Technical Interview Preparation
- Technical Interview Questions
- HTML Interview Questions
- CSS Interview Questions
- JavaScript Interview Questions
- React JS Interview Questions
- Node.js interview questions
- MERN Stack Interview Questions
- Data Analytics Interview Questions
- C++ Technical Interview Questions
- Python Interview Questions
- Java Interview Question
- Most Asked Coding Question
- DSA interview questions
- Computer Network Interview Question
- Cloud Computing Interview Question
- Other Related Links
MERN Stack Interview Questions
MERN Stack Interview Questions and Answers
MERN Stack Interview Questions – MERN Stack (MongoDB, Express.js, React, and Node.js) is one of the most popular technology stacks for web development. Many companies use it to build modern, high-performing applications.
If you are preparing for a MERN Stack interview questions asked in the interview, you need to be familiar with the key concepts and commonly asked questions.
This blog will help you by providing important MERN Stack Interview Questions that will enhance your confidence and improve your chances to crack any exam.
MERN Stack Interview Questions Asked
MERN Stack is a collection of technologies used to build full-stack web applications. It consists of four main components:
- MongoDB – A NoSQL database that stores data in JSON-like format.
- Express.js – A backend framework for building web applications and APIs using Node.js.
- React.js – A JavaScript library for creating interactive and dynamic user interfaces.
- Node.js – A JavaScript runtime that allows running JavaScript code outside the browser.
In simple words, MERN Stack helps developers build websites and applications using JavaScript for both the front end (React) and back end (Node.js and Express), while MongoDB stores the data.
Outline
Most Important concepts to keep in Mind
To prepare for MERN Stack Interview Questions, you should first focus on MongoDB on these key topics:
MongoDB
- Understanding NoSQL databases
- CRUD operations (Create, Read, Update, Delete)
- Schema design and relationships
- Aggregation framework
MongoDB Interview Questions and Answers for Freshers and Experienced
Ques 1: What is MongoDB, and what are its key features?
Ans.
MongoDB is an open-source, document-oriented NoSQL database designed for high performance, scalability, and flexibility. Unlike traditional relational databases that store data in tables, MongoDB stores data in JSON-like documents with dynamic schemas, making it easier to model and manipulate data.
Key features include:
- Flexible Schema: Allows for the storage of documents without a predefined structure, accommodating changes in data requirements over time.
- Scalability: Supports horizontal scaling through sharding, distributing data across multiple servers to handle large datasets and high throughput.
- High Performance: Optimized for read and write operations, making it suitable for applications requiring fast data access.
- Replication: Ensures data availability and redundancy through replica sets, which provide automatic failover and data recovery.
- Rich Query Language: Offers a powerful query language with support for ad-hoc queries, indexing, and real-time aggregation.
Ques 2: Explain the concept of a replica set in MongoDB.
Ans.
A replica set in MongoDB is a group of mongod instances that maintain the same dataset, providing redundancy and high availability. A typical replica set consists of:
- Primary Node: Handles all write operations and records all changes to the data.
- Secondary Nodes: Replicate the data from the primary node and can serve read operations, depending on the read preference configuration.
In the event of a primary node failure, an automatic election process selects a new primary from the secondary nodes, ensuring continuous availability without manual intervention.
Ques 3: What is sharding in MongoDB, and when would you use it?
Ans.
Sharding is MongoDB’s method for distributing data across multiple servers or clusters to support deployments with large data sets and high throughput operations. It involves partitioning data into smaller, more manageable pieces called shards. Sharding is particularly beneficial when:
- The dataset size exceeds the storage capacity of a single server.
- The read/write operations demand more resources than a single server can provide.
- You need to ensure high availability and scalability of the database.
By distributing data across multiple shards, MongoDB ensures balanced workloads and efficient query performance.
Ques 4: How does MongoDB handle data consistency?
Ans.
MongoDB provides strong consistency by default for read and write operations on the primary node. When a client sends a write operation, it is applied to the primary node, and then replicated to secondary nodes. To ensure data consistency across the replica set, MongoDB offers write concerns and read preferences:
- Write Concerns: Define the level of acknowledgment required from MongoDB when performing write operations. For example, a write concern of w: “majority” ensures that the write is acknowledged by the majority of replica set members before considering it successful.
- Read Preferences: Determine from which members of a replica set read operations retrieve data. The default is to read from the primary, but it can be configured to read from secondary nodes, which might return stale data depending on replication lag.
