Python Program to sort the elements of an array
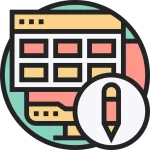
Sort the Array in Python
Here, in this page we will discuss the program to sort elements of the given array in Python programming language. We will discuss the program to sort the given input array using inbuilt sort function.
Here, in this page we will sort the given input array but not only in ascending order, but also in descending order.
Example :
- Input : arr[5] = [10, 40, 20, 30]
- Output : In ascending order = 10 20 30 40
In descending order = 40 30 20 10
Method 1 (Sort in ascending order):
In this method we will sort the given input array using inbuilt sort function.
- Declare an array.
- Call sort() function i.e, arr.sort()
This will sort the given input array in ascending order. We will also discuss to sort the given input array in descending order as well.
sort() function
The sort() method is a built-in Python method that, by default, sorts the list in ascending order.However, you can modify the order from ascending to descending by specifying the sorting criteria.
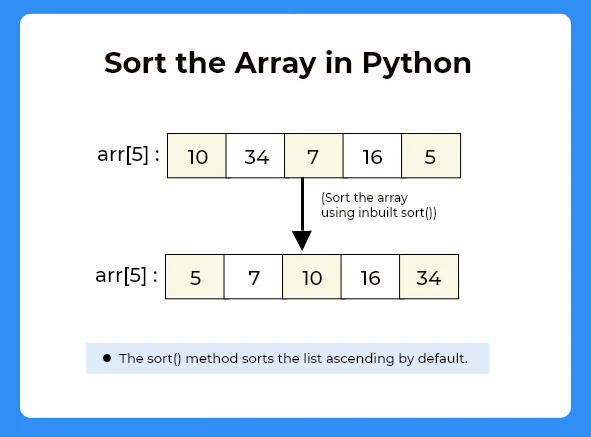
Code to Sort the Array in Python
# List of Integers numbers = [10, 30, 40, 20] # Sorting list of Integers numbers.sort() print(numbers)
Output
[10, 20, 30, 40]
Method 2 (Sort in descending order):
In this method we will sort the given input array using inbuilt sort function.
- Declare an array.
- Call sort() function i.e, arr.sort(reverse=True)
This will sort the given input array in descending order.
reverse parameter
On passing parameter reverse with value True, i.e, reverse-True : Then the array will get sorted in descending orderCode to Sort the Array in Python
# List of Integers numbers = [10, 30, 40, 20] # Sorting list of Integers numbers.sort(reverse=True) print(numbers)
Output
[40, 30, 20, 10]
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
a = [10, 20, 5, 3, 40, 2, 11]
n = len(a)
for i in range(0, n):
for j in range(i + 1, n):
if a[i] > a[j]:
temp = a[i]
a[i] = a[j]
a[j] = temp
print(a)
#decending order
a1 = list(map(int,input().split(“,”)))
a1.sort(reverse = True);
print(a1);
**Asscending order
a1 = list(map(int,input().split(“,”)))
a1.sort();
print(a1);
n = int(input())
arr = []
for i in range(n):
e = int(input())
arr.append(e)
print(arr)
arr.sort()
print(“A.O.”,arr)
arr.sort(reverse=True)
print(“D.O.”,arr)