Finding the frequency of element Frequency of elements in an array using Python
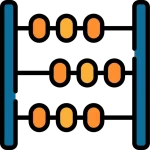
Frequency of Elements in an Array in Python
Here, in this page we will discuss the program to count the frequency of elements in an array in python programming language. We are given with an array and need to print each element along its frequency.
Methods Discussed are :
- Method 1 : Naive way using extra space.
- Method 2 : Naive way without using extra space.
- Method 3 : Using Sorting
- Method 4 : Using hash Map
Let’s discuss each method one by one,
Method 1 :
In this method we will count the frequency of each elements using two for loops.
- To check the status of visited elements create a array of size n.
- Run a loop from index 0 to n and check if (visited[i]==1) then skip that element.
- Otherwise create a variable count = 1 to keep the count of frequency.
- Run a loop from index i+1 to n
- Check if(arr[i]==arr[j]), then increment the count by 1 and set visited[j]=1.
- After complete iteration of for loop print element along with value of count.
Time and Space Complexity :
- Time Complexity : O(n2)
- Space Complexity : O(n)
Method 1 : Code in Python
Run
# Python 3 program to count frequencies # of array items def countFreq(arr, n): # Mark all array elements as not visited visited = [False for i in range(n)] # Traverse through array elements # and count frequencies for i in range(n): # Skip this element if already # processed if (visited[i] == True): continue # Count frequency count = 1 for j in range(i + 1, n, 1): if (arr[i] == arr[j]): visited[j] = True count += 1 print(arr[i], count) # Driver Code arr = [10, 30, 10, 20, 10, 20, 30, 10] n = len(arr) countFreq(arr, n)
Output
10 4
30 2
20 2
Method 2 :
In this method we will use the naive way to find the frequency of elements in the given integer array without using any extra space.
Method 2 : Code in python
Run
# Time Complexity : O(n^2) # Space Complexity : O(1) def countFrequency(arr, size): for i in range(0, size): flag = False count = 0 for j in range(i+1, size): if arr[i] == arr[j]: flag = True break # The continue keyword is used to end the current iteration # in a for loop (or a while loop), and continues to the next iteration. if flag == True: continue for j in range(0, i+1): if arr[i] == arr[j]: count += 1 print("{0}: {1}".format(arr[i], count)) # Driver Code arr = [5, 8, 5, 7, 8, 10] n = len(arr) countFrequency(arr, n)
Output
5 : 2
7 : 1
8 : 2
10 : 1
Method 3 :
In this method we will sort the array then, count the frequency of the elements.
Time and Space Complexity :
- Time Complexity : O(nlogn)
- Space Complexity : O(1)
Method 3 : Code in Python
Run
# Time Complexity : O(n log n) + O(n) = O(n logn) # Space Complexity : O(1) def countDistinct(arr, n): arr.sort() # Traverse the sorted array i = 0 while i < n: count = 1 # Move the index ahead whenever # you encounter duplicates while i < n - 1 and arr[i] == arr[i + 1]: i += 1 count +=1 print("{0}: {1}".format(arr[i], count)) i += 1 # Driver Code arr = [5, 8, 5, 7, 8, 10] n = len(arr) countDistinct(arr, n)
Output
5 : 2
7 : 1
8 : 2
10 : 1
Method 4 :
In this method we will count use hash-map to count the frequency of each elements.
- Declare a dictionary dict().
- Start iterating over the entire array
- If element is present in map, then increase the value of frequency by 1.
- Otherwise, insert that element in map.
- After complete iteration over array, start traversing map and print the key-value pair.
