Library Function atan in Math Class
Library Function atan of math.h Header File in C
On this page we will discuss about library function atan in math class which is used in C.The C header file math.h contains the standard math library functions that can be used for performing various mathematical operations. The atan function returns the arc tangent of x in radians.
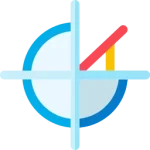
Library Function atan of math.h Header File
In C programming language the atan function is included in math.h header file.
The range of input argument which is passed to atan function is not limited here as it can be any value from negative to positive and returns the inverse tangent of parameter x in radians.
atan(x) = \tan^{-1}(x)
Declaration of atan function
double atan(double x)
Parameters of atan function
The atan function accepts a single input argument which is a double value between -1 and 1.
Parameter | Description |
---|---|
double value | This value of double function ranges from -1 to 1. |
Return value of atan function
The atan function returns a value in radians and it’s type is double. The function return value that lies in the range of \left [ -\Pi/2,\Pi/2 \right ] radians.
Parameter | Return Value |
---|---|
\left [ -\Pi/2,\Pi/2 \right ] | It returns the value in radians. |
Implementation of Library Function math.h atan
Example 1:
The following code shows the use of atan function.
#include<stdio.h> #include<conio.h> #define PI 3.141592654 int main() { /* Define temporary variables */ double a, b, result; /* Assign the two values to variables to find the atan2 of */ b = 4.56; a = -12.5; /* Calculate the Arc Tangent of value1 and value2 */ result = atan2(b, a); /* Converting radians to degrees */ result = result * 180.0/PI; /* Display the result of the calculation */ printf("The arc tangent of %.1lf and %.1lf is %.1lf degrees.", a, b, result); return 0; }
Output:
Inverse of tan(3.00) function = 1.25 in radians Inverse of tan(3.00) function = 71.57 in degrees
Example 2:
#include<stdio.h> #define PI 3.141592654 int main() { double number = 9.0; double return_value; return_value = atan(number); printf("Inverse of tan(%.3f) function = %.3f in radians", number, return_value); // Converting radians to degrees return_value = (return_value * 180) / PI; printf("\nInverse of tan(%.3f) function = %.3f in degrees", number, return_value); return 0; }
Output:
Inverse of tan(9.000) function = 1.460 in radians Inverse of tan(9.000) function = 83.660 in degrees
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others
Login/Signup to comment