Operators in C++
Operators in C++
C++ supports a rich set of operators. Operators say the compiler to perform mathematical and logical computations on the data stored in memory. Here, on this page, we will discuss different operators in C++.
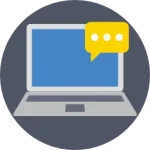
Operators
C++ library has the following –
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
Arithmetic Operators
For a = 100 and b = 50
Operator | Description | Example |
---|---|---|
+ | To add two operands | a + b = 150 |
– | To subtract two operands | a – b = 50 |
* | To multiply two operands | a * b = 5000 |
/ | To divide two operands | a / b = 2 |
% | Modulus operator : To find remainder | a % b = 0 10 % 4 = 2 |
++ | Increment Operator : To increase value by 1 | a++ = 101 |
— | Decrement Operator : To decrease value by 1 | a– = 99 |
Example of above operations –
#include <iostream> using namespace std; int main() { int a, b; a = 20; b = 3; // prints the sum of a & b cout << "a + b = " << (a + b) << endl; // prints the difference between a & b cout << "a - b = " << (a - b) << endl; // prints the multiplication of a & b cout << "a * b = " << (a * b) << endl; // prints the division of a by b // result 6.33 reduced to 6 since both numbers are int result will be int cout << "a / b = " << (a / b) << endl; // prints the remainder when a is divided by b cout << "a % b = " << (a % b) << endl; return 0; }
Output:
a + b = 23 a - b = 17 a * b = 60 a / b = 6 a % b = 2
5/2 is 2
5.0 / 2 is 2.5
5 / 2.0 is 2.5
5.0 / 2.0 is 2.5
Relational Operators
- The output of the relational operators is always in the form of –
- 0 (false)
- 1 or a positive number (true)
- If the condition is satisfied gives 1 and if the condition is false it gives 0
Operator | Name | Example | Result |
---|---|---|---|
> | Greater than | 10 > 5 | true |
>= | Greater than or Equal | 10 >=5 5 >= 5 2 >= 5 | true true false |
< | Lesser than | 10 < 5 | false |
<= | Lesser than or Equal | 10 <= 5 5 <= 5 2 <= 5 | false true true |
==’ | is equals | 10 == 10 100 = 20 | true false |
!=0 | is not equals | 10 != 10 100 != 20 | false true |
C++ program demonstrating Relational operators
#include<iostream> using namespace std; int main() { int a, b; a = 10; b = 5; bool result; result = (a == b); // false cout << "10 == 5 is " << result << endl; result = (a != b); // true cout << "10 != 5 is " << result << endl; result = a > b; // true cout << "10 > 5 is " << result << endl; result = a < b; // false cout << "10 < 5 is " << result << endl; result = a >= b; // true cout << "10 >= 5 is " << result << endl; result = a <= b; // false cout << "10 <= 5 is " << result << endl; return 0; }
Output
10 == 5 is 0 10 != 5 is 1 10 > 5 is 1 10 < 5 is 0 10 >= 5 is 1 10 <= 5 is 0
Logical Operators
- The output of the logical operators is always in the form of 0 (false) and 1 (true)
- A logical operator is a valid combination of values, variables & relational expressions
Operator | Example | Description |
---|---|---|
&& | A && B expression1 && expression 2 | Logical AND. True only if all the operands are true (non zero) |
|| | A || B expression1 || expression 2 | Logical OR. True only if any the operands are true (non zero) |
! | !A | Logical NOT Use to reverses the logical state of its operand. |
Logical approach to demonstrate
Imagine, a = 15 b = 20 // both should be true (a > 5) && (b > 10) // true && true = true (a > 5) && (b < 10) // true && false = false (a < 5) && (b > 10) // false && true = false (a < 5) && (b < 10) // false && false = false // any one or more can be true (a > 5) || (b > 10) // true || true = true (a > 5) || (b < 10) // true || false = true (a < 5) || (b > 10) // false || true = true (a < 5) || (b < 10) // false || false = false !(a < 5) // !(false) = true !(a > 5) // !(true) = false
The following code will help us understand more about these –
#include<iostream> using namespace std; int main() { int a = 20; int b = 10; bool output; output = (a > 5) && (b > 5); // true cout << "(a > 5) && (b > 5) is " << output << endl; output = (a > 0) && (b >= 10); // true cout << "(a > 0) && (b >= 10) is " << output << endl; output = (a != 0) && (b == 0); // false cout << "(a != 0) && (b == 0) is " << output << endl; output = (a != b) || (a < b); // true cout << "(a != b) || (a < b) is " << output << endl; output = (a < b) || (b > 10); // false cout << "(a < b) || (b > 10) is " << output << endl; // both are non zero numbers so both will be true output = (a) && (b); // true cout << "(a) && (b) is " << output << endl; output = !(a == 20); // false cout << "!(a == 20) is " << output << endl; output = !(a < 0); // true cout << "!(a < 0) is " << output << endl; // doing not operation on a non zero value will result to 0 & !(0) // !(a) => !(20) => !(true) => false output = !(a); // false cout << "!(a) is " << output << endl; return 0; }