Time and Space Complexity :
- Time Complexity : O(n)
- Space Complexity : O(n)
Dictionary in Python
Dictionary in Python is an unordered collection of data values, used to store data values like a map, which, unlike other Data Types that hold only a single value as an element, Dictionary holds key:value pair.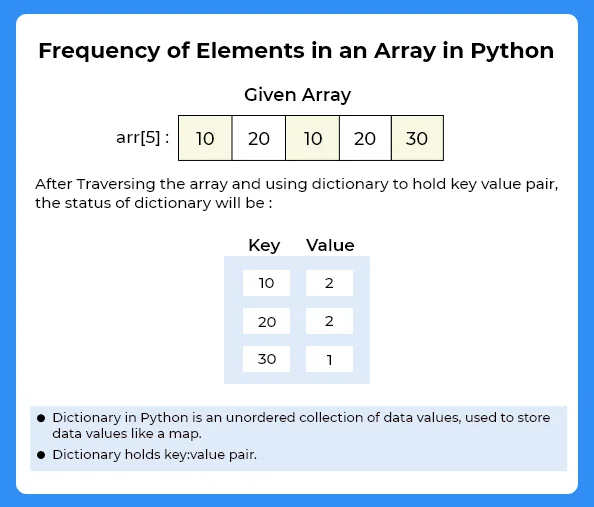
Method 4 : Code in Python
def countFreq(arr, n): mp = dict() # Traverse through array elements # and count frequencies for i in range(n): if arr[i] in mp.keys(): mp[arr[i]] += 1 else: mp[arr[i]] = 1 # Traverse through map and print # frequencies for x in mp: print(x, " ", mp[x]) # Driver Code arr = [10, 30, 10, 20, 10, 20, 30, 10] n = len(arr) countFreq(arr, n)
Output
10 4
20 2
30 2
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Login/Signup to comment
arr = [10, 30, 10, 20, 10, 20, 30, 10]
dict={}
for i in arr:
if i in dict:
dict[i]=dict[i]+1
else:
dict[i]=1
print(dict)
Kindly join our Discord Channel for technical queries.
lst = [10, 20, 40, 30, 50, 20, 10, 20]
out=[]
c = 0
for i in lst:
if i not in out:
out+=[i]
for i in out:
c = lst.count(i)
print(i,c)
#frequency of elemt in a list
l=[1,2,3,1,2,5,2,1,3,1,3,4,2,7,1,3,5,6,1,3,7]
print(set(l))
l2=[]
for i in range(0,len(l)):
if l[i] not in l2:
count=0
for j in range(0,len(l)):
if l[i]==l[j]:
count+=1
print(l[i],count)
l2.append(l[i])
arr = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
freq = {}
for i in arr:
if i in freq:
freq[i] += 1
else:
freq[i] = 1
print(freq)
PrepInsta
Feed
Prime
Notifications
Finding the frequency of element Frequency of elements in an array using Python
Frequency of Elements in an Array in Python
Here, in this page we will discuss the program to count the frequency of elements in an array in python programming language. We are given with an array and need to print each element along its frequency.
Methods Discussed are :
Method 1 : Naive way using extra space.
Method 2 : Naive way without using extra space.
Method 3 : Using Sorting
Method 4 : Using hash Map
Let’s discuss each method one by one,
Method 1 :
In this method we will count the frequency of each elements using two for loops.
To check the status of visited elements create a array of size n.
Run a loop from index 0 to n and check if (visited[i]==1) then skip that element.
Otherwise create a variable count = 1 to keep the count of frequency.
Run a loop from index i+1 to n
Check if(arr[i]==arr[j]), then increment the count by 1 and set visited[j]=1.
After complete iteration of for loop print element along with value of count.
