Postorder Traversal of Binary Tree
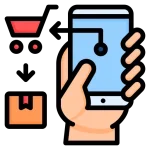
Introduction to Postorder Traversal of Binary Traversal
Postorder traversal of Binary Tree is particularly useful in scenarios where you need to perform operations or collect information about the descendants of a node before processing the node itself. Some common applications of postorder traversal include expression evaluation, binary tree deletion, memory management, and dependency resolution in software systems.
Let’s learn more about Postorder Traversal of Binary Tree along with its working and implementation.
Postorder Traversal of Binary Tree
Postorder traversal is a method used to explore and traverse a binary tree data structure. A binary tree is a hierarchical structure consisting of nodes, where each node can have, at most, two child nodes.
Postorder Traversal Example
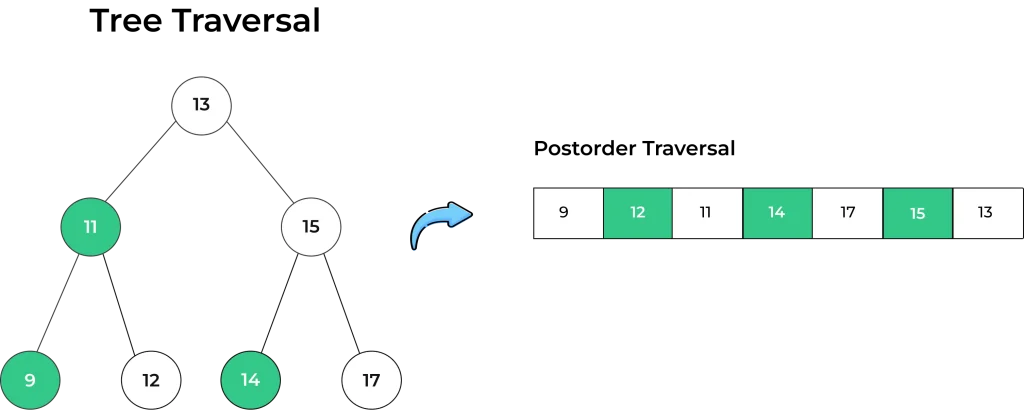
Visualization of Postorder Traversal of Binary Tree
In postorder traversal, you visit the nodes in a specific order:
- Traverse the left subtree in a postorder fashion.
- Traverse the right subtree in a postorder fashion.
- Visit the root node.
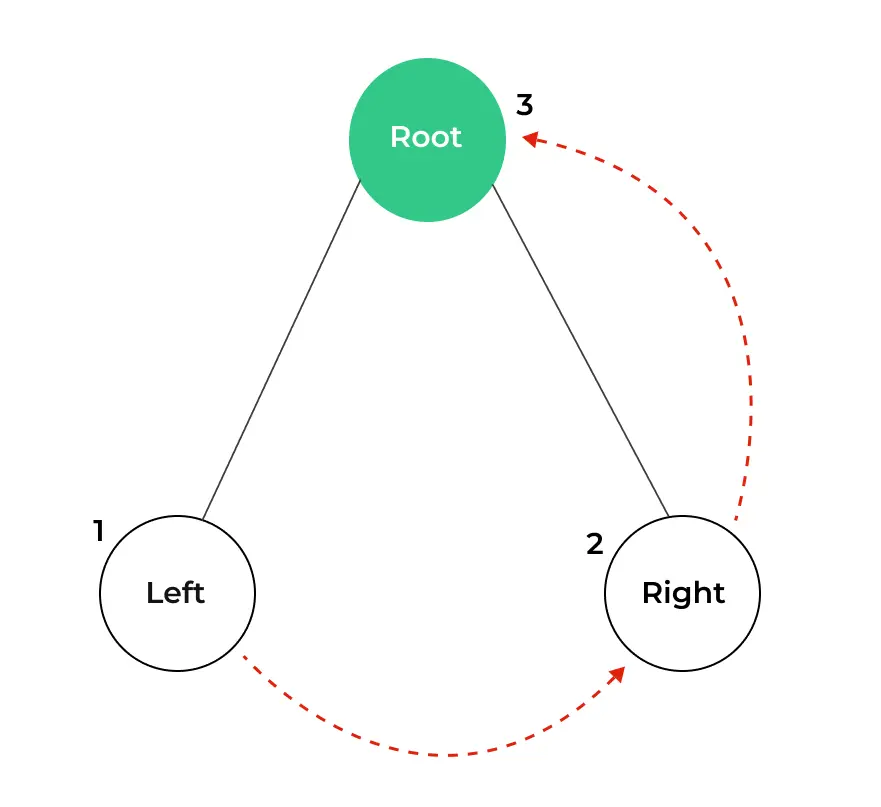
Working of Postorder Traversal of Binary Tree
Consider a binary tree :
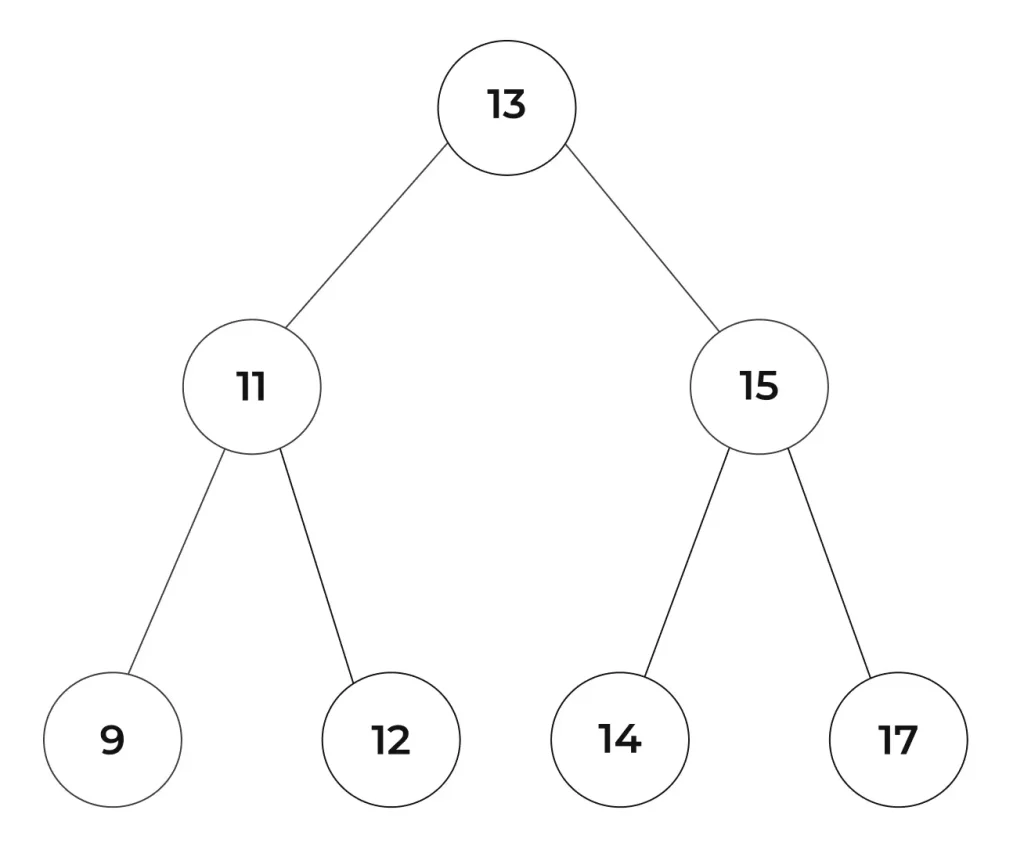
Performing a postorder traversal on this binary tree involves the following steps:
Step 1: Begin from the leftmost subtree.
Step 2: Traverse the right subtree of the root node 11 i.e. node 12.
Step 3: Now both left and right subtree are traversed. Now let’s visit root node i.e. node 11.
Step 4: Repeat these steps to get the final result.
