Persistent Advanced Coding Questions and Answers
Advanced Coding Questions for Persistent 2024
Persistent Advanced Coding Questions is a new section being added in the Persistent Hiring process for 2024 grads. This section is comprised of 2 advance coding questions which you have to code for in your desired language in the given time limit of 45 mins.
The difficulty level of this section is higher than the rest of the sections, as clearing this section will provide you a raise in your package. So we will suggest you to prepare well for solving Persistent Advanced Coding Questions.
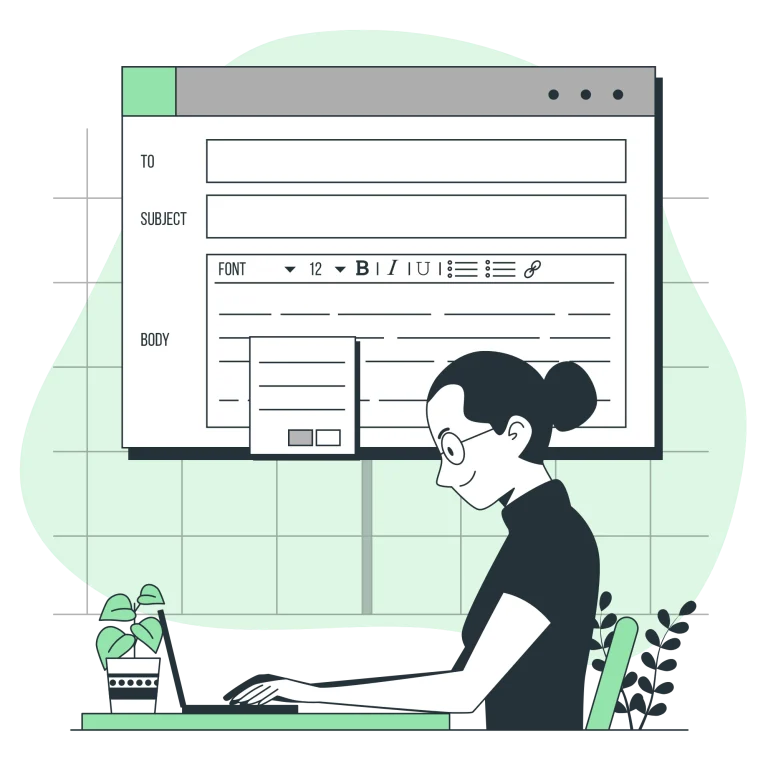
This round is an advanced round, which gives a chance to the students to increase their package. If you successfully clears Persistent Advanced Coding Questions, there is a very high probability that it will result in boosting up your package by 10% – 20%. But in case if you fail to clear this
Persistent Advanced Coding Round Details
Persistent Advanced coding round | Details |
---|---|
No. of questions | 2 |
Time Limit | 45 mins |
Difficulty | High |
Below we have provided some of the Sample Practice Questions for Persistent Advanced Coding Questions Round, make sure you go through all of them while preparing for this round, this will help you in analyzing the difficulty level and pattern of this Advance Coding Section
Lets Practice
Persistent Advanced Coding Question 1
Problem Statement – : Ramu has N dishes of different types arranged in a row: A1,A2,…,AN where Ai denotes the type of the ith dish. He wants to choose as many dishes as possible from the given list but while satisfying two conditions:- He can choose only one type of dish.
- No two chosen dishes should be adjacent to each other.
- Example :
- Given N= 9 and A= [1,2,2,1,2,1,1,1,1]
- For type 1, Ramu can choose at most four dishes. One of the ways to choose four dishes of type 1 is A1,A4, A7 and A9.
- For type 2, Ramu can choose at most two dishes. One way is to choose A3 and A5.
- The first line contains T, the number of test cases. Then the test cases follow.
- For each test case, the first line contains a single integer
- The second line contains N integers A1,A2,…,AN.
- For each test case, print a single line containing one integer ― the type of the dish that Ramu should choose from. If there are multiple answers, print the smallest one.
- 1 <= T <= 10^3
- 1 <= N <= 10^3
- 1 <= Ai <= 10^3
- Sample Input :
- Sample Output :
- EXPLANATION :
- Test case 1:
- For both type 1 and type 2, Ramu can pick at most two dishes. In the case of multiple answers, we pick the smallest one. Hence the answer will be 1
- Test case 2:
- There are only dishes of type 1. So the answer is 1.
