process # No code is allocated at the initial stage allocate = [-1] * n #select each process and search for an empty memory block as per its memory demand for i in range(n): # Find an empty memory block for the current process wstIdx = -1 for j in range(m): if b_size[j] >= p_size[i]: if wstIdx == -1: wstIdx = j elif b_size[wstIdx] < b_size[j]: wstIdx = j #code to find an empty block for the current process if wstIdx != -1: # allocating empty memory space j to p[i] process allocate[i] = wstIdx # Reduce available memory in this block. b_size[wstIdx] -= p_size[i] print ("Process Number Process Size Block Number") for i in range(n): print (i + 1, " ", p_size[i], end = " ") if allocate[i] != -1: print(allocate[i] + 1) else: print ("Not Allocated") # Driver code if __name__ == '__main__': b_size = [100, 500, 200, 300, 600] p_size = [212, 417, 112, 426] m = len(b_size) n = len(p_size) worstfit(b_size, m, p_size, n)
Output
[table id=679 /]
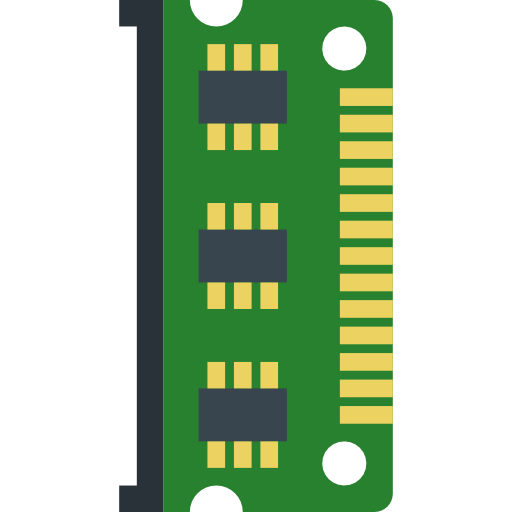
Read More
- Memory Management Introduction
- Partition Allocation Method
- Buddy- System Allocator
- Paging
- Types of Paging
- Fragmentation
- Mapping Virtual address to Physical Address.
- Virtual Memory
- Demand Paging
- Implementation of Demand paging and page fault
- Segmentation
- Page Replacement Algorithms
- Thrashing
- Belady’s Anomaly
- Static vs Dynamic Loading
- Static vs Dynamic Linking
- Swapping
- Translational Look Aside Buffer
- Process Address Space
- Difference between Segmentation and Paging
Login/Signup to comment