Constructor & Destructor in Python
Constructor & Destructor in Python:
Constructor & Destructor are an important concept of oops in Python.
Constructor: A constructor in Python is a special type of method which is used to initialize the instance members of the class. The task of constructors is to initialize and assign values to the data members of the class when an object of the class is created.
Destructor: Destructor in Python is called when an object gets destroyed. In Python, destructors are not needed, because Python has a garbage collector that handles memory management automatically.
Let’s Understand Constructor & Destructor in Python.
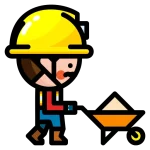
Constructor:
- The __init__ method is similar to constructors in c++ and Java.
- Constructors are used to initialize the object’s state.
- The task of constructors is to initialize(assign values) to the data members of the class when an object of class is created.
Synatx:
class ClassName:
def __init__( self , variables...):
##body
Types of Constructor:
- default constructor: The default constructor is a simple constructor which doesn’t have any argument to pass. Its definition has only one argument which is a reference to the instance being constructed.
- parameterized constructor: constructor which has parameters to pass is known as parameterized constructor. The parameterized constructor takes its first argument as a reference to the instance being constructed known as self.
Code #1:
Output:
PrepInsta
In constructor
Destructor:
- The __del__ method is similar to destructor in c++ and Java.
- Destructors are used to destroying the object’s state.
Syntax:
class ClassName:
def __del__( self ,):
##body
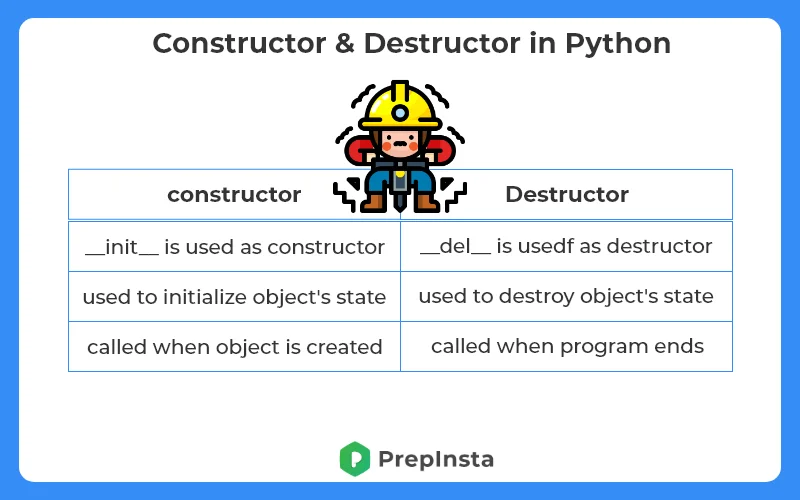
Code #2:
Output:
Object Created PrepInsta
DEstructor is called Manually
- The destructor was called after the program ended.
- It can be called Automatically as well as manually.
- Object will be deleted at the end of the program.
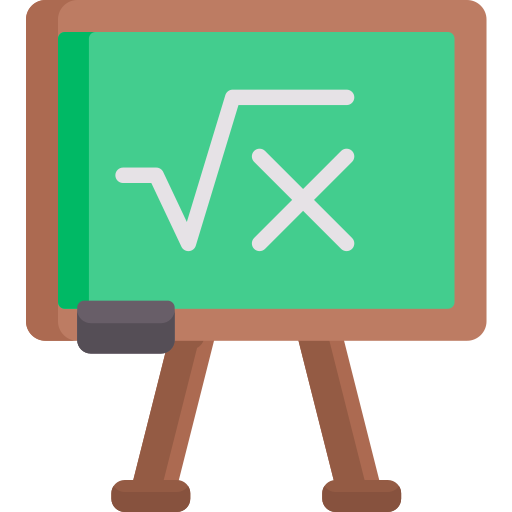
Question 1. Define the use of Destructor Program in Python
(Amazon – Interview)
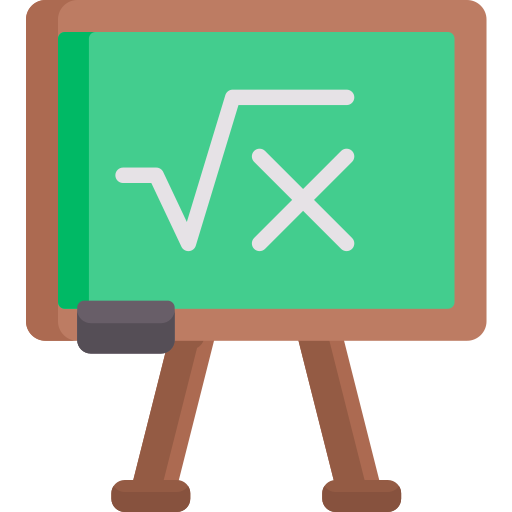
Question 2. The worst-case occurred in the linear search algorithm when
- The element in the middle of an array
- Item present in the last
- Item present in the starting
- Item has the maximum value
(TCS NQT)
If the element situated at the end of the array, so it takes maximum time to search for that of the element.
Ans. Option B
Login/Signup to comment