- Test case 3:
- For type 1, Ramu can choose at most two dishes. For type 2, he can choose three dishes. For type 3 and type 4, Ramu can choose the only dish available. Hence the maximum is in type 2 and so the answer is 2
- Test case 1:
#include<iostream> using namespace std; int main() { int t,n,i,max,x; cin>>t; while(t--) { cin>>n; int arr[n]; max=0; for(i=0;i<n;i++) { cin>>arr[i]; } int b[1001]={0}; for(i=0;i<n;i++) { b[arr[i]]++; if(arr[i]==arr[i+1]) i++; } for(i=1;i<=1000;i++) { if(b[i]>max) { max=b[i]; x=i; } } cout<<x<<endl; } return 0; }
Output 3 5 1 2 2 1 2 1 6 1 1 1 1 1 1 1 8 1 2 2 2 3 4 2 1 2
Advanced Coding Question 2
Problem Statement-: There are three piles of stones. The first pile contains ‘a’ stones, the second pile contains ‘b’ stones and the third pile contains ‘c’ stones. You must choose one of the piles and split the stones from it to the other two piles; specifically, if the chosen pile initially contained s stones, you should choose an integer k (0≤k≤s), move k stones from the chosen pile onto one of the remaining two piles and s−k stones onto the other remaining pile. Determine if it is possible for the two remaining piles (in any order) to contain x stones and y stones respectively after performing this action.
INPUT FORMAT :
- The first line of the input contains a single integer T denoting the number of test cases. The description of T test cases follows.
- The first and only line of each test case contains five space-separated integers
a,b,c, x and y.
OUTPUT FORMAT :
- For each test case, print a single line containing the string “YES” if it is possible to obtain piles of the given sizes or “NO” if it is impossible.
CONSTRAINTS :
- 1 <= T <= 100
- 1 <= a,b,c,x,y <= 10^9
SAMPLE INPUT :
4
1 2 3 2 4
3 2 5 6 5
2 4 2 6 2
6 5 2 12 1
SAMPLE OUTPUT :
YES
NO
YES
NO
EXPLANATION :
- Test case 1: You can take the two stones on the second pile, put one of them on the first pile and the other one on the third pile.
- Test case 2: You do not have enough stones.
- Test case 3: You can choose the first pile and put all stones from it on the second pile.
#include <bits/stdc++.h> using namespace std; using ll = long long; int main() { int t; cin >> t; while(t--) { int a, b, c, x, y; cin >> a >> b >> c >> x >> y; if((a + b + c ) != (x + y) ) { cout << "NO" << endl; } else { if(y < min(a, min(b, c)) || x < min(a, min(b, c))) { cout << "NO" << endl; } else { cout << "YES" << endl; } } } }
Output 4 1 2 3 2 4 YES 3 2 5 6 5 NO 2 4 2 6 2 YES 6 5 2 12 1 NO
Advanced Coding Question 3
Problem Statement -: Atulya is the judge of a competition. There are two players participating in this competition — Alice and Bob.
The competition consists of N races. For each i (1 ≤ i ≤ N), Alice finished the i-th race in Ai minutes, while Bob finished it in Bi minutes. The player with the smallest sum of finish times wins. If this total time is the same for Alice and for Bob, a draw is declared.
The rules of the competition allow each player to choose a race which will not be counted towards their total time. That is, Alice may choose an index x and her finish time in the race with this index will be considered zero; similarly, Bob may choose an index y and his finish time in the race with this index will be considered zero. Note that x can be different from y; the index chosen by Alice does not affect Bob’s total time or vice versa.
Atulya, as the judge, needs to announce the result of the competition. He knows that both Alice and Bob play optimally and will always choose the best option. Please help Atulya determine the result!
Sample Input :
- The first line of the input contains a single integer T denoting the number of test cases. The description of T test cases follows.
- The first line of each test case contains a single integer N.
- The second line contains N space-separated integers A1, A2, …, AN.
- The third line contains N space-separated integers B1, B2, …, BN.
Sample Output:
- For each test case, print a single line containing the string “Alice” if Alice wins, “Bob” if Bob wins or “Draw” if the result is a draw (without quotes).
Constraints:
- 1 ≤ T ≤ 100
- 2 ≤ N ≤ 100
- 1 ≤ Ai ≤ 1000 for each valid i
- 1 ≤ Bi ≤ 1000 for each valid i
SAMPLE INPUT :
3
5
3 1 3 3 4
1 6 2 5 3
5
1 6 2 5 3
3 1 3 3 4
3
4 1 3
2 2 7
SAMPLE OUTPUT :
Alice
Bob
Draw
EXPLANATION :
- Example case 1: Alice will choose the finish time in the last race to be considered zero, which means her sum of finish times is 3 + 1 + 3 + 3 + 0 = 10, while Bob will choose the finish time of his second race to be considered zero, so his total sum of finish times is 1 + 0 + 2 + 5 + 3 = 11. Since Alice’s sum is smaller, she is considered the winner.
