Java SortedMap Interface
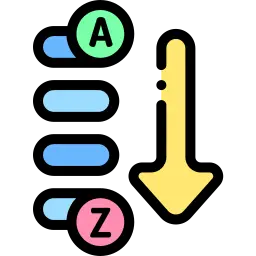
What is Java SortedMap Interface?
Java’s SortedMap is a subtype of Map interface that maintains its keys in ascending order, allowing efficient retrieval of entries based on their key’s natural ordering or a custom comparator.
It provides additional methods like subMap, headMap, tailMap to get a subset of the map, and firstKey, lastKey to get the first and last keys in the map. It’s implemented by TreeMap class in Java.
How to use SortedMap?
- To use a SortedMap in Java, you can follow these steps:
- Import the required packages:
- Create a SortedMap instance:
- Add elements to the sortedMap:
- Retrieve elements from the sortedMap:
- Iterate over the sortedMap:
Methods of SortedMap
comparator(): Returns the comparator used to order the keys in the map. If the natural ordering is used, this method returns null.
firstKey(): Returns the first (lowest) key in the map.
lastKey(): Returns the last (highest) key in the map.
subMap(K fromKey, K toKey): Returns a view of the portion of the map whose keys range from fromKey, inclusive, to toKey, exclusive.
headMap(K toKey): Returns a view of the portion of the map whose keys are strictly less than toKey.
tailMap(K fromKey): Returns a view of the portion of the map whose keys are greater than or equal to fromKey.
comparator(): Returns the comparator used to order the keys in the map.
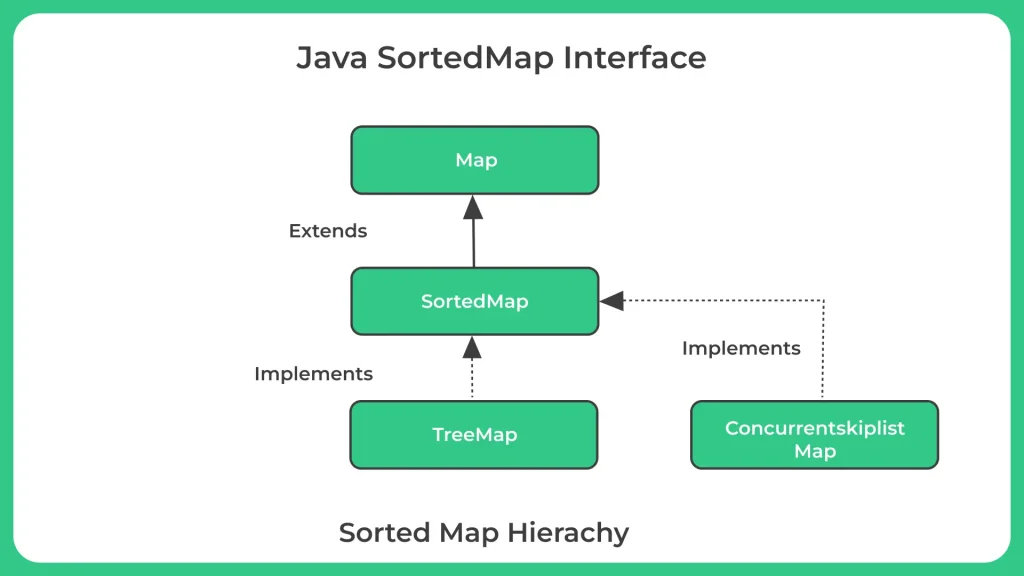
SortedMap Interface
The SortedMap interface provides several methods for working with sorted maps, including methods for retrieving the first and last keys and values, getting a submap of the sorted map, and finding the closest key to a given key.
SortedMap
The TreeMap class is a commonly used implementation of the SortedMap interface in Java. TreeMap sorts its keys in natural order (i.e., according to their natural ordering or the ordering specified by a custom Comparator), and provides efficient performance for most operations.
Example 1: Java program that uses the SortedMap interface:
Run
import java.util.*; public class Main { public static void main(String[] args) { // Creating a SortedMap SortedMapsortedMap = new TreeMap<>(); // Adding elements to the SortedMap sortedMap.put(1, "One"); sortedMap.put(4, "Four"); sortedMap.put(2, "Two"); sortedMap.put(5, "Five"); sortedMap.put(3, "Three"); // Printing the SortedMap System.out.println("SortedMap: " + sortedMap); // Retrieving the first key and value int firstKey = sortedMap.firstKey(); String firstValue = sortedMap.get(firstKey); System.out.println("First Key: " + firstKey + ", First Value: " + firstValue); // Retrieving the last key and value int lastKey = sortedMap.lastKey(); String lastValue = sortedMap.get(lastKey); System.out.println("Last Key: " + lastKey + ", Last Value: " + lastValue); // Retrieving a submap of the SortedMap SortedMap subMap = sortedMap.subMap(2, 5); System.out.println("Submap: " + subMap); // Removing an element from the SortedMap sortedMap.remove(4); System.out.println("SortedMap after removing element with key 4: " + sortedMap); } }
Output
SortedMap: {1=One, 2=Two, 3=Three, 4=Four, 5=Five} First Key: 1, First Value: One Last Key: 5, Last Value: Five Submap: {2=Two, 3=Three, 4=Four} SortedMap after removing element with key 4: {1=One, 2=Two, 3=Three, 5=Five}
Explanation:
This program creates a SortedMap using the TreeMap implementation, adds some elements to it, retrieves the first and last keys and values, gets a submap of the SortedMap, and removes an element from it.
Example 2: Java Program to SortMap By Key Examples
import java.util.*; //SortMapByKeyExample public class Main { public static void main (String[]args) { // Creating an unsorted Map Map < String, Integer > unsortedMap = new HashMap <> (); unsortedMap.put ("Alice", 26); unsortedMap.put ("Charlie", 32); unsortedMap.put ("Bob", 21); unsortedMap.put ("David", 29); unsortedMap.put ("Eve", 24); // Creating a SortedMap from the unsorted Map SortedMap < String, Integer > sortedMap = new TreeMap <> (unsortedMap); // Printing the original and sorted Maps System.out.println ("Unsorted Map: " + unsortedMap); System.out.println ("Sorted Map: " + sortedMap); } }
Output
Unsorted Map: {Bob=21, Eve=24, Alice=26, Charlie=32, David=29} Sorted Map: {Alice=26, Bob=21, Charlie=32, David=29, Eve=24}
Explanation:
This program creates an unsorted Map with String keys and Integer values, then creates a SortedMap from the unsorted Map using the TreeMap implementation. Because TreeMap sorts its keys in natural order (i.e., alphabetical order for String keys), the resulting SortedMap will be sorted by its keys.
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others
Login/Signup to comment