By configuring these settings appropriately, applications can balance between consistency, availability, and performance based on their specific requirements.
Ques 5: Describe the Aggregation Framework in MongoDB.
Ans.
The Aggregation Framework in MongoDB is a powerful tool for performing data processing and analysis directly within the database. It allows for the transformation and computation of data using a pipeline approach, where documents pass through a series of stages that filter, sort, group, reshape, and modify the data. Key components include:
- Stages: Each stage performs an operation on the data, such as $match for filtering documents, $group for grouping documents by a specified expression, and $project for reshaping documents.
- Pipelines: A sequence of stages through which the data passes. The output of one stage becomes the input for the next, allowing for complex data transformations.
For example, to calculate the total sales for each product category, you might use the following aggregation pipeline:
db.sales.aggregate([ { $match: { status: "completed" } }, { $group: { _id: "$category", totalSales: { $sum: "$amount" } } }, { $sort: { totalSales: -1 } } ]);
This pipeline filters sales to include only completed transactions, groups them by category, sums the sales amounts for each category, and sorts the results in descending order of total sales.
Ques 6: What are indexes in MongoDB, and how do they improve query performance?
Ans.
Indexes in MongoDB are special data structures that store a small portion of the dataset in an easy-to-traverse form, representing the value of a specific field or set of fields. They significantly enhance query performance by allowing the database to quickly locate and access the required data without scanning the entire collection.
For example, creating an index on the username field in a users collection can be done as follows:
db.users.createIndex({ username: 1 });
- This creates an ascending index on the username field.
- With this index in place, queries searching for a specific username can retrieve results much faster, as MongoDB can directly navigate to the relevant entries instead of performing a full collection scan.
However, while indexes improve read performance, they do introduce some overhead for write operations, as the indexes need to be updated with each insert or update.
Ques 7: Explain the purpose of the ObjectId in MongoDB
Ans.
In MongoDB, every document stored in a collection requires a unique identifier, known as the _id field. By default, MongoDB assigns a unique ObjectId to this field if no custom _id is provided.
An ObjectId is a 12-byte identifier that ensures uniqueness across different collections and databases. It consists of:
- 4 bytes representing the timestamp when the ObjectId was created.
- 5 bytes representing a randomly generated machine identifier.
- 2 bytes representing the process ID of the MongoDB server.
- 3 bytes representing an incrementing counter.
Using ObjectId ensures uniqueness while keeping storage efficient. You can retrieve the timestamp of an ObjectId like this:
var objectId = ObjectId("654abc123def4567890"); print(objectId.getTimestamp());
This makes ObjectId useful for tracking record creation time and ensuring distributed uniqueness.
Ques 8: What is the difference between find() and findOne() in MongoDB?
Ans.
The find() method in MongoDB retrieves multiple documents that match the given criteria, returning them as a cursor. You can iterate over this cursor to fetch multiple records.
Example:
db.users.find({ age: { $gt: 25 } });
This fetches all users older than 25.
The findOne() method, on the other hand, returns only the first document that matches the criteria. It does not return a cursor but a single document.
db.users.findOne({ age: { $gt: 25 } });
This returns only the first user who is older than 25.
Use find() when expecting multiple records and findOne() when a single document is sufficient.
Ques 9: How does MongoDB handle relationships between documents?
Ans.
MongoDB supports two primary ways to manage relationships between documents:
Embedding (Denormalization): Storing related data within the same document.
- Example: A user document embedding address details.
{ "_id": 1, "name": "John Doe", "address": { "street": "123 Main St", "city": "New York" } }
- Suitable when related data is frequently accessed together.
Referencing (Normalization): Storing related data in separate collections and linking them via references.
- Example: A user document storing only an address_id, referring to another collection.
{ "_id": 1, "name": "John Doe", "address_id": 1001 }
- The address is stored separately and can be fetched when needed.
Referencing is useful when data relationships are complex or frequently updated, while embedding is better for high-speed reads with minimal updates.
Ques 10: What is the difference between updateOne(), updateMany(), and replaceOne()?
