Java Program to Find the Largest Among Three Numbers
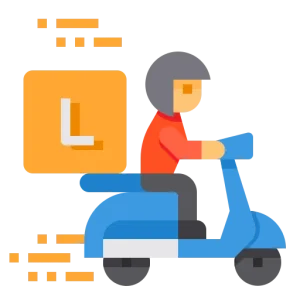
What is Number ?
An item in mathematics used for measurement, counting, and labelling is a number. Many categories can be used to categorise numbers, including natural, whole, integer, rational, irrational, real, and complex numbers. They carry out computations and offer solutions in a number of mathematical operations, including addition, subtraction, multiplication, and division.
Steps to Find the Largest Among Three Numbers :
- Put the three numbers in variables after taking them as input.
- In a new variable, let’s call it “temp,” compare the first and second numbers and store the greater one there.
- If the third number is greater than the “temp” variable, compare the two numbers and put the higher value in the “temp” variable.
- The “temp” variable now has the biggest number of the three. As output, print the value of the variable “temp.”
Pseudo Code for the above algorithm :
Input: three numbers a, b, c temp = a if b > temp: temp = b if c > temp: temp = c Output: temp
Example 1 :
Run
import java.util.Scanner; public class Main { public static void main(String[] args) { Scanner input = new Scanner(System.in); int a, b, c, largest; System.out.print("Enter the first number: "); a = input.nextInt(); System.out.print("Enter the second number: "); b = input.nextInt(); System.out.print("Enter the third number: "); c = input.nextInt(); largest = a; if (b > largest) { largest = b; } if (c > largest) { largest = c; } System.out.println("The largest number is " + largest); } }
Output :
Enter the first number: 12 Enter the second number: 17 Enter the third number: 5 The largest number is 17
Explanation :
In this approach, user input is read using the Scanner class. Following that, we create three integer variables, a, b, and c, as well as a variable called largest that will hold the largest of the three integers.
Each number is compared to the largest's current value, and if it is greater, largest is updated with the new value. The output is then printed with the value of biggest.
Each number is compared to the largest's current value, and if it is greater, largest is updated with the new value. The output is then printed with the value of biggest.
Example 2 :
Run
public class Main { public static void main(String[] args) { int a = 10, b = 20, c = 30, largest; if (a > b) { if (a > c) { largest = a; } else { largest = c; } } else { if (b > c) { largest = b; } else { largest = c; } } System.out.println("The largest number is " + largest); } }
Output :
The largest number is 30
Explanation :
All triple variables a, b, and c's values are hardcoded in this example. The largest of the three numbers is then chosen using stacked if-else statements.
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others
Login/Signup to comment