Infosys Coding Interview Questions
Infosys Coding Interview Questions 2022
Prepare for Infosys Interview with the latest Infosys Coding Questions. On this page, we have provided Infosys Coding Interview Questions for Freshers.
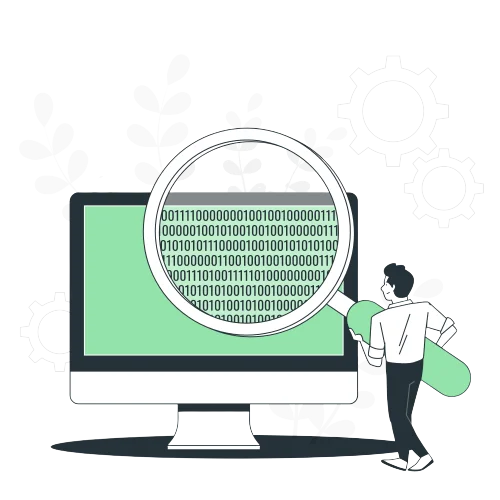
Infosys Coding Questions 2022
Question 1: Write a Program to Swap Two Numbers.
# Swap two variables in Python a=int(input(“Enter value : “)) b=int(input(“Enter value : “)) print(“Before swapping a :”,a) print(“Before swapping b :”,b) #logic to swap using third variable temp=a a=b b=temp print(“After swapping a becomes :”,a) print(“After swapping b becomes :”,b)
Question 2: Write a Program to Swap Two Numbers without using the Third Variable.
a=int(input(“Enter value : “)) b=int(input(“Enter value : “)) print(“Before swapping a :”,a) print(“Before swapping b :”,b) #logic to swap without using third variable a=a+b b=a-b a=a-b print(“After swapping a becomes :”,a) print(“After swapping b becomes :”,b)
Question 3: Write a Program to Convert Decimal Number to Binary Number.
// Decimal to binary conversion using an Array in C #include<stdio.h> void convert(int num) { // creating an array to store binary equivalent int binaryArray[32]; // using i to store binary bit at given array position int i = 0; while (num > 0) { // resultant remainder is stored at given array position binaryArray[i] = num % 2; num = num / 2; i++; } // printing binary array in reverse order for (int j = i - 1; j >= 0; j--) printf("%d",binaryArray[j]); } int main() { int n = 11; convert(n); return 0; }
Find More Solutions at: C Program to Concert Decimal to Binary
#include<iostream> using namespace std; void convertBinary(int num) { // creating an array to store binary equivalent int binaryArray[32]; // using i to store binary bit at given array position int i = 0; while (num > 0) { // resultant remainder is stored at given array position binaryArray[i] = num % 2; num = num / 2; i++; } // printing binary array in reverse order for (int j = i - 1; j >= 0; j--) cout << binaryArray[j]; } int main() { int n = 21; convertBinary(n); return 0; }
Find More Solutions at : C++ Program for Decimal to Binary Conversion
//Java program to convert decimal number to binary number public class Main { public static void main(String args[]) { //Decimal Number int decimal = 12; //integer array for storing binary digits int binary[] = new int[20]; int i = 0; //writing logic for the conversion while(decimal > 0) { int r = decimal % 2; binary[i++] = r; decimal = decimal/2; } //printing result System.out.print("Binary number : "); for(int j = i-1 ; j >= 0 ; j--) System.out.print(binary[j]+""); } }
Find more solutions at: JAVA Program for Decimal to Binary
def convertBinary(num): binaryArray = [] while num>0: binaryArray.append(num%2) num = num//2 for j in binaryArray: print(j, end="") decimal_num = 21 convertBinary(decimal_num)
Find More Solutions at: Python Program for Decimal to Binary Conversion
Question 4: Write a Program to Convert Decimal Number to Octal Number.