Time and Space Complexity :
Time Complexity : O(n2)
Space Complexity : O(n)
Method 1 : Code in Python
Run
# Python 3 program to count frequencies
# of array items
def countFreq(arr, n):
# Mark all array elements as not visited
visited = [False for i in range(n)]
# Traverse through array elements
# and count frequencies
for i in range(n):
# Skip this element if already
# processed
if (visited[i] == True):
continue
# Count frequency
count = 1
for j in range(i + 1, n, 1):
if (arr[i] == arr[j]):
visited[j] = True
count += 1
print(arr[i], count)
# Driver Code
arr = [10, 30, 10, 20, 10, 20, 30, 10]
n = len(arr)
countFreq(arr, n)
Output
10 4
30 2
20 2
Related Pages
Sort first half in ascending order and second half in descending
Sort the elements of an array
Sorting elements of an array by frequency
Finding the Longest Palindrome in an Array
Counting Distinct Elements in an Array
Method 2 :
In this method we will use the naive way to find the frequency of elements in the given integer array without using any extra space.
Method 2 : Code in python
Run
# Time Complexity : O(n^2)
# Space Complexity : O(1)
def countFrequency(arr, size):
for i in range(0, size):
flag = False
count = 0
for j in range(i+1, size):
if arr[i] == arr[j]:
flag = True
break
# The continue keyword is used to end the current iteration
# in a for loop (or a while loop), and continues to the next iteration.
if flag == True:
continue
for j in range(0, i+1):
if arr[i] == arr[j]:
count += 1
print(“{0}: {1}”.format(arr[i], count))
# Driver Code
arr = [5, 8, 5, 7, 8, 10]
n = len(arr)
countFrequency(arr, n)
Output
5 : 2
7 : 1
8 : 2
10 : 1
Method 3 :
In this method we will sort the array then, count the frequency of the elements.
Time and Space Complexity :
Time Complexity : O(nlogn)
Space Complexity : O(1)
Method 3 : Code in Python
Run
# Time Complexity : O(n log n) + O(n) = O(n logn)
# Space Complexity : O(1)
def countDistinct(arr, n):
arr.sort()
# Traverse the sorted array
i = 0
while i < n:
count = 1
# Move the index ahead whenever
# you encounter duplicates
while i < n – 1 and arr[i] == arr[i + 1]:
i += 1
count +=1
print("{0}: {1}".format(arr[i], count))
i += 1
# Driver Code
arr = [5, 8, 5, 7, 8, 10]
n = len(arr)
countDistinct(arr, n)
Output
5 : 2
7 : 1
8 : 2
10 : 1
Method 4 :
In this method we will count use hash-map to count the frequency of each elements.
Declare a dictionary dict().
Start iterating over the entire array
If element is present in map, then increase the value of frequency by 1.
Otherwise, insert that element in map.
After complete iteration over array, start traversing map and print the key-value pair.
Time and Space Complexity :
Time Complexity : O(n)
Space Complexity : O(n)
Dictionary in Python
Dictionary in Python is an unordered collection of data values, used to store data values like a map, which, unlike other Data Types that hold only a single value as an element, Dictionary holds key:value pair.
Frequency of element
Method 4 : Code in Python
def countFreq(arr, n):
mp = dict()
# Traverse through array elements
# and count frequencies
for i in range(n):
if arr[i] in mp.keys():
mp[arr[i]] += 1
else:
mp[arr[i]] [2,2,2,2,2,2,2,2,45,7,8,9,6,3,666]
n=int(input(“enter a number from the list”))
freq=0
for i in arr:
if n ==i:
freq+=1
else:
pass
print(“the number of times {} occurred is {}”.format(n,freq))
0Reply ↓
Sanjeet //Try this in C : Program to find frequency of an array
#include
int main()
{
int arr[100],n,i,j;
printf(“Enter the size of array: “);
scanf(“%d”,&n);
printf(“Enter value in array: \n”);
for(i=0;i<n;i++)
{
scanf("%d",&arr[i]);
}
for(i=0;i<n;i++)
{
int count=1;
if(arr[i]!=-1)
{
for(j=i+1;j<n;j++)
{
if(arr[i]==arr[j])
{
count=count+1;
arr[j]=-1;
}
}
printf("The count of %d is %d\n",arr[i],count);
arr[i]=-1;
}
}
return 0;
}
PrepInsta
Feed
Prime
Notifications
Finding the frequency of element Frequency of elements in an array using Python
Frequency of Elements in an Array in Python
Here, in this page we will discuss the program to count the frequency of elements in an array in python programming language. We are given with an array and need to print each element along its frequency.
