JAVA LinkedList
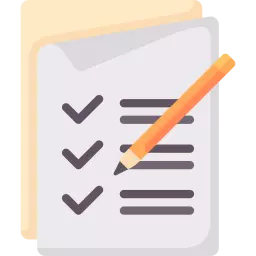
What is Java Linked List?
Java LinkedList is a class in Java’s Collection Framework that provides a linked list implementation of the List interface. The LinkedList class stores its elements in nodes, where each node contains both the element and a reference to the next node in the list.
This means that each element in the list is not stored in contiguous memory, unlike the ArrayList. Instead, the elements are connected through references. To Understand the Java LinkedList, Read the Java complete article.
Creating a Java LinkedList
- To create a LinkedList in Java, you need to do the following steps:
- Import the LinkedList class from the java.util package.
- Create an instance of the LinkedList class using the new keyword.
- Add elements to the LinkedList using the add() method.
Example 1:Java program for Creation Of Java LinkedList.
//Creation of Java Linked List import java.util.LinkedList; public class Main { public static void main (String[]args) { LinkedList < String > names = new LinkedList <> (); // Adding elements to the list names.add ("Ashish"); names.add ("anjali"); names.add ("vibudh"); // Printing the list to the console System.out.println (names); } }
Output
[Ashish, anjali, vibudh]
Method | Description |
---|---|
contains(Object o) | Returns true if the list contains the specified element. |
indexOf(Object o) | Returns the index of the first occurrence of the specified element in the list, or -1 if the element is not found. |
lastIndexOf(Object o) | Returns the index of the last occurrence of the specified element in the list, or -1 if the element is not found. |
iterator() | Returns an iterator over the elements in the list |
listIterator() | Returns a list iterator over the elements in the list. |
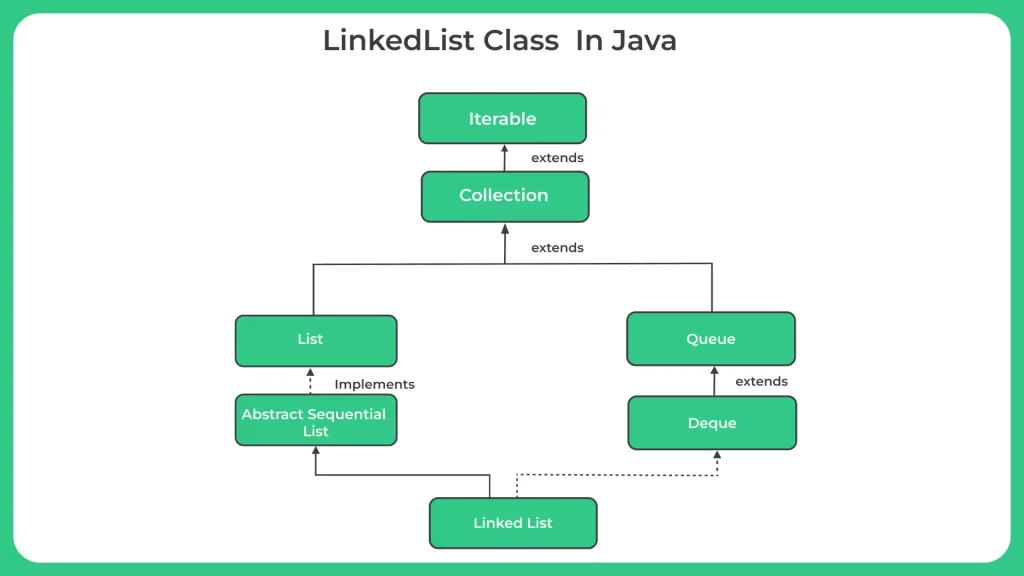
Let’s look at the Java LinkedList to perform certain operations.
Example 1:Java program to Insert Elements to LinkedHashSet.
//AddElementsToLinkedList import java.util.LinkedList; public class Main { public static void main (String[]args) { LinkedList < String > linkedList = new LinkedList <> (); // add elements to the linked list linkedList.add ("Bat"); linkedList.add ("Ball"); linkedList.add ("wicket"); // print the contents of the linked list System.out.println ("LinkedList: " + linkedList); } }
Output
LinkedList: [Bat, Ball, wicket]
Example 2 : Java program to Access LinkedList Elements
//AccessLinkedListElements import java.util.LinkedList; public class Main { public static void main(String[] args) { LinkedListlinkedList = new LinkedList<>(); // add elements to the linked list linkedList.add("Nokia"); linkedList.add("Samsung"); linkedList.add("Motorola"); // access the first element in the linked list String firstElement = linkedList.get(0); System.out.println("First Element: " + firstElement); // access the second element in the linked list String secondElement = linkedList.get(1); System.out.println("Second Element: " + secondElement); // access the third element in the linked list String thirdElement = linkedList.get(2); System.out.println("Third Element: " + thirdElement); } }
Output
First Element: Nokia Second Element: Samsung Third Element: Motorola
Example 3 : Java program to Remove Elements from LinkedList
import java.util.LinkedList; //RemoveFromLinkedList public class Main { public static void main(String[] args) { LinkedListlinkedList = new LinkedList<>(); // add elements to the linked list linkedList.add("Boat"); linkedList.add("Bike"); linkedList.add("Airplane"); // print the contents of the linked list before removing an element System.out.println("LinkedList: " + linkedList); // remove the second element from the linked list linkedList.remove(1); // print the contents of the linked list after removing an element System.out.println("LinkedList after removing second element: " + linkedList); } }
Output
LinkedList: [Boat, Bike, Airplane] LinkedList after removing second element: [Boat, Airplane]
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others
Login/Signup to comment