// C Program to convert Decimal to Octal using Array #include<stdio.h> void convert(int num) { // creating an array to store octal equivalent int octalArray[32]; // using i to store octal bit at given array position int i = 0; while (num > 0) { // resultant remainder is stored at given array position octalArray[i] = num % 8; num = num / 8; i++; } // printing octal array in reverse order for (int j = i - 1; j >= 0; j--) printf("%d",octalArray[j]); } int main() { int n = 148; convert(n); return 0; }
Find More Solutions at: C Program for Decimal to Octal Conversion
#include<iostream> using namespace std; void convertBinary(int num) { // creating an array to store binary equivalent int binaryArray[32]; // using i to store binary bit at given array position int i = 0; while (num > 0) { // resultant remainder is stored at given array position binaryArray[i] = num % 2; num = num / 2; i++; } // printing binary array in reverse order for (int j = i - 1; j >= 0; j--) cout << binaryArray[j]; } int main() { int n = 21; convertBinary(n); return 0; }
Find More Solutions at : C++ Program for Decimal to Binary Conversion
//Java program to convert decimal number to octal number import java.util.Scanner; public class Main { public static void main(String args[]) { //scanner class object creation Scanner sc = new Scanner(System.in); //Number int decimal = 148; //integer array for storing octal digits int octal[] = new int[20]; int i = 0; //writing logic for the conversion while(decimal > 0) { int r = decimal % 8; octal[i++] = r; decimal = decimal/8; } //printing result System.out.print("Octal number : "); for(int j = i-1 ; j >= 0 ; j--) System.out.print(octal[j]); //closing scanner class(not compulsory, but good practice) sc.close(); } }
Find More Solutions at: JAVA Program for Decimal to Octal Conversion
decimal = 148 octal = [] while decimal > 0: r = decimal % 8 octal.append(r) decimal = decimal // 8 for i in reversed(octal): print(i, end="")
Find More Solutions at Python Program for Decimal to Octal Conversion
Question 5: Write a Program to Convert Decimal Number to Hexadecimal Number.
#include <stdio.h> void convert (int num) { // creating a char array to store hexadecimal equivalent char hexa[100]; // using i to store hexadecimal bit at given array position int i = 0; while (num != 0) { int rem = 0; rem = num % 16; // check if rem < 10 : Digits : 0 - 9 // ascii 0 : 48 if (rem < 10) { hexa[i] = rem + 48; i++; } // else positional values : A - F // rem value will be > 10, adding 55 will result : A - F // ascii A : 65, B : 66 ..... F : 70 else { hexa[i] = rem + 55; i++; } num = num / 16; } // printing hexadecimal array in reverse order printf ("Hexadecimal:"); for (int j = i- 1; j >= 0; j--) printf ("%c" , hexa[j]); } int main () { int decimal = 1457; convert (decimal); return 0; }
Find More Solutions at: C Program for Decimal to Hexadecimal Conversion
#include<iostream> using namespace std; void getHexadecimal(int decimal) { // creating a char array to store hexadecimal equivalent char result[100]; // pos keep index track & is used to place value in result[] array int pos = 0; while (decimal != 0) { int rem = 0; rem = decimal % 16; // Whenever rem < 10 : we will have [0 - 9] as values in place // Note ASCII of 0 is 48 if (rem < 10) { result[pos] = rem + 48; pos++; } // else whenever remainder >= 10 we will have [A - F] // rem value will be > 10, adding 55 will result : A - F // Note: ASCII A -> 65, B -> 66 ......... F -> 70 else { result[pos] = rem + 55; pos++; } decimal = decimal / 16; } // to get result we need to read the array in opposite fashion cout << "Hexadecimal Value: "; for (int j = pos - 1; j >= 0; j--) cout << result[j]; } int main() { int decimal; cout << "Decimal Value: "; cin >> decimal; getHexadecimal(decimal); return 0; }
Find More Solutions at C++ Program for Decimal to Hexadecimal Conversion
public class Main { public static void main (String[]args) { int decimal = 1457; convert (decimal); } static void convert (int num) { // creating a char array to store hexadecimal equivalent char[] hexa= new char[100]; // using i to store hexadecimal bit at given array position int i = 0; while (num != 0) { int rem = 0; rem = num % 16; // check if rem < 10 : Digits : 0 - 9 // ascii 0 : 48 if (rem < 10) { hexa[i] = (char)(rem + 48); i++; } // else positional values : A - F // rem value will be > 10, adding 55 will result : A - F // ascii A : 65, B : 66 ..... F : 70 else { hexa[i] = (char)(rem + 55); i++; } num = num / 16; } // printing hexadecimal array in reverse order System.out.println("Hexadecimal:"); for (int j = i - 1; j >= 0; j--) System.out.print(hexa[j]); } }
Find More Solutions at: JAVA Program for Decimal to Hexadecimal Conversion
def convert(num): hexa = [] while num != 0: rem = num % 16 if rem < 10: hexa.append(chr(rem + 48)) else: hexa.append(chr(rem + 55)) num = num // 16 hexa.reverse() return ''.join(hexa) decimal = 2545 print("Hexadecimal :", convert(decimal))
Find More Solutions at: Python Program to Decimal to Hexadecimal
Question 6: Write a Program to Convert Octal Number to Binary Number.
