Merge K sorted list in Python
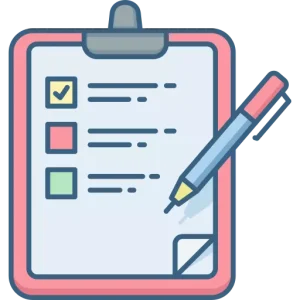
Understanding Merge K sorted list
Understanding Merge K sorted list in Python lists refers to comprehending the concept of lists that are nearly sorted or k-sorted. A Merge K sorted list in Python is an array or sequence of elements where each element is at most k positions away from its sorted position when the list is fully sorted.
Merge K sorted list in Python
A heap is a specialized tree-based data structure that satisfies the heap property. It is particularly useful for efficiently finding and extracting the maximum (or minimum) element in a collection of elements.
Here are the key points in an introduction to the heap data structure:
Heap Property:
In a max-heap, for every node i other than the root, the value of i is less than or equal to the values of its children.
In a min-heap, for every node i other than the root, the value of i is greater than or equal to the values of its children.
Types of Heaps:
Max-Heap: The root node contains the maximum element, and the values decrease as you move down the tree.
Min-Heap: The root node contains the minimum element, and the values increase as you move down the tree.
Basics of Merge K sorted list in Python
Merging k sorted lists in python is a common problem in computer science and is often encountered in the context of algorithms and data structures. The problem involves combining k sorted lists into a single sorted list. Here are the basics of merging k sorted lists:
Problem Statement:
- Given k sorted lists, the task is to merge them into a single sorted list.
Input:
- The input consists of k sorted lists, each containing elements in ascending order.
Output:
- The output should be a single sorted list containing all the elements from the input lists.
Approach:
- One of the common approaches to solve this problem is to use a min-heap or priority queue.
- Initialize the min-heap with the first element from each of the k lists.
- Pop the smallest element from the heap and add it to the result list.
- Replace the popped element in the heap with the next element from its respective list.
- Repeat the process until all elements are processed.
Algorithm Steps:
- Create a min-heap and initialize it with the first element from each of the k sorted lists.
- While the heap is not empty:
- Pop the smallest element from the heap and add it to the result list.
- Replace the popped element in the heap with the next element from its respective list.
- If the list is empty, continue with the next iteration.
- Return the merged sorted list.
Time Complexity:
- The time complexity of this approach is typically O(N log k), where N is the total number of elements across all k lists.
Implementation:
- The implementation involves using a data structure such as a priority queue or a heap to efficiently select the smallest element from the current set of elements.
Example:
- Suppose you have k sorted lists:
- List 1: [1, 4, 5]
- List 2: [1, 3, 6]
- List 3: [2, 7]
- The merged sorted list would be: [1, 1, 2, 3, 4, 5, 6, 7]
- Suppose you have k sorted lists:
import heapq def merge_k_sorted_lists(lists): # Create a min-heap to store elements along with their list index and element value min_heap = [] # Initialize the heap with the first element from each list for i, lst in enumerate(lists): if lst: # Check if the list is not empty heapq.heappush(min_heap, (lst[0], i, 0)) # (element, list_index, element_index) merged_list = [] # Continue until the min-heap is not empty while min_heap: # Pop the smallest element from the heap val, list_index, element_index = heapq.heappop(min_heap) # Add the smallest element to the merged list merged_list.append(val) # Move to the next element in the same list if element_index + 1 < len(lists[list_index]): next_element = lists[list_index][element_index + 1] heapq.heappush(min_heap, (next_element, list_index, element_index + 1)) return merged_list # Example usage: lists = [ [1, 4, 5], [1, 3, 6], [2, 7] ] result = merge_k_sorted_lists(lists) print(result)
Implementing Efficient Code of Merge K sorted list in Python (^)
Let’s implement an efficient solution to merge k sorted lists in Python using a min-heap. We’ll use the heapq module for heap operations:
import heapq def mergeKLists(lists): merged_list = [] heap = [] # Populate the heap with elements from each list for lst in lists: for val in lst: heapq.heappush(heap, val) # Construct the merged list by extracting elements from the heap while heap: merged_list.append(heapq.heappop(heap)) return merged_list
Applications of Merge K sorted list in Python
Database Merging:
- In database management systems, merging sorted lists is often required when performing operations like merging the results of multiple sorted queries or merging data from different sources.
External Sorting:
- In situations where the data to be sorted doesn’t fit into memory, external sorting techniques utilize merging k sorted chunks of data to efficiently achieve the overall sorted order.