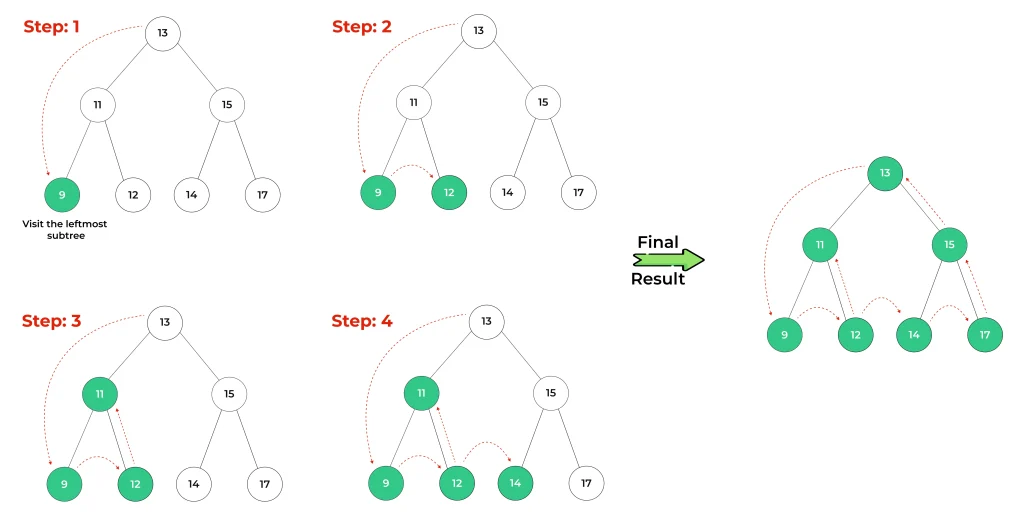
Postorder Traversal using Recursive Method
Code :
class TreeNode: def __init__(self, value): self.value = value self.left = None self.right = None def postorder_traversal(node): if node: # Traverse the left subtree in a postorder fashion postorder_traversal(node.left) # Traverse the right subtree in a postorder fashion postorder_traversal(node.right) # Visit (process) the current node print(node.value, end=" ") # Print the node value, separated by a space # Create a binary tree with different numbers and perform a postorder traversal root = TreeNode(10) root.left = TreeNode(5) root.right = TreeNode(15) root.left.left = TreeNode(3) root.left.right = TreeNode(7) root.right.left = TreeNode(12) root.right.right = TreeNode(18) print("Postorder Traversal:") postorder_traversal(root)
Output :
Postorder Traversal: 3 7 5 12 18 15 10
Explanation :
The code performs a postorder traversal on a binary tree with different numbers. Postorder traversal starts with the left subtree, then the right subtree, and finally the root. The output, “3 7 5 12 18 15 10,” represents the order in which the tree’s nodes are visited. Starting from the lowest values in the left subtree (3 and 7), it gradually moves to the right subtree (12 and 18) and ultimately the root node (10).
Postorder Traversal using Iterative Method(Using Stack)
class TreeNode: def __init__(self, value): self.value = value self.left = None self.right = None def postorder_iterative(root): if not root: return [] result = [] stack = [] current = root while stack or current: if current: if current.right: stack.append(current.right) stack.append(current) current = current.left else: node = stack.pop() if node.right and stack and node.right == stack[-1]: stack.pop() stack.append(node) current = node.right else: result.append(node.value) return result # Create a binary tree with different numbers and perform an iterative postorder traversal root = TreeNode(10) root.left = TreeNode(5) root.right = TreeNode(15) root.left.left = TreeNode(3) root.left.right = TreeNode(7) root.right.left = TreeNode(12) root.right.right = TreeNode(18) print("Postorder Traversal:") result = postorder_iterative(root) print(result)
Output :
Postorder Traversal: [3, 7, 5, 12, 18, 15, 10]
Explanation :
The provided code performs an iterative postorder traversal on a binary tree with various numbers. Postorder traversal visits the left subtree, then the right subtree, and finally the root. The output, “[3, 7, 5, 12, 18, 15, 10],” signifies the order in which the tree’s nodes are visited. It starts with the smallest values in the left subtree (3 and 7), proceeds to the right subtree (12 and 18), and concludes at the root node (10).