#include<stdio.h> #include<math.h> void convert(int octal) { int i = 0, decimal = 0; //converting octal to decimal while (octal!=0) { int digit = octal % 10; decimal += digit * pow(8, i); octal /= 10; i++; } printf("Decimal Value: %d\n",decimal); long long binary = 0; int rem; i = 1; // converting decimal to binary here while(decimal!=0) { rem = decimal % 2; decimal /= 2; binary += rem * i; // moving to next position ex: units -> tens i *= 10; } printf("Binary Value: %d",binary); } int main() { int octal; printf("Octal Value: "); scanf("%d", &octal); convert(octal); return 0; }
Find More Solutions at: C Program for Octal to Binary Conversion
#include<iostream> #include<math.h> using namespace std; void convert(int octal) { int i = 0, decimal = 0; //converting octal to decimal while (octal!=0) { int digit = octal % 10; decimal += digit * pow(8, i); octal /= 10; i++; } printf("Decimal Value: %d\n",decimal); long long binary = 0; int rem; i = 1; // converting decimal to binary here while(decimal!=0) { rem = decimal % 2; decimal /= 2; binary += rem * i; // moving to next position ex: units -> tens i *= 10; } cout<<binary; } int main() { int octal; cout<<"Octal Value: "; cin>>octal; convert(octal); return 0; }
Find More Solutions at C++ Program for Octal to Binary Conversion
class Main { public static void main(String args[]) { int octal = 12; //Declaring variable to store decimal number int decimal = 0; //Declaring variable to use in power int n = 0; //writing logic for the octal to decimal conversion while(octal > 0) { int temp = octal % 10; decimal += temp * Math.pow(8, n); octal = octal/10; n++; } int binary[] = new int[20]; int i = 0; //writing logic for the decimal to binary conversion while(decimal > 0) { int r = decimal % 2; binary[i++] = r; decimal = decimal/2; } //printing result System.out.print("Binary number : "); for(int j = i-1 ; j >= 0 ; j--) System.out.print(binary[j]+""); } }
Find More Solutions at JAVA Program for Octal to Binary Conversion
def convert(octal): i = 0 decimal = 0 while octal != 0: digit = octal % 10 decimal += digit * pow(8, i) octal //= 10 i += 1 print("Decimal Value :", decimal) binary = 0 rem = 0 i = 1 while decimal != 0: rem = decimal % 2 decimal //= 2 binary += rem * i i *= 10 print("Binary Value :", binary) octal = int(input("Octal Value : ")) convert(octal)
Find More Solutions at Python Program for Octal to Binary Conversion
Question 7: Write a Program to Convert Octal Number to Decimal Number.
#include<stdio.h> #include<math.h> // function to convert octal to decimal int convert(long long num) { int i = 0, decimal = 0; // will only work for bases 2 - 10 int base = 8; //converting octal to decimal while (num!=0) { int digit = num % 10; decimal += digit * pow(base, i); num /= 10; i++; } return decimal; } //main program int main() { // long used rather than int to store large values long long octal; printf("Enter Octal Number: "); scanf("%lld", &octal); printf("Decimal: %lld", convert(octal)); return 0; }
Find More Solutions at C Program for Octal to Decimal Conversion
#include<iostream> #include<math.h> using namespace std; // function to convert octal to decimal int getOctal(long long num) { int i = 0, decimal = 0; // will only work for bases 2 - 10 int base = 8; //converting octal to decimal while (num!=0) { int digit = num % 10; decimal += digit * pow(base, i); num /= 10; i++; } return decimal; } // main program int main() { // long used rather than int to store large values // Ex : int wont store 111111111111 (12 digits) as // limit for int is 2147483647 (10 digits) long long octal = 462; cout << getOctal(octal); return 0; }
Find More Solutions at C++ Program for Octal to Decimal Conversion
import java.util.Scanner; public class Main { public static void main(String args[]) { //scanner class object creation Scanner sc = new Scanner(System.in); //input from user System.out.print("Enter a octal number : "); int octal = sc.nextInt(); //Declare variable to store decimal number int decimal = 0; //Declare variable to use in power int n = 0; //writing logic for the conversion while(octal > 0) { int temp = octal % 10; decimal += temp * Math.pow(8, n); octal = octal/10; n++; } //printing result System.out.println("Decimal number : "+decimal); //closing scanner class(not compulsory, but good practice) sc.close(); } }
def OctalToDecimal(num): decimal_value = 0 base = 1 while num: last_digit = num % 10 num = int(num / 10) decimal_value += last_digit * base base = base * 8 return decimal_value octal = 512 print("The decimal value of",octal, " is",OctalToDecimal(octal))
Find More Solutions at Python Program for Octal to Decimal Conversion
Question 8: Write a Program to find out the Spiral Traversal of a Matrix.
