LTI Coding Questions and Solutions 2025
LTI Coding Questions for 2025 graduates
LTI Coding Questions 2025 with solutions are given here on this page. This section is the most important part of the LTI Recruitment Process 2025.
These type of Coding Questions will help you in your coding test as well as during your interview process.
- Written Assessment – 2 coding question : 40 mins
- Level 1 – 1 coding question : 60 mins (Shared)
- Level 2- 1 coding question : 60 mins (shared)
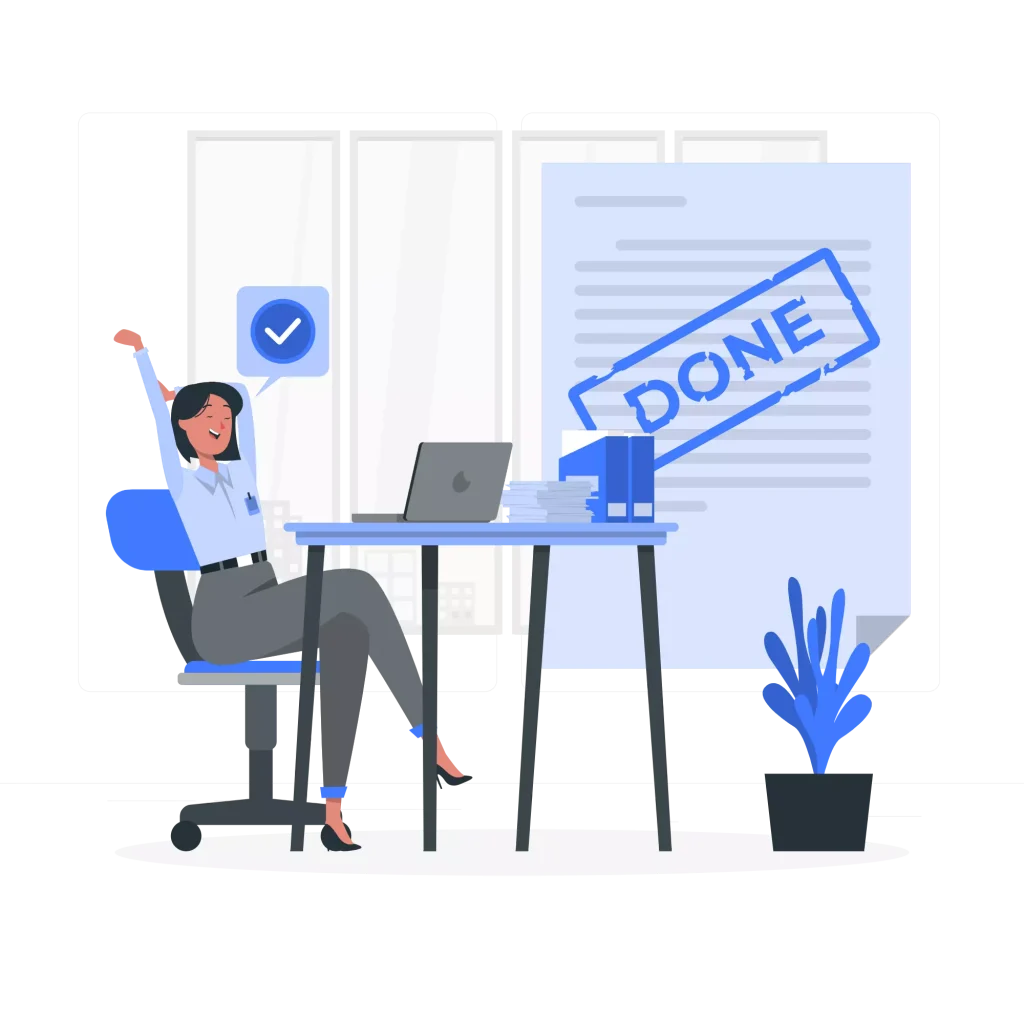
L&T Infotech Coding Questions Details
The LTI hiring process consists of multiple rounds, you need to clear each round to be eligible for the next round. The rounds have different coding questions, whose level of difficulty goes on increasing as you proceed to the next round every time.
Here below we have given some sample practice questions for LTI coding round, based on the pattern of previous year coding questions, make sure you practice all of these in order to prepare well for the LTI Coding Questions round.