Complexity Analysis of Postorder Traversal of Binary Tree
Applications of Tree Traversal in Data Structure
Tree traversal algorithms have a wide range of applications in computer science and real-world scenarios. Some common applications include:
To Wrap it up:
In conclusion, postorder traversal is a fundamental technique for exploring the nodes of a binary tree. By visiting the left and right subtrees before the root, it offers a unique perspective on the tree’s structure. Whether through a simple recursive approach or a memory-efficient iterative method, postorder traversal finds applications in diverse areas, from expression evaluation to memory management.
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
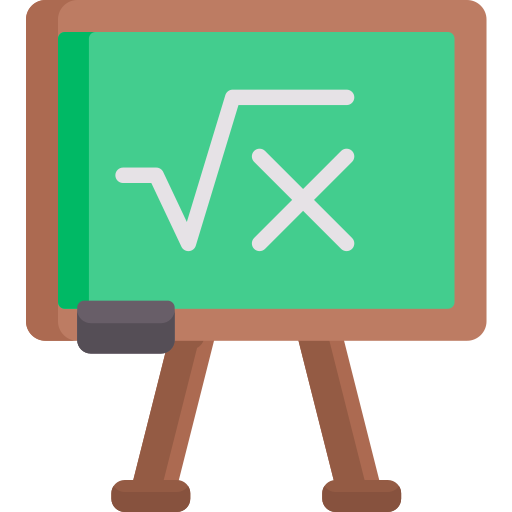
Question 1.
How does the time complexity of postorder traversal compare to other traversal methods?
The time complexity of postorder traversal is the same as preorder and inorder, O(n), where ‘n’ is the number of nodes in the tree.
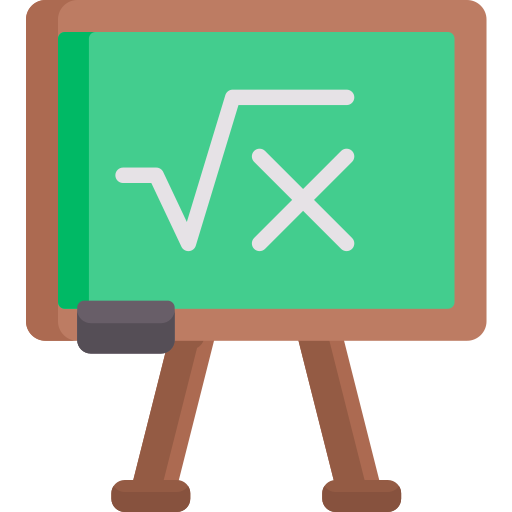
Question 2.
What is the difference between recursive and iterative methods for postorder traversal in terms of efficiency and memory usage?
The recursive method is simpler but may have higher space complexity due to the call stack. The iterative method can be more memory-efficient, especially for deep or skewed trees.
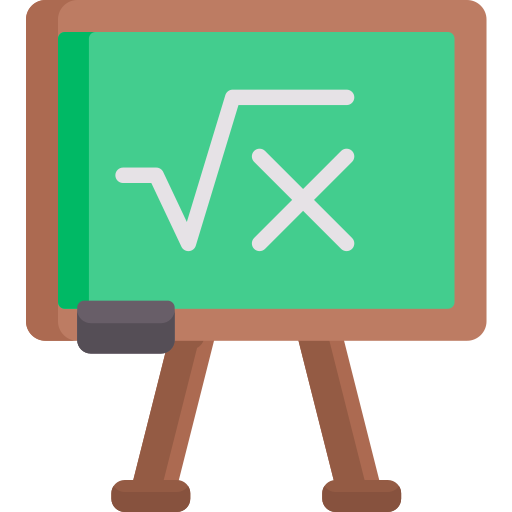
Question 3.
When should I choose postorder traversal over other traversal methods?
Use postorder traversal when you need to process a node’s descendants before the node itself. It’s suitable for scenarios like binary tree deletion, expression evaluation, or memory management.
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others