#include <stdio.h> #define r 4 #define c 4 int main() { int a[4][4] = { { 1, 2, 3, 4 }, { 5, 6, 7, 8 }, { 9, 10, 11, 12 }, { 13, 14, 15, 16 } }; int i, left = 0, right = c-1, top = 0, bottom = r-1; while (left <= right && top <= bottom) { /* Print the first row from the remaining rows */ for (i = left; i <= right; ++i) { printf("%d ", a[top][i]); } top++; /* Print the last column from the remaining columns */ for (i = top; i <= bottom; ++i) { printf("%d ", a[i][right]); } right--; /* Print the last row from the remaining rows */ if (top <= bottom) { for (i = right; i >= left; --i) { printf("%d ", a[bottom][i]); } bottom--; } /* Print the first column from the remaining columns */ if (left <= right) { for (i = bottom; i >= top; --i) { printf("%d ", a[i][left]); } left++; } } return 0; }
Find More Solutions at C Program to Find the Spiral Traversal of a Matrix
#include <bits/stdc++.h> #define r 4 #define c 4 using namespace std; int main() { int a[4][4] = { { 1, 2, 3, 4 }, { 5, 6, 7, 8 }, { 9, 10, 11, 12 }, { 13, 14, 15, 16 } }; int i, left = 0, right = c-1, top = 0, bottom = r-1; while (left <= right && top <= bottom) { /* Print the first row from the remaining rows */ for (i = left; i <= right; ++i) { cout<<a[top][i]<<" "; } top++; /* Print the last column from the remaining columns */ for (i = top; i <= bottom; ++i) { cout<<a[i][right]<<" "; } right--; /* Print the last row from the remaining rows */ if (top <= bottom) { for (i = right; i >= left; --i) { cout<<a[bottom][i]<<" "; } bottom--; } /* Print the first column from the remaining columns */ if (left <= right) { for (i = bottom; i >= top; --i) { cout<<a[i][left]<<" "; } left++; } } return 0; }
Find More Solutions at C++ Program for Spiral Traversal of a Matrix
import java.util.*; class Main{ static int R = 4; static int C = 4; static void print(int arr[][], int i, int j, int m, int n) { if (i >= m || j >= n) { return; } for (int p = i; p < n; p++) { System.out.print(arr[i][p] + " "); } for (int p = i + 1; p < m; p++) { System.out.print(arr[p][n - 1] + " "); } if ((m - 1) != i) { for (int p = n - 2; p >= j; p--) { System.out.print(arr[m - 1][p] + " "); } } if ((n - 1) != j) { for (int p = m - 2; p > i; p--) { System.out.print(arr[p][j] + " "); } } print(arr, i + 1, j + 1, m - 1, n - 1); } public static void main(String[] args) { int a[][] = { { 1, 2, 3, 4 }, { 5, 6, 7, 8 }, { 9, 10, 11, 12 }, { 13, 14, 15, 16 } }; print(a, 0, 0, R, C); } }
Find More Solutions at JAVA Program for Spiral Traversal of a Matrix.
Question 9: Write a Program to Rotate a Matrix by 90 degrees.
#include <stdio.h> void swap(int *x, int *y){ int temp = *x; *x = *y; *y= temp; } int main(){ int n=4; int mat[4][4]= { { 1, 2, 3, 4 },{ 5, 6, 7, 8 },{ 9, 10, 11, 12 },{ 13, 14, 15, 16 } }; //Tranposing the matrix for(int i=0; i<n; i++){ for(int j=i+1; j<n; j++) swap(&mat[i][j], &mat[j][i]); } //Reversing each row of the matrix for(int i=0; i<n; i++){ for(int j=0; j<n/2; j++){ swap(&mat[i][j], &mat[i][n-j-1]); } } //Print the matrix printf("Rotated Matrix :\n"); for(int i=0; i<n; i++){ for(int j=0; j<n; j++){ printf("%d ",mat[i][j]); } printf("\n"); } }
Find More Solutions at C Program to rotate a matrix by 90 degrees.