Levels | No. of questions | Total Time |
---|---|---|
Automata Fix | 2 Coding Questions | 40 mins |
Level 1 | Coding- 1 Ques | 60 mins (shared) |
Level 2 | Coding- 1 Ques | 60 mins (shared) |
Question 1
Johnny was absent in his english class when the vowel topic was taught by the teacher . His english teacher gave him two strings and told him to find the length of the longest common subsequence which contains only vowels, as a punishment for not attending english class yesterday .
Help Jhonny by writing a code to find the length of the longest common subsequence
Input Specification:
- input1: First string given by his teacher
- input2: Second string given by his teacher.
Output Specification:
- Return the length of the longest common subsequence which contains only vowels.
Example 1:
vowelpunish
english
Output : 2
Example 2:
prepinsta
prepare
Output : 2
#include <bits/stdc++.h>
using namespace std;
bool isVowel (char ch)
{
if (ch == 'a' || ch == 'e' || ch == 'i' ||ch == 'o' || ch == 'u')
return true;
return false;
}
int lcs (char *X, char *Y, int m, int n)
{
int L[m + 1][n + 1];
int i, j;
L[i][j] contains length of LCS of X[0..i - 1] and Y[0..j - 1]
for (i = 0; i <= m; i++)
{
for (j = 0; j <= n; j++)
{
if (i == 0 || j == 0)
L[i][j] = 0;
else if ((X[i - 1] == Y[j - 1]) && isVowel (X[i - 1]))
L[i][j] = L[i - 1][j - 1] + 1;
else
L[i][j] = max (L[i - 1][j], L[i][j - 1]);
}
}
return L[m][n];
}
int main ()
{
char X[];
char Y[];
cin >> X;
cin >> Y;
int m = strlen (X);
int n = strlen (Y);
cout << lcs (X, Y, m, n);
return 0;
}
import java.util.*;
public class Main
{
static boolean Vowel(char ch)
{
if (ch == 'a' || ch == 'e' ||ch == 'i' || ch == 'o' ||ch == 'u')
return true;
return false;
}
static int Lcs(String X, String Y, int m, int n)
{
int L[][] = new int[m + 1][n + 1];
int i, j;
for (i = 0; i <= m; i++)
{
for (j = 0; j <= n; j++)
{
if (i == 0 || j == 0)
L[i][j] = 0;
else if ((X.charAt(i - 1) == Y.charAt(j - 1)) &&Vowel(X.charAt(i - 1)))
L[i][j] = L[i - 1][j - 1] + 1;
else
L[i][j] = Math.max(L[i - 1][j],L[i][j - 1]);
}
}
return L[m][n];
}
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
String a = sc.next();
String b = sc.next();
int i = a.length();
int j = b.length();
System.out.println(""+Lcs(a, b, i, j));
}
}
a=input()
b= input()
m,n=len(a),len(b)
vow="aeiou"
DP= [[0 for i in range(n + 1)]for j in range(m + 1)]
i, j = 0, 0
for i in range(m + 1):
for j in range(n + 1):
if (i == 0 or j == 0):
DP[i][j] = 0
elif ((a[i - 1] == b[j - 1]) and a[i - 1] in vow):
DP[i][j] = DP[i - 1][j - 1] + 1
else:
DP[i][j] = max(DP[i - 1][j],DP[i][j - 1])
print(DP[m][n])
Question 2
Naman on his way home found ‘N’ tokens on the ground arranged in a line horizontally. Each token has some number written on it.
Naman wants to count the longest sub-sequence of the tokens with a decreasing arrangement.
A sub-sequence is a sequence that can be derived from another sequence by deleting some or no elements without changing the order of the remaining elements keeping the relative positions of elements same as it was in the initial sequence. A decreasing sub-sequence is a sub-sequence in which every element is strictly less than the previous number.
Your task is to help Naman in finding the longest subsequence of decreasing arrangement of tokens.
Input Specification:
- input1: integer ‘n’ denoting size of array
- input2: integer array ‘A’ containing ‘n’ elements
Output Specification:
For each test case, print the integer denoting the length of the longest decreasing subsequence.
Print the output of each test case in a separate line.