Ans.
updateOne(filter, update, options): Updates the first matching document based on the filter criteria.
db.users.updateOne({ name: "John" }, { $set: { age: 30 } });
- This updates the first document where name is “John”.
updateMany(filter, update, options): Updates all documents matching the filter criteria.
db.users.updateMany({ city: "New York" }, { $set: { status: "active" } });
- This updates all users in “New York” by setting their status to “active”.
replaceOne(filter, replacement, options): Replaces an entire document with a new document while keeping the _id unchanged.
db.users.replaceOne({ _id: 1 }, { name: "Alice", age: 28, city: "London" });
- This replaces the document with _id: 1 completely.
Ques 11: What is the difference between updateOne(), updateMany(), and replaceOne()?
Ans.
MongoDB provides multiple methods for deleting documents:
deleteOne(filter): Deletes the first document matching the filter.
db.users.deleteOne({ name: "John" });
- Deletes one user named “John”.
deleteMany(filter): Deletes all documents matching the filter.
db.users.deleteMany({ status: "inactive" });
- Deletes all inactive users.
drop(): Deletes an entire collection.
db.users.drop();
- Permanently deletes the users collection and all its documents.
Use deleteOne() when removing a single document, deleteMany() for bulk deletion, and drop() when you no longer need a collection.
Ques 12: How does MongoDB support transactions?
Ans.
MongoDB provides multi-document ACID transactions, ensuring operations are executed reliably across multiple documents. Transactions help maintain data integrity in cases requiring atomic operations, like financial transactions.
To start a transaction:
const session = db.getMongo().startSession(); session.startTransaction(); try { db.accounts.updateOne({ _id: 1 }, { $inc: { balance: -100 } }, { session }); db.accounts.updateOne({ _id: 2 }, { $inc: { balance: 100 } }, { session }); session.commitTransaction(); } catch (error) { session.abortTransaction(); } session.endSession();
- Here, if either update fails, the transaction is aborted, preventing inconsistent data.
Transactions are ideal for applications requiring high consistency, such as banking or e-commerce.
Ques 13: What are capped collections in MongoDB?
Ans.
A capped collection is a fixed-size collection that follows FIFO (First-In-First-Out) data management. Once the size limit is reached, older documents are automatically overwritten.
To create a capped collection:
db.createCollection("logs", { capped: true, size: 1024, max: 100 });
Key features:
- Fixed size: Defined during creation.
- Automatic deletion: Oldest documents are removed when the limit is exceeded.
- High-speed inserts: Optimized for logging and real-time data.
Capped collections are commonly used for logging, caching, and real-time analytics.
Ques 14: What is $lookup, and how is it used in MongoDB?
Ans.
The $lookup stage in MongoDB’s Aggregation Framework performs left outer joins, allowing data from different collections to be merged.
Example: Joining orders with customers:
db.orders.aggregate([ { $lookup: { from: "customers", localField: "customer_id", foreignField: "_id", as: "customer_info" } } ]);
This fetches customer details for each order where customer_id matches _id in the customers collection.
- $lookup is useful for relational-style queries in NoSQL databases.
Ques 15: How do you perform text search in MongoDB?
Ans.
MongoDB provides text indexing for performing efficient searches in string fields.
Steps to enable text search:
1. Create a text index:
db.articles.createIndex({ content: "text" });
2. Search using $text:
db.articles.find({ $text: { $search: "database" } });
- This fetches all articles containing the word “database”.
3. Use score for ranking results:
db.articles.find( { $text: { $search: "database" } }, { score: { $meta: "textScore" } } ).sort({ score: { $meta: "textScore" } });
- Text search is commonly used in search engines and full-text search applications.
Express.js Interview Questions and Answer for Freshers and Experienced (MERN Stack interview questions)
Ques 16: What is Express.js?
Ans.
Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications.
- It simplifies the process of building server-side applications by offering a thin layer of fundamental web application features, without obscuring Node.js features.
- Developers use Express.js to manage routing, handle requests and responses, and integrate with various templating engines, thereby facilitating the creation of single-page, multi-page, and hybrid web applications.
Ques 17: How do you create a simple server using Express.js?
Ans.
Creating a simple server with Express.js involves initializing an Express application and defining routes to handle client requests. Here’s an example:
const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello, World!'); }); app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); });
- In this code, we import Express, create an app instance, define a route for the root URL that sends a “Hello, World!” message, and start the server on port 3000.