#include<bits/stdc++.h> using namespace std; int main(){ int n=4; int mat[n][n]= { { 1, 2, 3, 4 },{ 5, 6, 7, 8 },{ 9, 10, 11, 12 },{ 13, 14, 15, 16 } }; //Tranposing the matrix for(int i=0; i<n; i++){ for(int j=i+1; j<n; j++) swap(mat[i][j], mat[j][i]); } //Reversing each row of the matrix for(int i=0; i<n; i++){ for(int j=0; j<n/2; j++){ swap(mat[i][j], mat[i][n-j-1]); } } //Print the matrix cout<<"Rotated Matrix :\n"; for(int i=0; i<n; i++){ for(int j=0; j<n; j++){ cout<<mat[i][j]<<" "; } cout<<endl; } }
Find More Solutions at C++ Program to Rotate a Matrix by 90 degrees.
import java.util.*; class Main { static void reverseRows (int mat[][]) { int n = mat.length; for (int i = 0; i < mat.length; i++){ for (int j = 0; j < mat.length/ 2; j++){ int temp = mat[i][j]; mat[i][j] = mat[i][n - j - 1]; mat[i][n - j - 1] = temp; } } } static void transpose (int arr[][]) { for (int i = 0; i < arr.length; i++) for (int j = i; j < arr[0].length; j++){ int temp = arr[j][i]; arr[j][i] = arr[i][j]; arr[i][j] = temp; } } static void printMatrix (int arr[][]){ for (int i = 0; i < arr.length; i++){ for (int j = 0; j < arr[0].length; j++) System.out.print (arr[i][j] + " "); System.out.println (""); } } static void rotate90 (int arr[][]) { transpose (arr); reverseRows (arr); } public static void main (String[]args) { int arr[][] = { {1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16} }; rotate90 (arr); printMatrix (arr); } }
Find More Solutions at JAVA Program to Rotate a Matrix by 90 degrees.
Question 10: Write a Program to Find a Specific Pair in a Matrix
#include <stdio.h> #include <limits.h> #define N 5 int findMaxValue(int mat[][N]) { int maxValue = INT_MIN; for (int a = 0; a < N - 1; a++) for (int b = 0; b < N - 1; b++) for (int d = a + 1; d < N; d++) for (int e = b + 1; e < N; e++) if (maxValue < (mat[d][e] - mat[a][b])) maxValue = mat[d][e] - mat[a][b]; return maxValue; } int main() { int mat[N][N] = { { 1, 2, -1, -4, -20 }, { -8, -3, 4, 2, 1 }, { 3, 8, 6, 1, 3 }, { -4, -1, 1, 7, -6 }, { 0, -4, 10, -5, 1 } }; printf("Maximum Value is %d", findMaxValue(mat)); return 0; }
Find More Solutions at C Program to Find a Specific Pair in a Matrix
import java.io.*; import java.util.*; class Main { static int findMaxValue(int N,int mat[][]) { int maxValue = Integer.MIN_VALUE; for (int a = 0; a < N - 1; a++) for (int b = 0; b < N - 1; b++) for (int d = a + 1; d < N; d++) for (int e = b + 1; e < N; e++) if (maxValue < (mat[d][e] - mat[a][b])) maxValue = mat[d][e] - mat[a][b]; return maxValue; } public static void main (String[] args) { int N = 5; int mat[][] = { { 1, 2, -1, -4, -20 }, { -8, -3, 4, 2, 1 }, { 3, 8, 6, 1, 3 }, { -4, -1, 1, 7, -6 }, { 0, -4, 10, -5, 1 } }; System.out.print("Maximum Value is " + findMaxValue(N,mat)); } }
Find More Solutions at JAVA Program to Find a Specific Pair in a Matrix
N = 5 def findMaxValue(mat): maxValue = 0 for a in range(N - 1): for b in range(N - 1): for d in range(a + 1, N): for e in range(b + 1, N): if maxValue < (mat[d][e] - mat[a][b]): maxValue = mat[d][e] - mat[a][b] return maxValue matrix = [[ 1, 2, -1, -4, -20], [-8, -3, 4, 2, 1], [ 3, 8, 6, 1, 3], [-4, -1, 1, 7, -6], [ 0, -4, 10, -5, 1]] print("Maximum Value is", findMaxValue(matrix))
Find More Solutions at Python Program to Find a Specific Pair in a Matrix.
More Infosys Coding Questions:-
- Write a program for swapping of two arrays.
- Write a program for swapping of two strings.
- Write a program to convert the string from upper case to lower case.
- Write a program to convert the string from lower case to upper case.
- Write a program to delete all consonants from a given string.
- Write a program to count the different types of characters in given string.
- Write a program to sort the characters of a string.
- Write a program for addition of two matrices.
- Write a program for subtraction of two matrices.
- Write a program for multiplication of two matrices.
- Write a program to find out the sum of diagonal element of a matrix.
These questions are easy .please provide us more top questions for Infosys
thankew so much
Post some difficult level programs…Thank you