Constraints:
- 1 <= n <= 5000
- 1 <= A[i] <= 10^9
- Time Limit: 1 sec
Example1:
Input1 : 5
Input2 : {5,0,3,2,9}
Output: 3
Explanation: The longest decreasing subsequence is of length 3, i.e. [5, 3, 2]
Example2:
input1: 9
input2: {15, 27, 14, 38, 63, 55, 46, 65, 85}
Output: 3
Explanation: The longest decreasing subsequence is of length 3, i.e. [63, 55, 46]
#include <bits/stdc++.h>
using namespace std;
int lds(int arr[], int n)
{
int lds[n];
int i, j, max = 0;
for (i = 0; i < n; i++)
lds[i] = 1;
for (i = 1; i < n; i++)
for (j = 0; j < i; j++)
if (arr[i] < arr[j] && lds[i] < lds[j] + 1)
lds[i] = lds[j] + 1;
for (i = 0; i < n; i++)
if (max < lds[i])
max = lds[i];
return max;
}
int main()
{ int n;
cin>>n;
int arr[n];
for(int i=0 ; i<n ; i++)
cin>>arr[i];
cout << lds(arr, n);
return 0;
}
import java.util.*;
import java.io.*;
public class Main
{
static int lds(int arr[], int n)
{
int lds[] = new int[n];
int i, j, max = 0;
for (i = 0; i < n; i++)
lds[i] = 1;
for (i = 1; i < n; i++)
for (j = 0; j < i; j++)
if (arr[i] < arr[j] && lds[i] < lds[j] + 1)
lds[i] = lds[j] + 1;
for (i = 0; i < n; i++)
if (max < lds[i])
max = lds[i];
return max;
}
public static void main (String[] args)
{
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int arr[] = new int[n];
for(int i=0 ; i<n ; i++){
arr[i] = sc.nextInt();
}
System.out.print("" +lds(arr, n));
}
}
n=int(input())
arr = list(map(int,input().split()))
tokens = [1]*n
for i in range(1,n):
for j in range(i):
if (arr[i] < arr[j] and tokens[i] < tokens[j] + 1):
tokens[i] = tokens[j] + 1
print(max(tokens))
Question 3
Maxwell filled his university exam form in which he has filled his mid sem marks but by mistake he has filled DS marks in AI field and AI marks in DS field. He wants to update his mid sem marks but for that he has to pay some penalty.
Penalty equal to the sum of absolute differences between adjacent subject marks.
Return the minimum penalty that must be paid by him.
Input Specification:
- input1: length of an integer array of numbers (2 <= input 1 <= 1000)
- input2: integer array(1 <= input2[i] <= 10000)
Example 1:
Input : arr = {4, 1, 5}
Output : 5
Explanation: Sum of absolute differences is |4-5| + |1-4| + |5-4|
Example 2:
Input : arr = {5, 10, 1, 4, 8, 7}
Output : 9
Example 3:
Input : {12, 10, 15, 22, 21, 20, 1, 8, 9}
Output : 18
#include <bits/stdc++.h>
using namespace std;
int sumOfMinAbsDifferences(int arr[], int n)
{
sort(arr, arr+n);
int sum = 0;
sum += abs(arr[0] - arr[1]);
sum += abs(arr[n-1] - arr[n-2]);
for (int i=1; i<n-1; i++)
sum += min(abs(arr[i] - arr[i-1]), abs(arr[i] - arr[i+1]));
// required sum
return sum;
}
// Driver program to test above
int main()
{
int n;
cin>>n;
int arr[n];
for(int i=0 ; i<n ; i++)
cin>>arr[i];
cout << "Sum = "<< sumOfMinAbsDifferences(arr, n);
}
import java.util.*;
public class Main {
static int Penalty(int arr[] ,int n)
{
Arrays.sort(arr);
int sum = 0;
sum += Math.abs(arr[0] - arr[1]);
sum += Math.abs(arr[n-1] - arr[n-2]);
for (int i = 1; i < n - 1; i++)
sum +=
Math.min(Math.abs(arr[i] - arr[i-1]),
Math.abs(arr[i] - arr[i+1]));
return sum;
}
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int arr[] = new int[n];
for(int i=0 ; i<n ; i++)
arr[i] = sc.nextInt();
System.out.println(""+ Penalty(arr, n));
}
}
n=int(input())
arr = sorted(list(map(int,input().split())))
s=abs(arr[0]-arr[1])+abs(arr[-1]-arr[-2])
for i in range(1,n-1):
s+=min(abs(arr[i]-arr[i-1]),abs(arr[i]-arr[i+1]))
print(s)
Question 4
Given an array arr[] of integers, find out the maximum difference between any two elements such that the larger element appears after the smaller number.