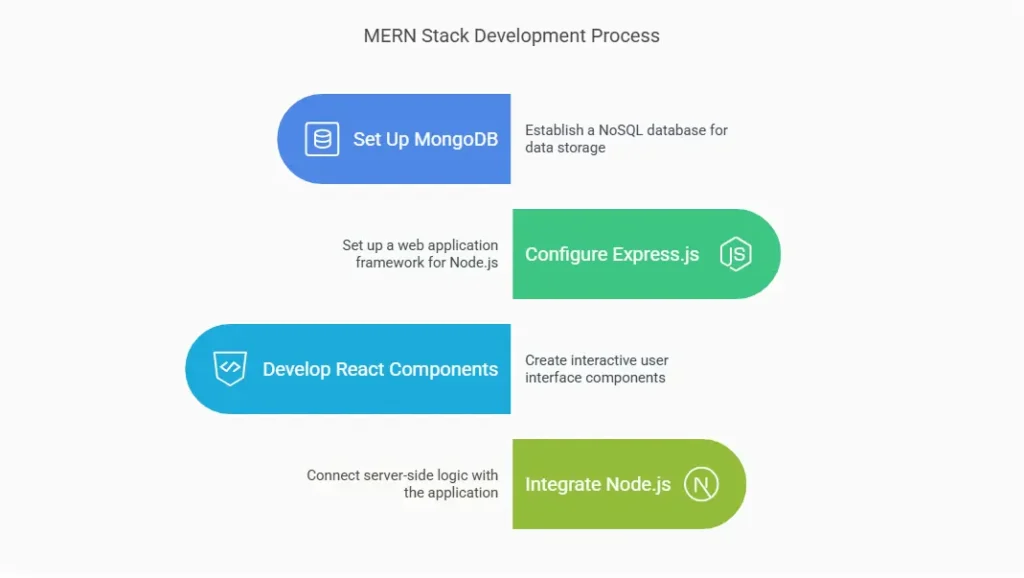
Ques 18: What is middleware in Express.js?
Ans.
Middleware functions in Express.js are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle.
- These functions can execute code, modify the request and response objects, end the request-response cycle, or call the next middleware function.
- Middleware is essential for tasks such as logging, authentication, parsing request bodies, and error handling.
Ques 19: How does routing work in Express.js?
Ans.
Routing in Express.js refers to determining how an application responds to client requests for specific endpoints. Each route can have one or more handler functions, which are executed when the route is matched. Here’s an example of defining routes:
app.get('/users', (req, res) => { res.send('Get request to /users'); }); app.post('/users', (req, res) => { res.send('Post request to /users'); });
- In this example, the first route handles GET requests to /users, and the second handles POST requests to the same path.
Ques 20: What is the role of the next function in Express.js middleware?
Ans.
The next function in Express.js middleware is used to pass control to the next middleware function in the stack.
- If a middleware function does not call next(), the request will be left hanging, and the subsequent middleware functions will not execute.
- This mechanism allows for a sequence of middleware functions to process a request.
Ques 21: How can you serve static files in Express.js?
Ans.
Express.js provides a built-in middleware function, express.static, to serve static files such as images, CSS files, and JavaScript files. Here’s how you can use it:
app.use(express.static('public'));
- In this example, Express will serve files from the public directory. If you have a file named logo.png in the public directory, it can be accessed via http://localhost:3000/logo.png.
Ques 22: How do you handle errors in Express.js?
Ans.
Error handling in Express.js is managed by defining middleware functions that accept four arguments: err, req, res, and next. Here’s an example:
app.use((err, req, res, next) => { console.error(err.stack); res.status(500).send('Something went wrong!'); });
- This middleware function logs the error stack trace and sends a 500 status code with a message. It’s important to define error-handling middleware after all other app.use() and route calls.
Ques 23: What is the difference between app.use() and app.get() in Express.js?
Ans.
In Express.js, app.use() is used to mount middleware functions at a specific path. This middleware will execute for any HTTP method that matches the path. On the other hand, app.get() is used to define a route handler for GET requests to a specific path.
// Middleware for all requests to /api app.use('/api', (req, res, next) => { // Middleware logic next(); }); // Route handler for GET requests to /api/users app.get('/api/users', (req, res) => { res.send('User list'); });
- In this example, the middleware defined with app.use() will execute for any request to paths under /api, while the route handler defined with app.get() will only respond to GET requests to /api/users.
Ques 24: How can you handle different HTTP methods in Express.js?
Ans.
