Reverse a Number in Python
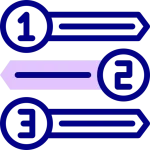
Find the Reverse of a Number in Python
Given an integer input the objective is to reverse the given integer number using loops and slicing. Therefore, we’ll write a program to Find the Reverse of a Number in Python Language.
Example Input : 123 Output : 321
Find the Reverse of a Number in Python Language
We need to write a python code to reverse the given integer and print the integer. The typical method is to use modulo and divide operators to break down the number and reassemble again in the reverse order. Here are some of the methods to solve the above mentioned problems,
- Method 1: Using Simple Iteration
- Method 2: Using String Slicing
- Method 3: Using Recursion
Let’s go through the above mentioned methods in the sections below.
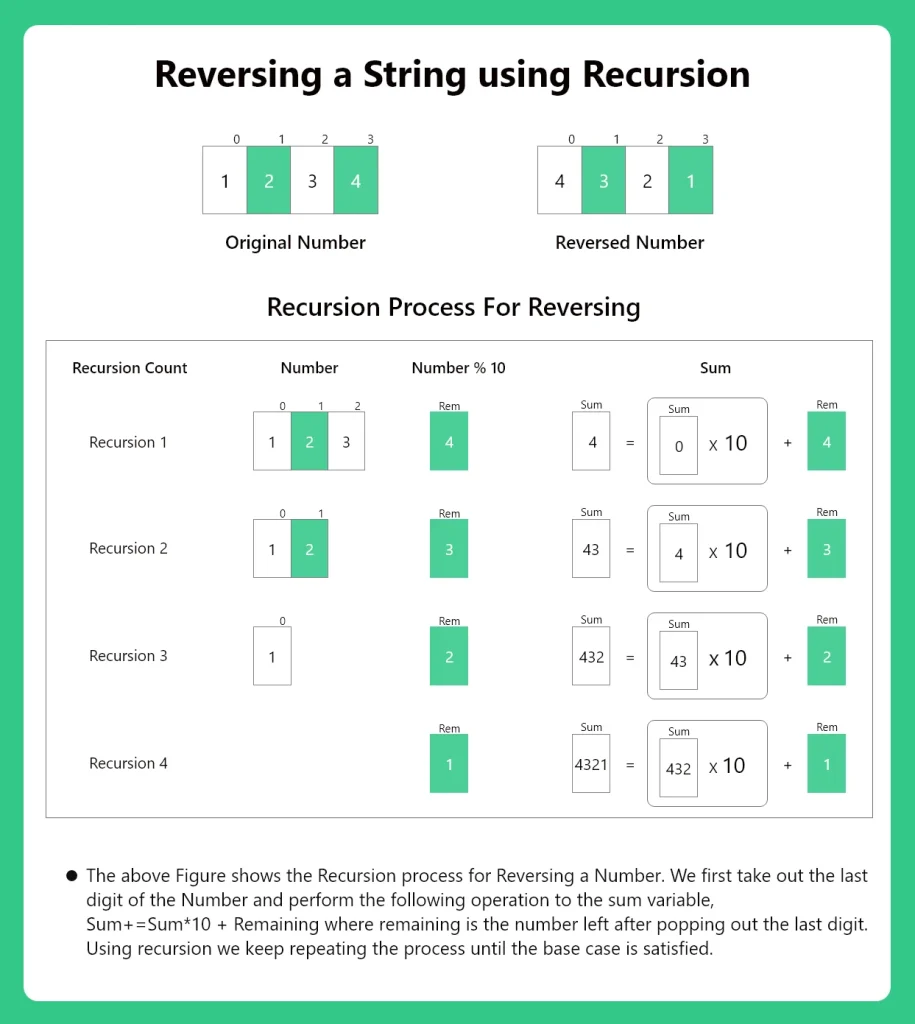
Method 1: Using Simple Iteration
Working
In this method we’ll use the concept of loops to repeat the process of breaking down the number and adding it back in the reverse order.
For a given integer variable number we perform the following,
- Run a while loop with the condition number >0.
- Extract the last digit as Remainder using Modulo operator.
- Using the formula reverse = ( reverse * 10 ) + remainder , we keep changing the reverse value.
- Break down the Nunber using divide operator.
- Print the reverse variable.
Let’s implement the above mentioned Logic in Python Language.
Python Code
num = 1234 temp = num reverse = 0 while num > 0: remainder = num % 10 reverse = (reverse * 10) + remainder num = num // 10 print(reverse)
Output
4321
Method 2: Using String Slicing
Working
In this method we’ll use the string slicing methods to reverse the number. First we’ll convert the number into a string and then using the string slicing property in python language, we’ll slice the string to break it down and add it up in reverse order.
For a given integer input, we perform the following operations,
- Convert the number into string format.
- Reverse the number using string slicing.
Let’s implement the above mentioned logic in Python Language.
Python Code
num = 1234 print(str(num)[::-1])
Output
4321
Method 3: Using Recursion
Working
In this method we’ll use recursion to perform all the operations we perform in method 1. We’ll use the same formula but instead of iterating through a loop, we’ll use recursion. To know more about recursion, check out our page Recursion in Python.
Given an integer input number, we perform the following,
- Define a recursive function recursum() that takes in number and reverse variable as arguments.
- Set the base case as number == 0 and step recursive call as recursum(num/10, reverse).
- Print the returned value using print() function.
Let’s implement the above mentioned logic in Python Language.
Python Code
def recursum(number,reverse): if number==0: return reverse remainder = int(number%10) reverse = (reverse*10)+remainder return recursum(int(number/10),reverse) num = 1234 reverse = 0 print(recursum(num,reverse))
Output
4321
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
#Using Slicing
def reverse_number_string_slicing(n):
return int(str(n)[::-1])
print(reverse_number_string_slicing(12345))
Hey there,
Kindly join our Discord server for all your subject related queries.
def Reverse_N(x:int) -> int:
a = ”
for _ in range(len(str(x))):
digit = int(x%10)
a+=str(digit)
x = x // 10
return a
v = 123450894750000
if __name__ == “__main__”:
print(Reverse_N(v))
# 11. Find the Reverse of a Number in Python.
a=str(123)
x=-1
for i in a:
print(a[x],end=””)
x=x-1
# 11.Find the Reverse of a Number in Python.
a=str(input(“Enter the num:”))
x=-1
for i in a:
print(a[x],end=””)
x=x-1
data = int(input(“enter the num: “))
x = [int(i) for i in str(data)]
x.reverse()
print(x)
number = (input(“Enter a number: “))
###### METHOD – 1
print(str(number[::-1]))
###### METHOD – 2
num = int(input(“Enter a number: “))
rev = 0
while(num > 0):
rev = (rev * 10) + num % 10
num = num//10
print(f”reverse = {rev}”)
num = input(‘Enter the number’)
lst = list(num)
ln = len(num)
if ln ==0:
print(‘No number’)
else:
rev_lst = list(reversed(lst))
print(rev_lst)
rev = ”.join(rev)
print(rev)
num=input()
r=””
for i in num:
r=str(i)+r
print(r)
num = input()
s = ”
for i in range(len(num)-1,-1, -1):
s = s + num[i]
print(s)
is this correct approach?
num=input(“enter the number:”)
i=num[::-1]
print(int(i))
Code is right but you have take input as string.because indexing working only on strings.
def getReverse(num):
res=0
while num:
remainder = num%10
num = num//10
res = res*10+remainder
return res
print(getReverse(int(input())))