Examples :
Input : arr = {2, 3, 10, 6, 4, 8, 1}
Output : 8
Explanation : The maximum difference is between 10 and 2.
Input : arr = {7, 9, 5, 6, 3, 2}
Output : 2
Explanation : The maximum difference is between 9 and 7.
#include <bits/stdc++.h>
using namespace std;
int maxDiff(int arr[], int arr_size)
{
int max_diff = arr[1] - arr[0];
for (int i = 0; i < arr_size; i++)
{
for (int j = i+1; j < arr_size; j++)
{
if (arr[j] - arr[i] > max_diff)
max_diff = arr[j] - arr[i];
}
}
return max_diff;
}
int main()
{
int arr[] = {1, 2, 90, 10, 110};
int n = sizeof(arr) / sizeof(arr[0]);
// Function calling
cout << "Maximum difference is " << maxDiff(arr, n);
return 0;
}
public class MaximumDiffrence
{
int maxDiff(int arr[], int arr_size)
{
int max_diff = arr[1] - arr[0];
int i, j;
for (i = 0; i < arr_size; i++)
{
for (j = i + 1; j < arr_size; j++)
{
if (arr[j] - arr[i] > max_diff)
max_diff = arr[j] - arr[i];
}
}
return max_diff;
}
public static void main(String[] args)
{
MaximumDiffrence maxdif = new MaximumDiffrence();
int arr[] = {1, 2, 90, 10, 110};
System.out.println("Maximum differnce is " +maxdif.maxDiff(arr, 5));
}
}
n=int(input())
arr=list(map(int,input().split()))
m=-9999999
for i in range(n-1):
for j in range(i+1,n):
m=max(m,arr[j]-arr[i])
print(m)
Question 5
Given an array of integers (both odd and even), the task is to arrange them in such a way that odd and even values come in alternate fashion in non-decreasing order(ascending) respectively.
- If the smallest value is Even then we have to print Even-Odd pattern.
- If the smallest value is Odd then we have to print Odd-Even pattern.
Note: No. of odd elements must be equal to No. of even elements in the input array.
Examples:
Input: arr[] = {1, 3, 2, 5, 4, 7, 10}
Output: 1, 2, 3, 4, 5, 10, 7
Smallest value is 1(Odd) so output will be Odd-Even pattern.
Input: arr[] = {9, 8, 13, 2, 19, 14}
Output: 2, 9, 8, 13, 14, 19
Smallest value is 2(Even) so output will be Even-Odd pattern.
#include <bits/stdc++.h>
using namespace std;
void AlternateRearrange(int arr[], int n)
{
// Sort the array
sort(arr, arr + n);
vector<int> v1; // to insert even values
vector<int> v2; // to insert odd values
for (int i = 0; i < n; i++)
if (arr[i] % 2 == 0)
v1.push_back(arr[i]);
else
v2.push_back(arr[i]);
int index = 0, i = 0, j = 0;
bool flag = false;
// Set flag to true if first element is even
if (arr[0] % 2 == 0)
flag = true;
// Start rearranging array
while (index < n) {
// If first element is even
if (flag == true) {
arr[index++] = v1[i++];
flag = !flag;
}
// Else, first element is Odd
else {
arr[index++] = v2[j++];
flag = !flag;
}
}
// Print the rearranged array
for (i = 0; i < n; i++)
cout << arr[i] << " ";
}
// Driver code
int main()
{
int arr[] = { 9, 8, 13, 2, 19, 14 };
int n = sizeof(arr) / sizeof(int);
AlternateRearrange(arr, n);
return 0;
}
import java.util.* ;
class Main
{
static void AlternateRearrange(int arr[], int n)
{
// Sort the array
// Collection.sort() sorts the
// collection in ascending order
Arrays.sort(arr) ;
Vector v1 = new Vector(); // to insert even values
Vector v2 = new Vector(); // to insert odd values
for (int i = 0; i < n; i++)
if (arr[i] % 2 == 0)
v1.add(arr[i]);
else
v2.add(arr[i]);
int index = 0, i = 0, j = 0;
boolean flag = false;
// Set flag to true if first element is even
if (arr[0] % 2 == 0)
flag = true;
// Start rearranging array
while (index < n)
{
// If first element is even
if (flag == true)
{
arr[index] = (int)v1.get(i);
i += 1 ;
index += 1 ;
flag = !flag;
}
// Else, first element is Odd
else
{
arr[index] = (int)v2.get(j) ;
j += 1 ;
index += 1 ;
flag = !flag;
}
}
// Print the rearranged array
for (i = 0; i < n; i++)
System.out.print(arr[i] + " ");
}
// Driver code
public static void main(String []args)
{
int arr[] = { 9, 8, 13, 2, 19, 14 };
int n = arr.length ;
AlternateRearrange(arr, n);
}
}
Question 6
Given a string of consecutive digits and a number Y, the task is to find the number of minimum sets such that every set follows the below rule:
Set should contain consecutive numbers
No digit can be used more than once.
