Singly Linked List Insertion at beginning in C
Insertion in singly linked list at the beginning
C Program for insertion at the beginning of the Singly Linked List. A Singly linked list is made up of many nodes which are connected. Every node is mainly divided into two parts, one part holds the data and the other part is the link that connects to the next node
Note 1
in the Singly Linked list, we can traverse only in one direction because each node contains the address of the next node only, but not the address of the previous node.
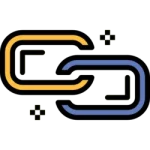
C Program for Insertion at the Beginning of the Singly Linked List:-
Without Interface
With Interface
Without Interface
Run
#include<stdio.h> #include<stdlib.h> struct Node { int data; struct Node *next; }; void insertStart (struct Node **head, int data) { // dynamically create memory for this newNode struct Node *newNode = (struct Node *) malloc (sizeof (struct Node)); // assign data value newNode->data = data; // change the next node of this newNode // to current head of Linked List newNode->next = *head; //re-assign head to this newNode *head = newNode; printf ("%d Insertion Successful\n", newNode->data); } void printLinkedList (struct Node *node) { printf ("\nLinked List: "); // as linked list will end when Node is Null while (node != NULL) { printf ("%d ", node->data); node = node->next; } printf ("\n"); } int main () { struct Node *head = NULL; // Use '&' i.e. address as we need to change head address insertStart (&head, 100); insertStart (&head, 80); insertStart (&head, 60); insertStart (&head, 40); insertStart (&head, 20); // Don't use '&' as not changing head in printLinkedList operation printLinkedList (head); return 0; }
Output
100 Insertion Successful 80 Insertion Successful 60 Insertion Successful 40 Insertion Successful 20 Insertion Successful Linked List: 20 40 60 80 100
With Interface
Run
#include<stdio.h> #include<stdlib.h> struct Node { int data; struct Node *next; }; void insertStart (struct Node **head, int data) { // dynamically create memory for this newNode struct Node *newNode = (struct Node *) malloc (sizeof (struct Node)); // assign data value newNode->data = data; // change the next node of this newNode // to current head of Linked List newNode->next = *head; //re-assign head to this newNode *head = newNode; printf ("%d Insertion Successful\n", newNode->data); } void printLinkedList (struct Node *node) { printf ("\nLinked List: "); // as linked list will end when Node is Null while (node != NULL) { printf ("%d ", node->data); node = node->next; } printf ("\n"); } int main () { struct Node *head = NULL; int input, item; do { printf ("\nEnter the item to insert?\n"); scanf ("%d", &item); // Use '&' i.e. address as we need to change head address insertStart (&head, item); printf ("\nPress 0: To Exit\n"); printf ("Press 1: To insert more items\n"); scanf ("%d", &input); } while (input == 1); // Don't use '&' as not changing head in printLinkedList operation printLinkedList (head); return 0; }
Output
Enter the item to insert? 10 10 Insertion Successful Press 0: To Exit Press 1: To insert more items 1 Enter the item to insert? 20 20 Insertion Successful Press 0: To Exit Press 1: To insert more items 1 Enter the item to insert? 30 30 Insertion Successful Press 0: To Exit Press 1: To insert more items 0 Linked List: 30 20 10
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others
Singly Linked List
- Introduction to Linked List in Data Structure
- Linked List in – C | C++ | Java
- Singly Linked List in – C | C++ | Java
- Insertion in singly Linked List – C | C++ | Java
- Deletion in singly Linked List – C | C++ | Java
- Reverse a linked list without changing links between nodes (Data reverse only) – C | C++ | Java
- Linked List Insertion and Deletion – C | C++ | Java
- Reverse a linked list by changing links between nodes – C | C++ | Java
- Linked List insertion in the middle – C | C++ | Java
- Print reverse of a linked list without actually reversing – C |C++ | Java
- Search an element in a linked list – C | C++ | Java
- Insertion in a Sorted Linked List – C | C++ | Java
- Delete alternate nodes of a Linked List – C | C++ | Java
- Find middle of the linked list – C | C++ | Java
- Reverse a linked list in groups of given size – C | C++ | Java
- Find kth node from end of the linked list – C | C++ | Java
- Append the last n nodes of a linked list to the beginning of the list – C | C++ | Java
- Check whether linked list is palindrome or not – C | C++ | Java
- Fold a Linked List – C | C++ | Java
- Insert at a given position – C | C++ | Java
- Delete at a given position – C | C++ | Java
Singly Linked List
- Introduction to Linked List in Data Structure
Click Here - Linked List in –
- Singly Linked List in –
- Insertion in singly Linked List –
- Insertion at beginning in singly Linked List –
- Insertion at nth position in singly Linked List –
- Insertion at end in singly Linked List –
- Deletion in singly Linked List –
- Deletion from beginning in singly linked list :
- Deletion from nth position in singly linked list :
- Deletion from end in singly linked list :
- Linked List Insertion and Deletion –
C | C++ | Java - Reverse a linked list without changing links between nodes (Data reverse only) –
C | C++ | Java - Reverse a linked list by changing links between nodes –
- Print reverse of a linked list without actually reversing –
- Print reverse of a linked list without actually reversing –
- Insertion in the middle Singly Linked List –
- Insertion in a Sorted Linked List –
- Delete alternate nodes of a Linked List –
- Find middle of the linked list –
- Reverse a linked list in groups of given size –
- Find kth node from end of the linked list –
- Append the last n nodes of a linked list to the beginning of the list –
- Check whether linked list is palindrome or not –
- Fold a Linked List –
- Insert at given Position –
- Deletion at given Position –
Login/Signup to comment