Methods Discussed are :
Method 1 : Naive way using extra space.
Method 2 : Naive way without using extra space.
Method 3 : Using Sorting
Method 4 : Using hash Map
Let’s discuss each method one by one,
Method 1 :
In this method we will count the frequency of each elements using two for loops.
To check the status of visited elements create a array of size n.
Run a loop from index 0 to n and check if (visited[i]==1) then skip that element.
Otherwise create a variable count = 1 to keep the count of frequency.
Run a loop from index i+1 to n
Check if(arr[i]==arr[j]), then increment the count by 1 and set visited[j]=1.
After complete iteration of for loop print element along with value of count.
Time and Space Complexity :
Time Complexity : O(n2)
Space Complexity : O(n)
Method 1 : Code in Python
Run
# Python 3 program to count frequencies
# of array items
def countFreq(arr, n):
# Mark all array elements as not visited
visited = [False for i in range(n)]
# Traverse through array elements
# and count frequencies
for i in range(n):
# Skip this element if already
# processed
if (visited[i] == True):
continue
# Count frequency
count = 1
for j in range(i + 1, n, 1):
if (arr[i] == arr[j]):
visited[j] = True
count += 1
print(arr[i], count)
# Driver Code
arr = [10, 30, 10, 20, 10, 20, 30, 10]
n = len(arr)
countFreq(arr, n)
Output
10 4
30 2
20 2
Related Pages
Sort first half in ascending order and second half in descending
Sort the elements of an array
Sorting elements of an array by frequency
Finding the Longest Palindrome in an Array
Counting Distinct Elements in an Array
Method 2 :
In this method we will use the naive way to find the frequency of elements in the given integer array without using any extra space.
Method 2 : Code in python
Run
# Time Complexity : O(n^2)
# Space Complexity : O(1)
def countFrequency(arr, size):
for i in range(0, size):
flag = False
count = 0
for j in range(i+1, size):
if arr[i] == arr[j]:
flag = True
break
# The continue keyword is used to end the current iteration
# in a for loop (or a while loop), and continues to the next iteration.
if flag == True:
continue
for j in range(0, i+1):
if arr[i] == arr[j]:
count += 1
print(“{0}: {1}”.format(arr[i], count))
# Driver Code
arr = [5, 8, 5, 7, 8, 10]
n = len(arr)
countFrequency(arr, n)
Output
5 : 2
7 : 1
8 : 2
10 : 1
Method 3 :
In this method we will sort the array then, count the frequency of the elements.
Time and Space Complexity :
Time Complexity : O(nlogn)
Space Complexity : O(1)
Method 3 : Code in Python
Run
# Time Complexity : O(n log n) + O(n) = O(n logn)
# Space Complexity : O(1)
def countDistinct(arr, n):
arr.sort()
# Traverse the sorted array
i = 0
while i < n:
count = 1
# Move the index ahead whenever
# you encounter duplicates
while i < n – 1 and arr[i] == arr[i + 1]:
i += 1
count +=1
print("{0}: {1}".format(arr[i], count))
i += 1
# Driver Code
arr = [5, 8, 5, 7, 8, 10]
n = len(arr)
countDistinct(arr, n)
Output
5 : 2
7 : 1
8 : 2
10 : 1
Method 4 :
In this method we will count use hash-map to count the frequency of each elements.
Declare a dictionary dict().
Start iterating over the entire array
If element is present in map, then increase the value of frequency by 1.
Otherwise, insert that element in map.
After complete iteration over array, start traversing map and print the key-value pair.
Time and Space Complexity :
Time Complexity : O(n)
Space Complexity : O(n)
Dictionary in Python
Dictionary in Python is an unordered collection of data values, used to store data values like a map, which, unlike other Data Types that hold only a single value as an element, Dictionary holds key:value pair.