The number in the set should not be more than Y.
Examples:
Input: s = “1234”, Y = 30
Output: 3
Three sets of {12, 3, 4}
Input: s = “1234”, Y = 4
Output: 4
Four sets of {1}, {2}, {3}, {4}
#include <bits/stdc++.h>
using namespace std;
// Function to find the minimum number of shets
int minimumSets(string s, int y)
{
// Variable to count
// the number of sets
int cnt = 0;
int num = 0;
int l = s.length();
int f = 0;
// Iterate in the string
for (int i = 0; i < l; i++) {
// Add the number to string
num = num * 10 + (s[i] - '0');
// Mark that we got a number
if (num <= y)
f = 1;
else // Every time it exceeds
{
// Check if previous was
// anytime less than Y
if (f)
cnt += 1;
// Current number
num = s[i] - '0';
f = 0;
// Check for current number
if (num <= y)
f = 1;
else
num = 0;
}
}
// Check for last added number
if (f)
cnt += 1;
return cnt;
}
// Driver Code
int main()
{
string s = "1234";
int y = 30;
cout << minimumSets(s, y);
return 0;
}
import java.util.*;
class solution
{
// Function to find the minimum number of shets
static int minimumSets(String s, int y)
{
// Variable to count
// the number of sets
int cnt = 0;
int num = 0;
int l = s.length();
boolean f = false;
// Iterate in the string
for (int i = 0; i < l; i++) {
// Add the number to string
num = num * 10 + (s.charAt(i) - '0');
// Mark that we got a number
if (num <= y)
f = true;
else // Every time it exceeds
{
// Check if previous was
// anytime less than Y
if (f)
cnt += 1;
// Current number
num = s.charAt(i) - '0';
f = false;
// Check for current number
if (num <= y)
f = true;
else
num = 0;
}
}
// Check for last added number
if (f == true)
cnt += 1;
return cnt;
}
// Driver Code
public static void main(String args[])
{
String s = "1234";
int y = 30;
System.out.println(minimumSets(s, y));
}
}
Question 7
Alice is a kindergarten teacher. She wants to give some candies to the children in her class. All the children sit in a line and each of them has a rating score according to his or her performance in the class. Alice wants to give at least 1 candy to each child. If two children sit next to each other, then the one with the higher rating must get more candies. Alice wants to minimize the total number of candies she must buy.
Example
She gives the students candy in the following minimal amounts: . She must buy a minimum of 10 candies.
Function Description
Complete the candies function in the editor below.
candies has the following parameter(s):
- int n: the number of children in the class
- int arr[n]: the ratings of each student
Returns
- int: the minimum number of candies Alice must buy
Input Format
The first line contains an integer, , the size of .
Each of the next lines contains an integer indicating the rating of the student at position .
Sample Input 0
3
1
2
2
Sample Output 0
4
Explanation 0
Here 1, 2, 2 is the rating. Note that when two children have equal rating, they are allowed to have a different number of candies. Hence optimal distribution will be 1, 2, 1.
