Diameter of Binary Tree Leetcode Solution
Diameter of Binary Tree Leetcode Problem :
Given the root of a binary tree, return the length of the diameter of the tree. The diameter of a binary tree is the length of the longest path between any two nodes in a tree. This path may or may not pass through the root. The length of a path between two nodes is represented by the number of edges between them.
Example :
Input: root = [1,2,3,4,5]
Output: 3
Explanation: 3 is the length of the path [4,2,1,3] or [5,2,1,3].
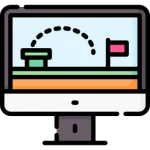
Diameter of Binary Tree Leetcode Solution :
Constraints :
- The number of nodes in the tree is in the range [1, 104].
- -100 <= Node.val <= 100
Example 1:
- Input: root = [1,2]
- Output: 1
Intuition :
- Traverse the tree using a depth-first search (DFS) or breadth-first search (BFS) algorithm.
- For each node, calculate the length of the longest path that passes through that node. This length is equal to the height of the left subtree of the node plus the height of the right subtree of the node, plus one (to count the node itself).
- The diameter of the tree is equal to the maximum of all of these lengths.
Approach :
- Start traversing the tree recursively and do work in Post Order.
In the Post Order of every node , calculate diameter and height of the current node. - If current diameter is maximum then update the variable used to store the maximum diameter.
- Return height of current node to the previous recursive call.
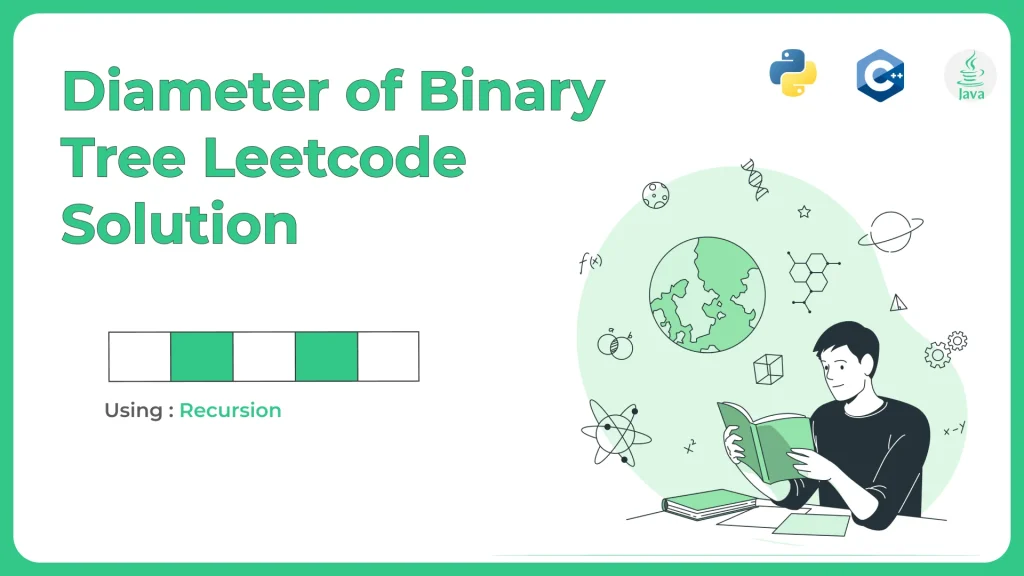
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Code :
C++
Java
Python
C++
class Solution { public: int diameterOfBinaryTree(TreeNode* root) { int diameter = 0; height(root,diameter); return diameter; } int height(TreeNode* node, int& diameter){ if(!node) return 0; int lh = height(node->left,diameter); int rh = height(node->right,diameter); diameter = max(diameter,lh+rh); return 1 + max(lh,rh); } };
Java
class Solution { public int diameterOfBinaryTree(TreeNode root) { int dia[] = new int[1]; ht(root,dia); return dia[0]; } public int ht(TreeNode root , int dia[]){ if(root == null) return 0; int lh = ht(root.left,dia); int rh = ht(root.right,dia); dia[0] = Math.max(dia[0],lh+rh); return 1 + Math.max(lh,rh); } }
Python
class Solution(object): def diameterOfBinaryTree(self, root): """ :type root: TreeNode :rtype: int """ if root is None: return 0 left_height=self.height(root.left) right_height=self.height(root.right) left_diameter=self.diameterOfBinaryTree(root.left) right_diameter=self.diameterOfBinaryTree(root.right) return max((left_height+right_height), max(left_diameter,right_diameter)) def height(self,root): if root is None: return 0 else: left_height=self.height(root.left) right_height=self.height(root.right) return 1 + max(left_height, right_height)
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others
Login/Signup to comment