Library Function atan2 in Math Class
Library Function atan2 of math.h Header File in C
On this page we will discuss about library function atan2 in math class which is used in C.The C header file math.h contains the standard math library functions that can be used for performing various mathematical operations. The atan2 function returns the arc tangent of \frac{y}{x} in radians.
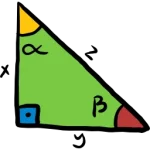
Library Function atan2 in Math class used in C
In C programming language the atan2 function is included in math.h header file.
The range of input arguments which are passed to atan2 function can be either a positive or a negative number and returns the arc tangent of parameter \frac{y}{x} in radians.
atan2(y,x) = \tan^{-1}\frac{y}{x}
Declaration of atan2 function
double atan2(double y, double x);
Parameters of atan2 function
The atan2 function accepts two input arguments of double type as given below:
Parameter | Description |
---|---|
x axis | This parameter is represented by x coordinate at input point of type double. |
y axis | This parameter is represented by y coordinate at input point of type double. |
Return value of atan2 function
The atan2 function returns a value in radians and it’s type is double. The function return value lies in the range of \left [ -\Pi,\Pi \right ] radians.
Parameter | Return Value |
---|---|
x> 0,y> 0\; or\; x<0,y< 0 | It returns the value between -\Pi\; and\; \Pi of type double. |
x=0, y=0 | The function will return a domain error. |
Implementation of Library Function math.h atan2
Example 1:
The following code shows the use of atan2 function.#include <stdio.h> #include <math.h> #define PI 3.141592654 int main() { double number = 3.0; double return_value; return_value = atan(number); printf("Inverse of tan(%.2f) function = %.2f in radians", number, return_value); // Converting radians to degrees return_value = (return_value * 180) / PI; printf("\nInverse of tan(%.2f) function = %.2f in degrees", number, return_value); return 0; }
Output:
The arc tangent of -12.5 and 4.6 is 160.0 degrees.
Example 2:
The following code shows use of atan2 function when inputs are entered by user.#include <stdio.h> #include <math.h> #define PI 3.141592654 int main() { /* Define temporary variables */ double variable1, variable2, result; /* Assign the two values to variables to find the atan2 of */ printf("Enter the value of variable1: "); scanf("%lf", &variable1); printf("Enter the value of variable2: "); scanf("%lf", &variable2); /* Calculate the Arc Tangent of value1 and value2 */ result = atan2(variable2, variable1); /* Converting radians to degrees */ result = result * 180.0/PI; /* Display the result of the calculation */ printf("The arc tangent of %.1lf and %.1lf is %.1lf degrees.", variable1, variable2, result); return 0; }
Output:
Enter the value of variable1: 6.78 Enter the value of variable2: -13.5 The arc tangent of 6.8 and -13.5 is -63.3 degrees.
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others
Login/Signup to comment