Sample Input 1
10
2
4
2
6
1
7
8
9
2
1
Sample Output 1
19
Sample Input 2
8
2
4
3
5
2
6
4
5
Sample Output 2
12
import java.util.Arrays;
import java.util.Scanner;
public class Solution {
private static int countMinCandies(int[] ratings) {
int[] candies = new int[ratings.length];
Arrays.fill(candies, 1);
for (int i = 0; i < candies.length;) {
if (i > 0 && ratings[i] > ratings[i-1] && candies[i] <= candies[i-1]) {
candies[i] = candies[i-1] + 1;
i--;
}
else
if (i < candies.length - 1 && ratings[i] > ratings[i+1] && candies[i] <= candies[i+1]) {
candies[i] = candies[i+1] + 1;
if (i > 0) i--;
}
else i++;
}
int totalCandies = 0;
for (int c: candies) {
totalCandies += c;
}
return totalCandies;
}
private static int[] readRatings() {
try {
Scanner scanner = new Scanner(System.in);
int ratingsExpected = scanner.nextInt();
if (ratingsExpected <= 0) throw new RuntimeException("Input: first line is a negative number");
int[] ratings = new int[ratingsExpected];
int i = 0;
while (i < ratingsExpected) {
int rating = scanner.nextInt();
ratings[i] = rating;
i++;
}
return ratings;
} catch (NumberFormatException e) {
throw new RuntimeException("Input corrupt: " + e.getMessage());
}
}
public static void main(String[] args) {
int[] ratings = readRatings();
int minCandies = countMinCandies(ratings);
System.out.println(minCandies);
}
}
#include <bits/stdc++.h>
using namespace std;
int rate [100000 + 10];
long long values [100000 + 10]; // use long long
int main()
{
int n;
long long ans = 0, tmp = 0;
cin >> n;
for(int i = 0; i < n; i++) cin >> rate[i];
values[0] = 1;
// case 1
for(int i = 1; i < n; i++){ // scan input array from left to right
if(rate[i] > rate[i - 1]){
values[i] = values[i - 1] + 1; // case 1, a
} else values[i] = 1; // case 1,b
}
ans = values[n - 1]; // case 2
for(int i = n - 2; i >= 0; i--){ // scan input array from right to left
if(rate[i] > rate[i + 1]){
tmp = values[i + 1] + 1; // case 2, a
} else tmp = 1; // case 2, b
ans += max(tmp, values[i]); // maximum of the two values for child (i)
values[i] = tmp;
}
cout << ans;
return 0;
}
Question 8
Given a string s, the task is to find out the minimum number of adjacent swaps required to make a string is palindrome. If it is not possible, then return -1.
Examples:
Input: aabcb
Output: 3
Explanation:
After 1st swap: abacb
After 2nd swap: abcab
After 3rd swap: abcba
Input: adbcdbad
Output: -1
#include <bits/stdc++.h>
using namespace std;
// Function to Count minimum swap
int countSwap(string s)
{
// calculate length of string as n
int n = s.length();
// counter to count minimum swap
int count = 0;
// A loop which run till mid of
// string
for (int i = 0; i < n / 2; i++) {
// Left pointer
int left = i;
// Right pointer
int right = n - left - 1;
// A loop which run from right
// pointer towards left pointer
while (left < right) {
// if both char same then
// break the loop.
// If not, then we have to
// move right pointer to one
// position left
if (s[left] == s[right]) {
break;
}
else {
right--;
}
}
// If both pointers are at same
// position, it denotes that we
// don't have sufficient characters
// to make palindrome string
if (left == right) {
return -1;
}
// else swap and increase the count
for (int j = right; j < n - left - 1; j++) {
swap(s[j], s[j + 1]);
count++;
}
}
return count;
}
// Driver code
int main()
{
string s;
cin>>s;
// Function calling
int ans1 = countSwap(s);
reverse(s.begin(), s.end());
int ans2 = countSwap(s);
cout << max(ans1, ans2);
return 0;
}
import java.util.*;
public class Main {
// Function to Count minimum swap
static int countSwap(String str)
{
// Legth of string
int n = str.length();
// it will convert string to
// char array
char s[] = str.toCharArray();
// Counter to count minimum
// swap
int count = 0;
// A loop which run in half
// string from starting
for (int i = 0; i < n / 2; i++) {
// Left pointer
int left = i;
// Right pointer
int right = n - left - 1;
// A loop which run from
// right pointer to left
// pointer
while (left < right) {
// if both char same
// then break the loop
// if not same then we
// have to move right
// pointer to one step
// left
if (s[left] == s[right]) {
break;
}
else {
right--;
}
}
// it denotes both pointer at
// same position and we don't
// have sufficient char to make
// palindrome string
if (left == right) {
return -1;
}
else if (s[left] != s[n - left - 1]) {
char temp = s[right];
s[right] = s[n - left - 1];
s[n - left - 1] = temp;
count++;
}
}
return count;
}
// Driver Code
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in)
String s = sc.next();
// Function calling
int ans1 = countSwap(s);
System.out.println(ans1);
}
}
Question 9
Ronny is given a string along with the string which contains single character x. She has to remove the character x from the given string. Help her write a function to remove all occurrences of x character from the given string.