Frequency of element
Method 4 : Code in Python
def countFreq(arr, n):
mp = dict()
# Traverse through array elements
# and count frequencies
for i in range(n):
if arr[i] in mp.keys():
mp[arr[i]] += 1
else:
mp[arr[i]] = 1
# Traverse through map and print
# frequencies
for x in mp:
print(x, " ", mp[x])
# Driver Code
arr = [10, 30, 10, 20, 10, 20, 30, 10]
n = len(arr)
countFreq(arr, n)
Output
10 4
20 2
30 2
Logged in as MYTHILIESVARAN. Log out?
Enter comment here…
SUMAN def frequency(arr,n):
visited = [False for i in range(n)];
for i in range(n):
if (visited[i] == True) :
continue count = 1;
for j in range(i+1,n,1):
if (arr[i] == arr[j]) :
visited[j] = True
count += 1;
print(arr[i],count);
a1 = list(map(int,input().split(“,”)))
l = len(a1);
frequency(a1,l)
1Reply ↓
dileep arr=[2,2,2,2,2,2,2,2,45,7,8,9,6,3,666]
n=int(input(“enter a number from the list”))
freq=0
for i in arr:
if n ==i:
freq+=1
else:
pass
print(“the number of times {} occurred is {}”.format(n,freq))
0Reply ↓
Sanjeet //Try this in C : Program to find frequency of an array
#include
int main()
{
int arr[100],n,i,j;
printf(“Enter the size of array: “);
scanf(“%d”,&n);
printf(“Enter value in array: \n”);
for(i=0;i<n;i++)
{
scanf("%d",&arr[i]);
}
for(i=0;i<n;i++)
{
int count=1;
if(arr[i]!=-1)
{
for(j=i+1;j<n;j++)
{
if(arr[i]==arr[j])
{
count=count+1;
arr[j]=-1;
}
}
printf("The count of %d is %d\n",arr[i],count);
arr[i]=-1;
}
}
return 0;
}
import collections
arr=[5, 8, 5, 7, 8, 10]
x=collections.Counter(arr)
for v in x.keys():
print(v,” “,x[v])
#count of elements in array
arr=[10,20,30,10,10,10,20,30,20,30,30]
d={}.fromkeys(arr,0)
for i in arr:
d[i]=arr.count(i)
print(d)
for i,j in d.items():
print(i,j)
arr=[2,2,2,3,12,3,2344,22,12,12,10,2432]
l1=list(set(arr))
for i in l1:
z=l1.count(i)
print(i,z)
def frequency(arr,n):
visited = [False for i in range(n)];
for i in range(n):
if (visited[i] == True) :
continue
count = 1;
for j in range(i+1,n,1):
if (arr[i] == arr[j]) :
visited[j] = True
count += 1;
print(arr[i],count);
a1 = list(map(int,input().split(“,”)))
l = len(a1);
frequency(a1,l)
arr=[2,2,2,2,2,2,2,2,45,7,8,9,6,3,666]
n=int(input(“enter a number from the list”))
freq=0
for i in arr:
if n ==i:
freq+=1
else:
pass
print(“the number of times {} occurred is {}”.format(n,freq))
//Try this in C : Program to find frequency of an array
#include
int main()
{
int arr[100],n,i,j;
printf(“Enter the size of array: “);
scanf(“%d”,&n);
printf(“Enter value in array: \n”);
for(i=0;i<n;i++)
{
scanf("%d",&arr[i]);
}
for(i=0;i<n;i++)
{
int count=1;
if(arr[i]!=-1)
{
for(j=i+1;j<n;j++)
{
if(arr[i]==arr[j])
{
count=count+1;
arr[j]=-1;
}
}
printf("The count of %d is %d\n",arr[i],count);
arr[i]=-1;
}
}
return 0;
}