Output
(a > 5) && (b > 5) is 1 (a > 0) && (b >= 10) is 1 (a != 0) && (b == 0) is 0 (a != b) || (a < b) is 1 (a < b) || (b > 10) is 0 (a) && (b) is 1 !(a == 20) is 0 !(a < 0) is 1 !(a) is 0
Bitwise Operators
Bitwise operators work on individual bits of a number. All numbers are stored in binary format in c++.Example: 10 -> 00001010
Bitwise operators will work on these binary bits. Following are the operators –
- Bitwise AND
- Bitwise OR
- Bitwise XOR
- Bitwise Not or 1’s compliment
- Bitwise shift left
- Bitwise Shirt right
Following is the truth table for common operations –
a | b | a & b | a | b | a ^ b |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 0 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
- A: 0000 1011
- B: 0000 0111
- A & B: 0000 0011
- A | B: 0000 1111
- A ^ B: 0000 1100
- ~A: 1111 0100
- A << 2: 0010 1100
- B >> 2: 0000 0010
Operator | Description | Examples |
---|---|---|
& | Bitwise AND Applies & i.e. AND operator on individual bits for two operands | A & B: 0000 0011 |
| | Bitwise OR Applies | i.e. OR operator on individual bits for two operands | A | B: 0000 1111 Which is 15 in decimal |
^ | Bitwise XOR Applies | i.e. XOR operator on individual bits for two operands | A ^ B: 0000 1100 Which is 12 in decimal |
~ | Bitwise NOT Applies 1 compliment or bitwise NOT on a single operand | ~A: 1111 0100 Which is -12 in decimal (Negative numbers are stored in 2’s compliment, 1st bit shows its negative) |
<< | Bitwise SHIFT Left Shifts all successive bits towards left by ‘x’ bits | A << 2: 0010 1100 Which is 42 in DecimalAll bits of A: 0000 1011 shifted 2 places left |
>> | Bitwise SHIFT Right Shifts all successive bits towards right by ‘x’ bits | A << 2: 0000 0010 Which is 2 in decimalAll bits of A: 0000 1011 shifted 2 places right |
#include<iostream> using namespace std; int main() { int a = 11; // 0000 1011 int b = 7; // 0000 0111 cout << "(a & b) : " << (a & b) << endl; cout << "(a | b) : " << (a | b) << endl; cout << "(a ^ b) : " << (a ^ b) << endl; cout << "(~a) : " << ~a << endl; cout << "(a << 2) : " << (a << 2) << endl; cout << "(a >> 2) : " << (a >> 2) << endl; return 0; }
Output
(a & b) : 3 (a | b) : 15 (a ^ b) : 12 (~a) : -12 (a << 2) : 44 (a >> 2) : 2
Assignment Operators
Assignment operators are used for shortening mathematical assignments.
Operator | Description | Examples |
---|---|---|
= | Simple Assignment Operator | c = a + b |
+= | Shorter form for addition assignment | a = a + b can be written as a += b |
-= | Shorter form for subtraction assignment | a = a – b can be written as a -= b |
*= | Shorter form for multiplication assignment | a = a * b can be written as a *= b |
/= | Shorter form for division assignment | a = a / b can be written as a /= b |
%= | Shorter form for modulo assignment | a = a % b can be written as a %= b |
<<= | Shorter form for shift left assignment | A <<= 2 can be written as A = A << 2 |
>>= | Shorter form for shift right assignment | A >>= 2 can be written as A = A >> 2 |
&= | Shorter form for bitwise AND assignment | a = a & b can be written as a &= b |
^= | Shorter form for bitwise XOR assignment | a = a ^ b can be written as a ^= b |
|= | Shorter form for bitwise OR assignment | a = a | b can be written as a |= b |
Code to demonstrate assignment operators –
#include<iostream> using namespace std; int main() { int a = 10; int b = 5; a += b; // same as a = a + b | a becomes 15 cout << a << endl; a /= b; // same as a = a / b | a becomes 3 cout << a << endl; return 0; }
Output
15 3
Ternary Operator
The conditional operator is a decision-making operator whose statement is evaluated based on the test conditionSyntax:
(Test condition)? (Do this if True) : (Do this is False)If the condition is true statement 1 is evaluated and if it is false, statement 2 is evaluated
Test whether a number is even or odd using the ternary operator
#include<iostream> using namespace std; int main() { int n; cout << "Enter a number:" << endl; cin >> n; //using ternary operator // modulo operator to check remainder (n % 2 == 0) ? cout << "Even": cout << "Odd"; return 0; }
Output
Enter a number: 10 Even
Find the maximum of three numbers using the ternary operator
#include<iostream> using namespace std; int main() { int a, b, result; cout<<"Enter two numbers :"; cin >> a >> b; result = a > b ? a : b; cout<<"Larger: "<< result; return 0; }
Enter two numbers :10 20 Larger: 20
Comma Operator
