Java Program to Generate Multiplication Table
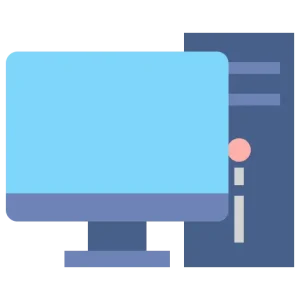
Multiplication Table
In this Article, we will write a program to generate multiplication table of the given number in Java.
This will be done using for loops and while loops.
Program to Generate Multiplication Table Using For Loop:
Run
import java.util.*; public class Main{ public static void main(String[] args) { // Scanner class for taking input Scanner scn = new Scanner(System.in); int num = scn.nextInt(); // Loop to print the table for(int i = 1; i <= 10; i++){ // Printing the table System.out.println(num + " * " + i + " = " + num * i); } } }
Output: 5 * 1 = 5 5 * 2 = 10 5 * 3 = 15 5 * 4 = 20 5 * 5 = 25 5 * 6 = 30 5 * 7 = 35 5 * 8 = 40 5 * 9 = 45 5 * 10 = 50
Explanation :
- The program starts by creating an instance of the Scanner class to take user input.
- It prompts the user to enter an integer, which is then stored in the variable num.
- A for loop is used to iterate from i = 1 to i = 10, representing the numbers in the multiplication table.
- Inside the loop, the multiplication table is printed using the System.out.println statement.
- The statement concatenates the values of num, i, and num * i to form the output string.
a. num represents the input number provided by the user.
b. i represents the current iteration value, ranging from 1 to 10.
c. num * i calculates the result of multiplying num with i. - The output displays the equation num * i = num * i for each iteration of the loop, showing the multiplication table.
- After the loop finishes executing, the program ends.
- The output is the multiplication table for the given input number, ranging from 1 to 10.
Explanation:
In the above Example, We are taking an input of a number for which the multiplication table have to be print. For loop is used for printing the multiplication table.
Program to Create Multiplication Table Using While Loop:
Run
import java.util.*; public class Main{ public static void main(String[] args) { // Scanner class for taking input Scanner scn = new Scanner(System.in); int num = scn.nextInt(); int i = 1; // Loop to print the table while(i <= 10){ // Printing the table System.out.println(num + " * " + i + " = " + num * i); i++; } } }
Output: 5 * 1 = 5 5 * 2 = 10 5 * 3 = 15 5 * 4 = 20 5 * 5 = 25 5 * 6 = 30 5 * 7 = 35 5 * 8 = 40 5 * 9 = 45 5 * 10 = 50
Explanation:
In the above Example, We are taking an input of a number for which the multiplication table have to be print. While loop is used for printing the multiplication table.
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others
Login/Signup to comment