Java Program to Find Factorial of a Number Using Recursion
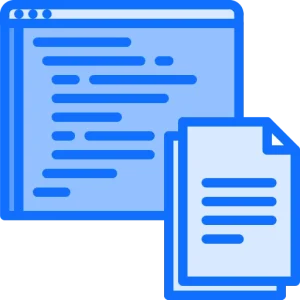
What is Recursion?
Recursion is a Process in which a Function can call itself directly and sometimes indirectly. The Function calling itself, again and again, is known as the Recursive Function.
We can solve certain complex problems quite easily using recursive algorithms like inorder and preorder traversals and many more.
In this Article, we will write a program to Find Factorial of a Number Using Recursion.
Recursion in Java :
Recursion in Java is a programming technique where a method calls itself repeatedly until a termination condition is met. The termination condition is necessary to avoid an infinite loop. In Java, recursion is often used to solve problems that can be divided into smaller sub-problems, such as computing the factorial of a number, searching an element in an array, or traversing a tree data structure. To implement recursion in Java, you need to define the base case and the recursive case. The base case provides the stopping condition, while the recursive case defines the logic for breaking down the problem into smaller sub-problems.
What is Factorial of a Number?
The factorial of a number is the product of all positive integers less than or equal to that number. The factorial function can be calculated using a loop or recursion. In a loop, the factorial is calculated by repeatedly multiplying the current value by the next decreasing positive integer until 1 is reached. In recursion, the factorial is calculated by defining a function that calls itself with the next smaller positive integer until it reaches the base case (1). The final result is the product of all intermediate results.
Example :
The factorial of 5 (written as 5!) is 5 x 4 x 3 x 2 x 1 = 120. The factorial of 0 (0!) is defined as 1.
Program to calculate Factorial of a Number using Loops :
// Importing the required packages import java.util.Scanner; import java.util.*; public class Main{ public static void main(String[] args){ // scanner class for taking input values Scanner sc = new Scanner(System.in); System.out.print("Enter a number: "); int n = sc.nextInt(); // Initializing the variable for storing answer int factorial = 1; for (int i = 1; i <= n; i++) { // calculating factorial using loop factorial *= i; } // Printing the final factorial System.out.println("The factorial of " + n + " is " + factorial); } }
Output: Enter a number: 5 The factorial of 5 is 120
Explanation :
The above program takes an integer input from the user and calculates its factorial using a for loop. The variable factorial is initialized to 1 and is multiplied by each positive integer less than or equal to n in each iteration of the loop. The final value of factorial is the result.
Program to calculate Factorial of a Number using Recursion :
// Importing the required packages import java.util.Scanner; import java.util.*; public class Main{ public static void main(String[] args){ // Scanner class for taking input value Scanner sc = new Scanner(System.in); System.out.print("Enter a number: "); int n = sc.nextInt(); // Calling the factorial function int result = factorial(n); System.out.println("The factorial of " + n + " is " + result); } public static int factorial(int n){ // Base case for recursion if (n == 0) { return 1; } // Returning the Factorial return n * factorial(n - 1); } }
Output: Enter a number: 5 The factorial of 5 is 120
Explanation:
This program takes an integer input from the user and calculates its factorial using a recursive function factorial(int n). The function has a base case if (n == 0), which returns 1, and a recursive case return n * factorial(n – 1), which calls itself with the next smaller positive integer until the base case is reached. The final result is the product of all intermediate results, which is returned to the caller.
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others
Login/Signup to comment