The comma operator is a special operator which evaluates statements from left to right and returns the rightmost expression as the final resultC++ program to demonstrate comma operator
#include&t;iostream> using namespace std; int main() { int a = 1, b = 2, c; c = (a = a + 2, b = a + 3, b = a + b); // comma operator association is left to right so // left operations happen first and then right // initially a = a + 2 is evaluated (a = 1 + 2) which makes a : 3 then // b = a + 3 is evaluated (b = 3 + 3) which makes b : 6 // finally b = a + b is evaluated (b = 3 + 6) which b : 9 // this final value is returned to assignment c = return value 9 cout << c; // 9 return 0; }
Output
9
Increment/Decrement operators
The ++ and — operators add 1 and subtract 1 from the existing value at the memory locationPost increment/decrement
In a single execution line assignment may happen first and increment/decrement may happen later#include<iostream> using namespace std; int main() { int a = 10; int b = 20; int result; // demonstrating post increment operator // value is assigned first and then incremented later result = a++; cout << "a: " << a << ", res: " << result << endl; // value is assigned first and then incremented later result = b--; cout << "b: " << b << ", res: " << result << endl; return 0; }
Output
a: 11, res: 10 b: 19, res: 20
Pre increment/decrement
Increment happens first and assignment happens later#include<iostream> using namespace std; int main() { int a = 10; int b = 20; int result; // demonstrating pre increment operator // value is incremented first and assigned later result = ++a; cout << "a: " << a << ", res: " << result << endl; // value is assigned first and then incremented later result = --b; cout << "b: " << b << ", res: " << result << endl; return 0; }
Output
a: 11, res: 11 b: 19, res: 19
Cleary understand the diffeernce between pre and post inc/dec operators
Below can be an interesting program to learn new things –#include<iostream> using namespace std; int main() { int a = 5; cout << "++a :" << ++a << "\ta: " << a << endl; cout << "--a :" << --a << "\ta: " << a << endl; cout << "a++: " << a++ << "\ta: " << a << endl; cout << "a--: " << a-- << "\ta: " << a << endl; return 0; }
Output
++a :6 a: 6 --a :5 a: 5 a++: 5 a: 6 a--: 6 a: 5
Precedence
Operator precedence gives priorities to operators while evaluating an expression
For example: when 2 * 3 + 2 is evaluated output is 8 but not 12 because the * operator is having more priority than + hence 2 * 3 is evaluated first followed by 6 + 2.
Operator precedence table
- The operator precedence table gives the detailed list of priorities for each and every operator
- Operators are listed from higher priority to lower
Precedence | Operator | Description | Associativity |
---|---|---|---|
1 | :: | Scope resolution | Left-to-right |
2 | ++ -- | Suffix/postfix increment and decrement | |
type() type{} | Function-style typecast | ||
() | Function call | ||
[] | Array subscripting | ||
. | Element selection by reference | ||
-> | Element selection through pointer | ||
3 | ++ -- | Prefix increment and decrement | Right-to-left |
+ - | Unary plus and minus | ||
! ~ | Logical NOT and bitwise NOT | ||
(type) | C-style type cast | ||
* | Indirection (dereference) | ||
& | Address-of | ||
sizeof | Size-of | ||
new , new[] | Dynamic memory allocation | ||
delete , delete[] | Dynamic memory deallocation | ||
4 | .* ->* | Pointer to member | Left-to-right |
5 | * / % | Multiplication, division, and remainder | |
6 | + - | Addition and subtraction | |
7 | << >> | Bitwise left shift and right shift | |
8 | < <= | For relational operators < and ≤ respectively | |
> >= | For relational operators > and ≥ respectively | ||
9 | == != | For relational = and ≠ respectively | |
10 | & | Bitwise AND | |
11 | ^ | Bitwise XOR (exclusive or) | |
12 | | | Bitwise OR (inclusive or) | |
13 | && | Logical AND | |
14 | || | Logical OR | |
15 | ?: | Ternary conditional operator | Right-to-left |
= | Direct assignment (provided by default for C++ classes) | ||
+= -= | Assignment by sum and difference | ||
*= /= %= | Assignment by product, quotient, and remainder | ||
<<= >>= | Assignment by bitwise left shift and right shift | ||
&= ^= |= | Assignment by bitwise AND, XOR, and OR | ||
16 | throw | Throw operator (for exceptions) | |
17 | , | Comma | Left-to-right |
Example1
Evaluate 5*4+(3+2)
- Parenthesis is having the highest priority
5*4+5 - Among * and +,* is having the highest priority
20 + 5= 25 is the final output
Example 2
Arithmetic operators for having higher priority than relational operators
Observe the difference With Parenthesis and without Parenthesis
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others
Login/Signup to comment