Python Program for Dining Table Seating Arrangement Problem
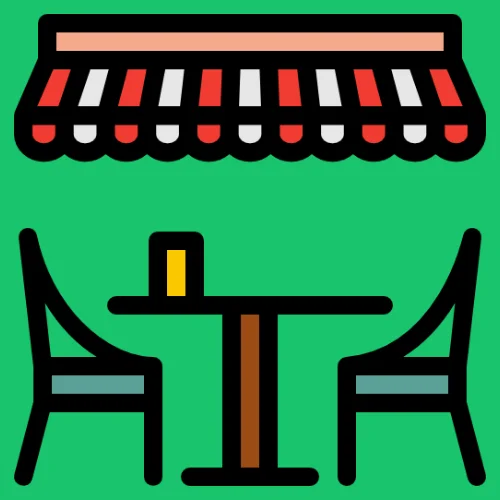
Dining Table Seating Arrangement Problem
One of the real-world problems asked in TCS CodeVita where we need to find the impactful seating arrangements for the N number of attendees. Here is the Python Program for Dining Table Seating Arrangement Problem, which takes user input R for no of Round tables and N for the total number of attendees.
Problem Statement
In a Conference ,attendees are invited for a dinner after the conference.The Co-ordinator,Sagar arranged around round tables for dinner and want to have an impactful seating experience for the attendees.Before finalizing the seating arrangement,he wants to analyze all the possible arrangements.These are R round tables and N attendees.In case where N is an exact multiple of R,the number of attendees must be exactly N//R,,If N is not an exact multiple of R, then the distribution of attendees must be as equal as possible.Please refer to the example section before for better understanding.
For example, R = 2 and N = 3
All possible seating arrangements are
(1,2) & (3)
(1,3) & (2)
(2,3) & (1)
Attendees are numbered from 1 to N.
Input Format:
- The first line contains T denoting the number of test cases.
- Each test case contains two space separated integers R and N, Where R denotes the number of round tables and N denotes the number of attendees.
Output Format:
Single Integer S denoting the number of possible unique arrangements.
Constraints:
- 0 <= R <= 10(Integer)
- 0 < N <= 20 (Integer)
2 5
10
R = 2, N = 5
(1,2,3) & (4,5)
(1,2,4) & (3,5)
(1,2,5) & (3,4)
(1,3,4) & (2,5)
(1,3,5) & (2,4)
(1,4,5) & (2,3)
(2,3,4) & (1,5)
(2,3,5) & (1,4)
(2,4,5) & (1,3)
(3,4,5) & (1,2)
Arrangements like
(1,2,3) & (4,5)
(2,1,3) & (4,5)
(2,3,1) & (4,5) etc.
But as it is a round table,all the above arrangements are same.
testcases = int(input())
for i in range(testcases):
tables, people = map(int, input().split())
if tables >= people:
print(1)
else:
PA = people // tables
PB = PA + 1
TB = people % tables
TA = tables - TB
# Using DP to store factorials pre-hand
fact = [1]
for j in range(1, people + 2):
fact.append(j*fact[j-1])
# Dividing people between tables
divide = fact[people] / (pow(fact[PA], TA) * pow(fact[PB], TB) * fact[TA] * fact[TB])
if PB >= 4:
result = divide * (pow(fact[PA - 1] / 2, TA) * pow((fact[PB - 1] / 2), TB))
else:
result = divide
print(int(result))
Dining Table Seating Arrangement Problem in few other Coding Languages
C
We don’t have the solution for this problem, you can contribute the answer of this code in C programming language, we post that answer on our page
C++
We don’t have the solution for this problem, you can contribute the answer of this code in C++ programming language, we post that answer on our page
Java
We don’t have the solution for this problem, you can contribute the answer of this code in Java programming language, we post that answer on our page
Login/Signup to comment