Library Function acosh in Math Class
Library Function acosh of math.h Header File in C
On this page we will discuss about library function acosh in math class which is used in C.The C header file math.h contains the standard math library functions that can be used for performing various mathematical operations. The acosh function returns the arc hyperbolic cosine of x in radians.
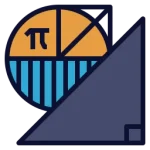
Library Function acosh of math.h Header File
- In C programming language the acosh function is included in math.h header file.
- The acosh function takes input argument in the range of (x≥1) and returns the inverse hyperbolic cosine of parameter x in radians.
acosh(x)=cosh−1(x)
Declaration of acosh function
double acosh(double x)
int x = 0; double result; result = acosh(double(x));
Parameters of acosh function
The acosh function accepts a single input value which is greater than or equal to 1.
Parameter | Description |
---|---|
double value | This value of double function is greater than or equal to 1(x≥1). |
Return value of acosh function
The acosh function returns a value or a number which is greater than or equal to 0 in radians. The function returns NaN if the parameter passed to acosh function is less than 1.
Parameter | Return Value |
---|---|
x≥1 | It returns a number greater than or equal to 0 in radians |
x>1 or x<-1 | NaN (not a number) |
Implementation of Library Function math.h acosh
Example 1:
The following code shows the use of acosh function with different parameters passed to the function.
#include <stdio.h> #include <math.h> int main() { // constant PI is defined const double PI = 3.1415926; double y, return_value_of_function; y = 6.8; return_value_of_function = acosh(y); printf("Value of acosh(%.2f) function = %.2lf in radians\n", y, return_value_of_function); // converting radians to degree return_value_of_function = acosh(y)*180/PI; printf("Value of acosh(%.2f) function = %.2lf in degrees\n", y, return_value_of_function); // parameter not in range y = 0.7; return_value_of_function = acosh(y); printf("Value of acosh(%.2f) function = %.2lf", y, return_value_of_function); return 0; }
Output:
Value of acosh(6.80) function = 2.60 in radians Value of acosh(6.80) function = 149.23 in degrees Value of acosh(0.70) function = -nan
Example 2:
The following code shows the use of acosh function when parameters such as INFINITY and DBL_MAX are passed to the function.
#include <stdio.h> #include <math.h> #include <float.h> int main() { double y, return_value_of_function; // maximum representable finite floating-point number y = DBL_MAX; return_value_of_function = acosh(y); printf("Maximum value of acosh() function in radians = %.3lf\n", return_value_of_function); // Infinity y = INFINITY; return_value_of_function = acosh(y); printf("When infinity is passed to acosh() function, return_value = %.3lf\n", return_value_of_function); return 0; }
Output:
Maximum value of acosh() function in radians = 710.476 When infinity is passed to acosh() function, return_value = inf
Also, math.h header file is included to use INFINITY parameter in our program which is a constant expression representing positive infinity.
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others
Login/Signup to comment