Express.js provides methods corresponding to HTTP methods, such as app.get(), app.post(), app.put(), and app.delete(), to define route handlers for different HTTP requests. Here’s an example:
app.route('/resource') .get((req, res) => { res.send('GET request to /resource'); }) .post((req, res) => { res.send('POST request to /resource'); }) .put((req, res) => { res.send('PUT request to /resource'); }) .delete((req, res) => { res.send('DELETE request to /resource'); });
- This approach allows you to chain multiple handlers for a single route path, each handling a different HTTP method.
Ques 25: What is express.Router() and how is it used?
Ans.
express.Router() is a built-in method in Express.js that creates a new router object.
- This router object can be used to define a set of routes and middleware, which can then be mounted onto the main application.
- This modular approach helps in organizing routes and middleware.
Here’s an example:
const express = require('express'); const router = express.Router(); router.get('/users', (req, res) => { res.send('List of users'); }); router.post('/users', (req, res) => { res.send('User added'); }); module.exports = router;
- In this example, the router handles /users routes separately. To use it in the main application, mount it like this:
const userRoutes = require('./userRoutes'); app.use('/api', userRoutes);
- Now, the routes will be accessible under /api/users.
Ques 26: How do you parse request bodies in Express.js?
Ans.
Express.js does not parse request bodies by default. You need middleware like express.json() and express.urlencoded() to handle JSON and URL-encoded data. Here’s how you can use them:
const express = require('express'); const app = express(); app.use(express.json()); // Parses JSON payloads app.use(express.urlencoded({ extended: true })); // Parses form data app.post('/data', (req, res) => { console.log(req.body); res.send('Data received'); });
- Now, when a client sends a JSON payload in a POST request, Express will parse it and make it available in req.body.
Ques 27: What is CORS, and how do you enable it in Express.js?
Ans.
CORS (Cross-Origin Resource Sharing) is a security feature that restricts resources from being accessed by different origins. By default, browsers block such requests.
You can enable CORS in Express using the cors package:
const cors = require('cors'); app.use(cors());
To allow only specific origins:
app.use(cors({ origin: 'https://example.com' }));
This configuration ensures that only https://example.com can access your API.
Ques 28: How do you implement authentication in Express.js?
Ans.
Authentication in Express can be done using JWT (JSON Web Tokens), session-based authentication, or OAuth. Here’s an example using JWT:
const jwt = require('jsonwebtoken'); app.post('/login', (req, res) => { const user = { id: 1, username: 'user1' }; const token = jwt.sign(user, 'secretkey', { expiresIn: '1h' }); res.json({ token }); }); app.get('/protected', verifyToken, (req, res) => { jwt.verify(req.token, 'secretkey', (err, data) => { if (err) res.sendStatus(403); else res.json({ message: 'Protected data', data }); }); }); function verifyToken(req, res, next) { const bearerHeader = req.headers['authorization']; if (bearerHeader) { req.token = bearerHeader.split(' ')[1]; next(); } else res.sendStatus(403); }
- This example issues a JWT when the user logs in and verifies it for protected routes.
Ques 29: What is the difference between synchronous and asynchronous code in Express.js?
Ans.
Synchronous code executes one statement at a time and blocks the execution of subsequent code until the current operation completes. Asynchronous code, on the other hand, allows non-blocking execution using callbacks, promises, or async/await.
Example of synchronous code:
app.get('/sync', (req, res) => { const data = fs.readFileSync('file.txt', 'utf8'); // Blocks execution res.send(data); });
Example of asynchronous code:
app.get('/async', async (req, res) => { const data = await fs.promises.readFile('file.txt', 'utf8'); // Non-blocking res.send(data); });
Asynchronous code is preferred in Express.js for better performance.
Ques 30: How can you improve the performance of an Express.js application?
Ans.
Here are some best practices to improve performance:
- Use Compression: Enable gzip compression to reduce response size.
const compression = require('compression'); app.use(compression());
- Cache responses: Use caching strategies like Redis for frequently accessed data.
- Use a Reverse Proxy: Tools like Nginx can handle static assets and SSL termination.
- Optimize Database Queries: Avoid unnecessary queries and use indexing.
- Minimize Middleware: Only use required middleware to reduce processing overhead.
- Use Cluster Mode: Utilize Node.js cluster to take advantage of multi-core CPUs.
const cluster = require('cluster'); const os = require('os'); if (cluster.isMaster) { for (let i = 0; i < os.cpus().length; i++) { cluster.fork(); } } else { app.listen(3000); }
Following these practices helps in building a scalable and efficient Express.js application.