- Example case 2: We’re dealing with the same situation as in the previous case, but finish times for the players are swapped, so Bob wins this time.
- Example case 3: Alice will choose the finish time of the first race to be considered zero, which means her total time is 0 + 1 + 3 = 4. Bob will choose the finish time of his last race to be considered zero, which makes his total time 2 + 2 + 0 = 4. The competition is considered a draw because both players have equal sums of finish times.
#include<bits/stdc++.h> using namespace std; int compare (const void *a, const void *b) { return (*(int *) a - *(int *) b); } int main () { int t; cin >> t; while (t--) { int n; cin >> n; int *a = new int[n]; int *b = new int[n]; for (int i = 0; i < n; i++) cin >> a[i]; for (int i = 0; i < n; i++) cin >> b[i]; qsort (a, n, sizeof (int), compare); qsort (b, n, sizeof (int), compare); int suma = 0, sumb = 0; for (int i = 0; i < n - 1; i++) suma += a[i]; for (int i = 0; i < n - 1; i++) sumb += b[i]; if (suma > sumb) cout << "Bob" << '\n'; else if (suma < sumb) cout << "Alice" << '\n'; else if (suma == sumb) cout << "Draw" << '\n'; } return 0; }
Output Alice Bob Draw
Advanced Coding Question 4
Problem Statement-: Altaf has recently learned about number bases and is becoming fascinated.
Altaf learned that for bases greater than ten, new digit symbols need to be introduced, and that the convention is to use the first few letters of the English alphabet. For example, in base 16, the digits are 0123456789ABCDEF. Altaf thought that this is unsustainable; the English alphabet only has 26 letters, so this scheme can only work up to base 36. But this is no problem for Altaf, because Altaf is very creative and can just invent new digit symbols when she needs them. (Altaf is very creative.)
Altaf also noticed that in base two, all positive integers start with the digit 1! However, this is the only base where this is true. So naturally, Altaf wonders: Given some integer N, how many bases b are there such that the base-b representation of N starts with a 1?
- Input Format :
- The first line of the input contains an integer T denoting the number of test cases. The description of T test cases follows.
- Each test case consists of one line containing a single integer N (in base ten).
- Output Format :
- For each test case, output a single line containing the number of bases b, or INFINITY if there are an infinite number of them.
- Constraints:
- 1 <= T <= 10^5
- 0 <= N < 10^12
Sample Input
4
6
9
11
24
Sample Output:
4
7
8
14
Explanation:
In the first test case, 6 has a leading digit 1 in bases 2, 4, 5 and 6: 610 = 1102 = 124 = 115 = 106.
#include<bits/stdc++.h> using namespace std; vector < long long int >v[47]; int main () { long long int i, j; for (i = 2; i <= (int) 1e6; i++) { long long int tem = i; for (j = 2; j <= 40; j++) { tem = tem * i; if (tem > (long long int) 1e12) break; v[j].push_back (tem); } } int t; scanf ("%d", &t); while (t--) { long long int m; scanf ("%lld", &m); if (m == 1) { printf ("INFINITY\n"); continue; } long long int ans = (m + 1) / 2; long long int p = m / 2 + 1; for (i = 2; i <= 40; i++) ans = ans + (lower_bound (v[i].begin (), v[i].end (), m + 1) - lower_bound (v[i].begin (), v[i].end (), p)); printf ("%lld\n", ans); } return 0; }
Output 4 6 4 9 7 11 8 24 14
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Frequently Asked Questions (FAQs)
What is the difficulty level of this coding round?
The difficulty level of this section is higher than the rest of the sections, as clearing this section will provide you a raise in your package.
Is advanced coding round a new section?
Persistent Advanced Coding Questions is a new section being added in the Persistent Hiring process for 2024 grads
import math
# Function to precompute the number of mentors for each manager within the range [1, sqrt(r)]
def precompute_mentor_counts(r):
mentor_counts = [0] * (int(math.sqrt(r)) + 1)
for x in range(1, int(math.sqrt(r)) + 1):
for n in range(x**2, (x+1)**2):
if n % x == 0:
mentor_counts[x] += 1
return mentor_counts
# Function to count the number of employees who will not be removed from the company for a given query
def count_employees_within_range(mentor_counts, l, r):
count = 0
for num in range(l, r):
if num <= int(math.sqrt(r)):
count += mentor_counts[num]
return count
# Input
Q = int(input())
queries = []
for _ in range(Q):
i, r = map(int, input().split())
queries.append((i, r))
# Precompute mentor counts for each query
mentor_counts = precompute_mentor_counts(10**18)
# Output
for query in queries:
i, r = query
print(count_employees_within_range(mentor_counts, i, r))