Swap two variables using function in Python
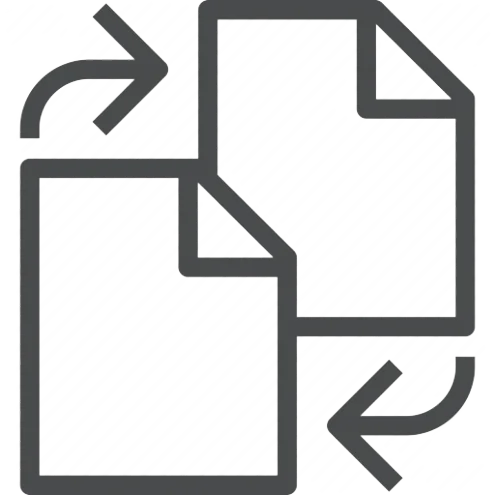
Swap two variables using functions
Swap of two variables using function in Python can be done by passing our variables to the user defined function as arguments and returning or printing the swapped data.
Regarding arguments and returning values , functions are 4 types
- With arguments and return value
- With arguments and without return value
- Without arguments and with return value
- Without arguments and without return value
Working
- Step 1: define a function swap
- Step 2: read two input variables
- Step 3: As per the type of function , pass argument and return the swapped value
- Step 4:Print swapped value
Python code: Swap two variables using function in Python
With arguments and return value:
def swap(x,y):
print(“Before swapping a :”,x)
print(“Before swapping b :”,y)
#logic to swap without using third variable
x,y=y,x
return x,y
a=int(input(“Enter value : “))
b=int(input(“Enter value : “))
a,b=swap(a,b)
print(“After swapping a becomes :”,a)
print(“After swapping b becomes :”,b)
With arguments and without return value:
def swap(x,y):
print(“Before swapping a :”,x)
print(“Before swapping b :”,y)
#logic to swap without using third variable
x,y=y,x
print(“After swapping a becomes :”,x)
print(“After swapping b becomes :”,y)
a=int(input(“Enter value : “))
b=int(input(“Enter value : “))
swap(a,b)
Without arguments and with return value:
def swap():
x=int(input(“Enter value : “))
y=int(input(“Enter value : “))
print(“Before swapping a :”,x)
print(“Before swapping b :”,y)
#logic to swap without using third variable
x,y=y,x
return x,y
a,b=swap()
print(“After swapping a becomes :”,a)
print(“After swapping b becomes :”,b)
Without arguments and without return value:
def swap():
x=int(input(“Enter value : “))
y=int(input(“Enter value : “))
print(“Before swapping a :”,x)
print(“Before swapping b :”,y)
#logic to swap without using third variable
x,y=y,x
print(“After swapping a becomes :”,x)
print(“After swapping b becomes :”,y)
swap()
Enter value : 123
Enter value : 456
Before swapping a : 123
Before swapping b : 456
After swapping a becomes : 456
After swapping b becomes : 123
Login/Signup to comment