Inverted Index Construction in Information Retrieval:
- When building inverted indexes for information retrieval systems, merging postings lists (sorted lists of document IDs containing a particular term) is a common operation.
Merge Sort Algorithm:
- The merge step in the merge sort algorithm involves merging two sorted lists. Extending this concept to k sorted lists leads to an efficient sorting algorithm known as k-way merge sort.
Online Streaming Algorithms:
- In scenarios where data is continuously streaming in, such as in financial markets or network monitoring, merging k sorted lists can be used to maintain a consolidated sorted view of the incoming data.
Task Scheduling:
- In task scheduling algorithms, merging k sorted lists can represent the scheduling of tasks with different priorities or deadlines.
Graph Algorithms:
- In algorithms like Dijkstra’s shortest path algorithm or Prim’s minimum spanning tree algorithm, merging sorted lists of edges or vertices is a common step in the process.
7. Advantages of Merge K sorted list in Python
To wrap it up:
Efficiently merging K sorted lists in Python is essential in various data handling scenarios. Understanding the available techniques, like priority queues, divide and conquer strategies, and leveraging built-in functions, empowers developers to tackle this task proficiently. Python’s versatility in handling data manipulation tasks makes it a go-to language for such operations, providing multiple pathways to accomplish merging K sorted lists effortlessly.
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
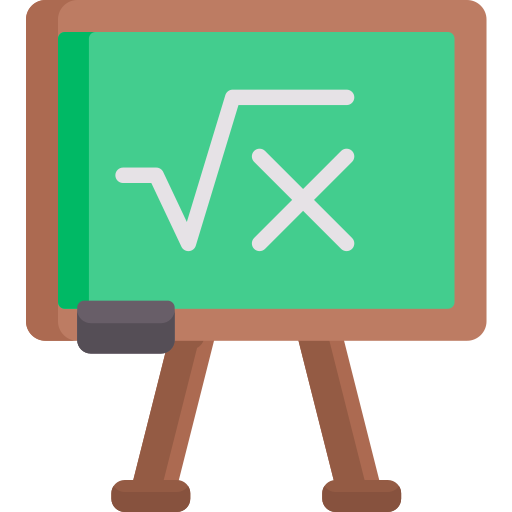
Question 1.
How does a heap assist in merging K sorted lists?
The heap helps in selecting the smallest element among K lists, ensuring efficient merging by maintaining the smallest element at the root.
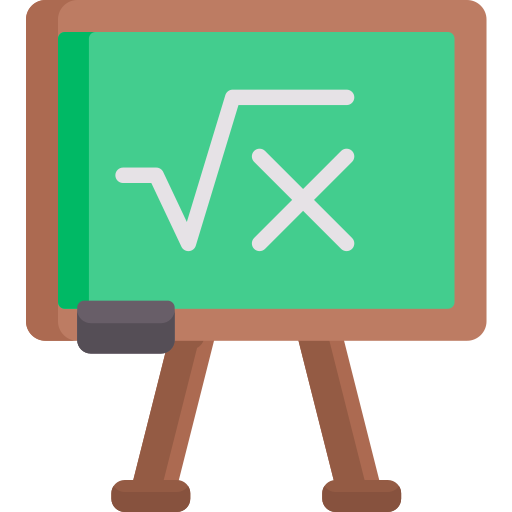
Question 2.
Can I merge more than K lists using this technique?
Absolutely! The method can merge any number of sorted lists, not just limited to K lists.
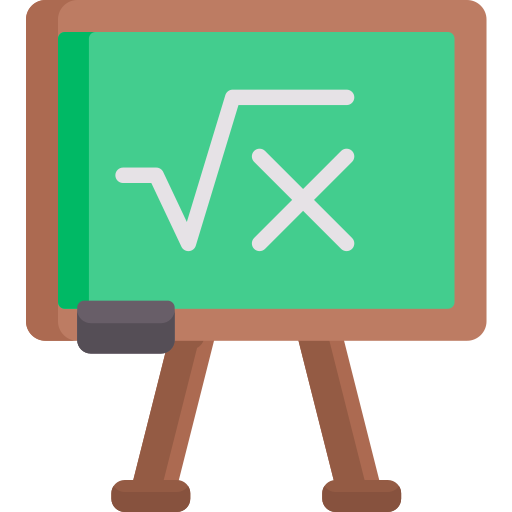
Question 3.
Is the heap-based approach more efficient than the conventional iterative merging method?
Yes, using heaps can significantly improve efficiency, especially when dealing with a large number of lists.
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others