Input Specification:
- input1: input string s
- input2: String containing any character x
Output Specification:
String without the occurrence of character x
Example 1:
Input1: Prepinsta
input2: i
Output: prepnst
Explanation: As i is the character which is required to be removed, therefore all the occurrences of i are removed, keeping all other characters.
#include<stdio.h>
#include<string.h>
int isPalindrome(char str[])
{
int l = 0;
int h = strlen(str) - 1;
while (h > l)
{
if (str[l++] != str[h--])
{
return 0;
}
}
return 1;
}
int main()
{
char str[50];
scanf("%s",str);
printf("%d", isPalindrome(str));
return 0;
}
import java.util.*;
class Solution
{
public static void main(String[] args)
{
Scanner sc=new Scanner(System.in);
String str1=sc.nextLine();
String str2=sc.next();
str1=str1.replaceAll(str2,"");
System.out.println(str1);
}
}
Question 10
Encryption is needed to be done in important chats between army officers so that no one else can read it .
Encryption of message is done by replacing each letter with the letter at 3
positions to the left
e.g. ‘a’ is replaced with ‘x. ‘b’ with ‘y’ … ‘d’ with ‘a’ and so on.
Given a encrypted input string find the corresponding plaintext and return the plaintext as output string.
Note:- All the characters are in the lower case for input and output strings
Input Specification
input1: the ciphertext
Output Specification
Return the corresponding plaintext.
Example1:
input 1: ABCDEFGHIJKLMNOPQRSTUVWXYZ
output: XYZABCDEFGHIJKLMNOPQRSTUVW
Example2:
Input 1: ATTACKATONCE
output: EXXEGOEXSRGI
#include <iostream>
using namespace std;
string encrypt(string text, int s)
{
string result = "";
// traverse text
for (int i=0;i<text.length();i++)
{
if (isupper(text[i]))
result += char(int(text[i]+s-65)%26 +65);
else
result += char(int(text[i]+s-97)%26 +97);
}
return result;
}
int main()
{
string text;
cin>>text;
int s = 3;
cout << encrypt(text, s);
return 0;
}
import java.util.*;
public class CaesarCipher
{
public static StringBuffer encrypt(String text, int s)
{
StringBuffer result= new StringBuffer();
for (int i=0; i<text.length(); i++)
{
if (Character.isUpperCase(text.charAt(i)))
{
char ch = (char)(((int)text.charAt(i) +s - 65) % 26 + 65);
result.append(ch);
}
else
{
char ch = (char)(((int)text.charAt(i) +s - 97) % 26 + 97);
result.append(ch);
}
}
return result;
}
public static void main(String[] args)
{ Scanner sc = new Scanner(System.in)
String text = sc.next();
int s = 3;
System.out.println("" + encrypt(text, s));
}
}
A company manufactures different types of software products. They deliver their products to their N clients. Whenever the company fulfills the complete order of a client. the orderID generated is the concatenation of the number of products delivered for every committed product type. The head of the sales team wishes to find the clientwise data for the total number of products of any type delivered to every client.
63
66
67
68
69
78
int main() 71 (
72
73
74
75
Write an algorithm for the head of sales team to calculate the total number of products of any type delivered to the respective clients.
76 77
78
Input
79
80
The first line of the input consists of an
81
82
integer numOfClients, representing the number of clients (N). The second line consists of N space separated integers orderiDo
83
84
array s
input
scanf(
order
for (
array single
array sing
return an
arra
for
1
pr
orderID, orderiD
representing the orderIDs of the orders delivered to the clients.
90
Output
92
93
Print N space-seperated integers representing the clientwise data for the total number of products of any type delivered to each of the respective clients.