React JS Interview Questions and Answer for Freshers and Experienced(MERN Stack interview questions)
Ques 31: What is React JS?
Ans.
React JS is an open-source JavaScript library developed by Facebook for building user interfaces, particularly single-page applications.
- It allows developers to create reusable UI components, leading to efficient and manageable code.
- React’s virtual DOM ensures high performance by updating only the parts of the DOM that have changed, rather than reloading the entire page.
- This approach enhances user experience by making web applications faster and more responsive.
Ques 32: What is JSX in React?
Ans.
JSX, or JavaScript XML, is a syntax extension for JavaScript used in React to describe what the UI should look like.
- It allows developers to write HTML-like code within JavaScript files, making the code more readable and easier to understand.
- Under the hood, JSX is transformed into regular JavaScript objects that React can render.
Example:
const element = <h1>Hello, World!</h1>;
In this example, <h1>Hello, World!</h1> is JSX that represents an h1 element with the text “Hello, World!”.
Ques 33: What are Components in React?
Ans.
Components are the building blocks of a React application. They encapsulate parts of the UI into reusable pieces, each managing its own state and logic. Components can be classified into two types:
- Functional components
- Class components
Functional Components: These are JavaScript functions that accept props as arguments and return React elements.
Example:
function Greeting(props) { return <h1>Hello, {props.name}!</h1>; }
Class Components: These are ES6 classes that extend React.Component and must include a render method returning React elements.
Example:
class Greeting extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; } }
Ques 34: What is the Virtual DOM, and how does it work in React?
Ans.
The Virtual DOM is a lightweight, in-memory representation of the real DOM elements generated by React components.
- When a component’s state or props change, React creates a new Virtual DOM tree and compares it to the previous one in a process called “reconciliation.”
- It then calculates the minimal set of changes required to update the real DOM to match the new Virtual DOM, enhancing performance by reducing direct DOM manipulations.
Ques 35: What are Props in React?
Ans.
Props, short for properties, are read-only attributes passed from parent components to child components.
- They allow data to flow unidirectionally, enabling child components to render dynamic content based on the received data.
- Props are immutable, meaning a component receiving props cannot modify them.
Example :
function Welcome(props) { return <h1>Hello, {props.name}</h1>; } // Usage <Welcome name="Alice" />
- In this example, the Welcome component receives a name prop and renders it accordingly
Ques 36: What is State in React?
Ans.
State is a built-in object that allows components to manage and respond to dynamic data or user input.
- Unlike props, which are immutable and passed down from parent to child, state is local to the component and can be changed using the setState method in class components or the useState hook in functional components.
- When the state changes, React re-renders the component to reflect the new state.
Example using useState in a functional component:
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return ( <div> <p>You clicked {count} times</p> <button onClick={() => setCount(count + 1)}> Click me </button> </div> ); }
- In this example, count is a state variable initialized to 0, and setCount is the function used to update it.
Ques 37: What are Lifecycle Methods in React?
Ans.
Lifecycle methods are special methods in React class components that allow developers to hook into different stages of a component’s lifecycle:
- Mounting: When the component is being inserted into the DOM.
- constructor()
- static
- getDerivedStateFromProps()
- render()
- componentDidMount()
- Updating: When the component is being re-rendered due to changes in props or state.
- static
- getDerivedStateFromProps()
- shouldComponentUpdate()
- render()
- getSnapshotBeforeUpdate()
- componentDidUpdate()
- Unmounting: When the component is being removed from the DOM.
- componentWillUnmount()
These methods provide control over the component’s behavior during its lifecycle.
Ques 38: What are React Hooks?
Ans.
Introduced in React 16.8, Hooks are functions that enable functional components to use state and other React features without writing class components. They promote code reuse and simplify complex component logic.
Commonly used hooks include:
- useState: Manages state in functional components.
- useEffect: Performs side effects in functional components, such as data fetching or subscriptions.
- useContext: Accesses the value of a context directly.
Example using useEffect:
import React, { useState, useEffect } from 'react'; function Timer() { const [seconds, setSeconds] = useState(0); useEffect(() => { const interval = setInterval(() => { setSeconds(prevSeconds => prevSeconds + 1); }, 1000); return () => clearInterval(interval); // Cleanup function to clear interval }, []); return <p>Time elapsed: {seconds} seconds</p>; } export default Timer;
- In this example, useEffect starts a timer that updates every second and clears the interval when the component unmounts.
