Plus One Leetcode Solution
Plus One Leetcode Problem :
You are given a large integer represented as an integer array digits, where each digits[i] is the i th digit of the integer. The digits are ordered from most significant to least significant in left-to-right order. The large integer does not contain any leading 0’s.
Increment the large integer by one and return the resulting array of digits.
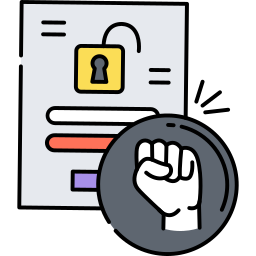
Plus One Leetcode Problem Solution :
Constraints :
- 1 <= digits.length <= 100
- 0 <= digits[i] <= 9
- digits does not contain any leading 0’s.
Example 1:
- Input: digits = [4,3,2,1]
- Output: [4,3,2,2]
- Explanation: The array represents the integer 4321
Incrementing by one gives 4321 + 1 = 4322
Thus, the result should be [4,3,2,2]
Example 2:
- Input: digits = [9]
- Output: [1,0]
- Explanation: The array represents the integer 9.
Incrementing by one gives 9 + 1 = 10.
Thus, the result should be [1,0].
Idea:
Simple Brute Force Approach.
Approach:
Here, we are describing a process where we increment the last digit of an array outside of a loop, and then, within the loop, we have to check if any digit becomes 10.
If a digit becomes 10, you set it to 0 and increment the preceding digit by 1.
This process continues until there are no more 10s. Additionally, if the array has a single-digit number like 9, then add a 0 at the end and change it to 10.
Here’s the explanation for this process:
- Start with an array of digits.
- Increment the last digit by 1 outside of the loop.
- Enter a loop to handle carrying over when a digit becomes 10.
- Inside the loop, check if the current digit is 10.
- If it’s 10, set it to 0 and increment the preceding digit by 1.
- Continue this process until there are no more 10s.
- If the first digit becomes 10, add a new leading 1 to the array.
Finally, you have your modified array.
Complexity:
-
Time Complexity:
Time complexity is O(n). -
Space Complexity:
Space complexity is O(1).
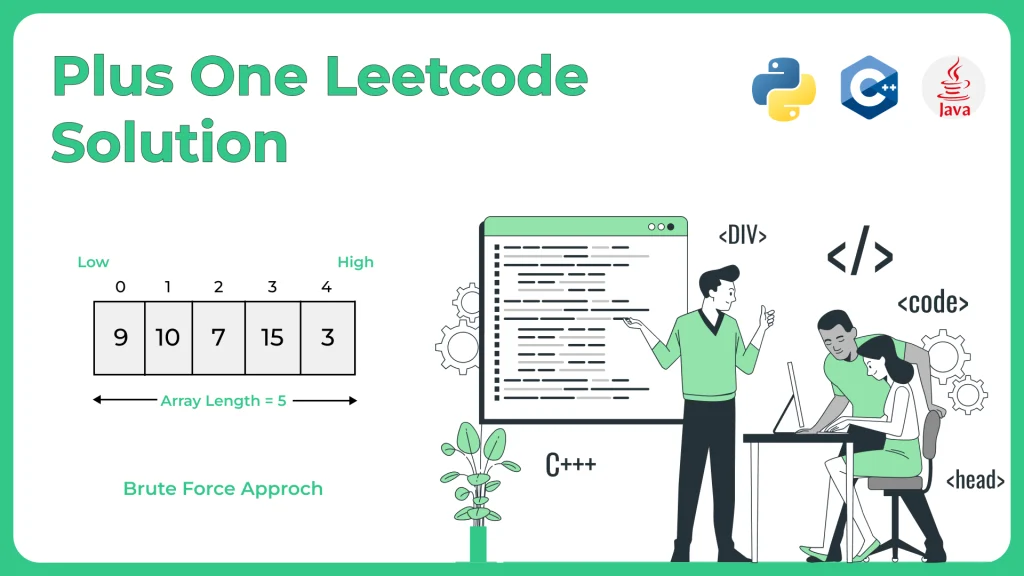
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Code :
class Solution { public: vectorplusOne(vector< int>& digits) { int n=digits.size(); int sum=0; digits[n-1]++; for(int i=n-1;i>=0;i--){ if(digits[i]==10){ digits[i]=0; if(i-1>=0) { digits[i-1]++; } else{ digits.push_back(0); digits[i]++; } } } return digits; } };
import java.util.ArrayList; import java.util.List; class Solution { public List< Integer> plusOne(List< Integer> digits) { int n = digits.size(); int sum = 0; digits.set(n-1, digits.get(n-1) + 1); for (int i = n-1; i >= 0; i--) { if (digits.get(i) == 10) { digits.set(i, 0); if (i-1 >= 0) { digits.set(i-1, digits.get(i-1) + 1); } else { digits.add(0); digits.set(i, digits.get(i) + 1); } } } return digits; } }
class Solution: def plusOne(self, digits): n = len(digits) digits[n-1] += 1 for i in range(n-1, -1, -1): if digits[i] == 10: digits[i] = 0 if i-1 >= 0: digits[i-1] += 1 else: digits.append(0) digits[i] += 1 return digits
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others
Login/Signup to comment