Ques 39: What is the difference between useEffect and componentDidMount?
Ans.
Both useEffect and componentDidMount are used for handling side effects, but they work differently:
- componentDidMount is a lifecycle method in class components that runs once after the component is mounted.
- useEffect is a Hook used in functional components that can mimic componentDidMount when an empty dependency array [] is passed.
Example:
useEffect(() => { console.log("Component Mounted"); }, []); // Runs once, like componentDidMount
- Unlike componentDidMount, useEffect can also handle updates and unmounting with dependencies.
Ques 40: What is the difference between controlled and uncontrolled components in React?
Ans.
In React, form elements can be either controlled or uncontrolled:
- Controlled Components: The component manages form state using React state. Changes trigger state updates.
- Uncontrolled Components: Form elements maintain their state, and React does not control them.
Controlled Example:
function ControlledInput() {
const [value, setValue] = useState("");
return (
<input value={value} onChange={(e) => setValue(e.target.value)} />
);
}
Uncontrolled Example:
function UncontrolledInput() {
const inputRef = useRef();
return <input ref={inputRef} />;
}
- Controlled components are recommended for better state management.
Ques 41: What is React Context API?
Ans.
The Context API allows data to be shared globally across components without prop drilling.
- It is useful for managing global state, such as themes and authentication.
Example:
const ThemeContext = React.createContext("light"); function App() { return ( <ThemeContext.Provider value="dark"> <ChildComponent /> </ThemeContext.Provider> ); } function ChildComponent() { const theme = useContext(ThemeContext); return <p>Current theme: {theme}</p>; }
- Here, ChildComponent accesses the theme value without receiving props from App.
Ques 42: What are React Fragments?
Ans.
React Fragments (<React.Fragment> or <> </>) allow grouping multiple elements without adding extra nodes to the DOM.
Example:
function Example() { return ( <> <h1>Hello</h1> <p>This is a React Fragment</p> </> ); }
- Using fragments improves performance and avoids unnecessary div elements.
Ques 43: What is the significance of keys in React lists?
Ans.
Keys in React lists help identify elements uniquely, improving rendering efficiency. They prevent unnecessary re-renders by tracking changes efficiently.
Example:
const items = ["Apple", "Banana", "Cherry"]; function List() { return ( <ul> {items.map((item, index) => ( <li key={index}>{item}</li> ))} </ul> ); }
- A unique key (not index, if possible) ensures optimal React performance.
Ques 44: What is React Router?
Ans.
React Router is a library for handling navigation in React applications. It enables dynamic routing without reloading the page.
Example:
import { BrowserRouter as Router, Route, Switch } from "react-router-dom"; function App() { return ( <Router> <Switch> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> </Switch> </Router> ); }
- Here, Switch ensures only one route renders at a time.
Ques 45: What is Redux in React?
Ans.
Redux is a state management library that helps manage global state in React applications. It follows a unidirectional data flow and uses actions, reducers, and stores.
Basic Example:
import { createStore } from "redux"; const reducer = (state = { count: 0 }, action) => { if (action.type === "INCREMENT") return { count: state.count + 1 }; return state; }; const store = createStore(reducer); store.dispatch({ type: "INCREMENT" }); console.log(store.getState()); // { count: 1 }
- Redux is useful for complex applications requiring centralized state management.
Node.js Interview Questions and Answer for Freshers and Experienced
For Node.js Interview Questions and Answers, You can check our dedicated page of Node.js Interview Question
👇
Conclusion
Preparing for a MERN Stack interview questions requires a good understanding of MongoDB, Express.js, React.js, and Node.js. In this blog, we covered important questions that can help you build confidence and improve your knowledge.
To crack your interview, focus on practicing coding problems, understanding core concepts, and building real-world projects. Also, stay updated with the latest trends and best practices in full-stack development.
We hope these MERN Stack interview questions and answers help you in your preparation.
Also Check
Note – We divided Technical interview questions on the basis of Skill, Language and Core Subjects to prepare well for exam.
Join Our Interview Course Now to Get Yourself Prepared -
Join Our Interview Course Now to Get Yourself Prepared
Prepare for the interview process in both Service and Product Based companies along with Group Discussion, Puzzles, Resume Building, HR, MR and Technical Interviews.