Member Functions in C++
Member Functions
On this page we will discuss about member function in C++ . A member function of a class is a function that has its definition or its prototype within the class definition like any other variable
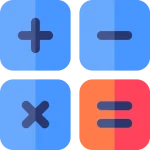
Member Functions in C++
It operates on an object of the class of which it is a member, and has access to all the members of a class for that object.
Lets have a look at member functions below –
Member Function inside the class
Lets look at an example below
class Cube { public: // member variables double len; double breadth; double height; // member function declared and defined // inside class double getVolume(){ return len * breadth * height; } };
Example:-
#include <iostream> using namespace std; class Cube { public: // member variables double len; double breadth; double height; // member function declared and defined // inside class double getVolume(){ return len * breadth * height; } // setters : to set values declared and defined // inside class void setLen(int l){ len = l; } void setBreadth(int b){ breadth = b; } void setHeight(int h){ height = h; } }; int main(){ Cube cube_obj1; cube_obj1.setLen(10.0); cube_obj1.setBreadth(10.0); cube_obj1.setHeight(10.0); cout << "The Volume : " << cube_obj1.getVolume(); }
Output
The Volume : 1000
// member function definition inside the class #include <iostream> using namespace std; class Student//class definition { // member variables int id; string name; public: // member function declared and defined // inside class void displayStudent() { cout << "Name: " << name << endl; cout << "ID: " << id << endl; } void setDetails(int studID, string studName){ id = studID; name = studName; } }; int main() { Student s1; s1.setDetails(1, "Naman"); s1.displayStudent(); }
Output
Name: Naman ID: 1
Problem with function definition inside:
If a function defined inside the class, it becomes inline and implicit expansion takes place, hence performance decreases and recommended to define the function outside the class.
Member Function outside the class
Member functions are defined outside the class using scope resolution operator(::) as shown below –
return_Data_type class_name::function_name()
Let’s look at an example below
class Cube { public: // member variables double len; double breadth; double height; // member function declared inside class // but defined outside double getVolume(); }; // Member function definitioned outside // scope resolutor :: is used double Cube::getVolume() { return length * breadth * height; }
Example:-
#include <iostream> using namespace std; class Cube { public: // member variables double len; double breadth; double height; // member function declared inside // but defined outside double getVolume(); void setLen(int l); void setBreadth(int b); void setHeight(int h); }; double Cube::getVolume(){ return len * breadth * height; } // all class's members functions // defined outside the class with scope resolutor :: void Cube::setLen(int l){ len = l; } void Cube::setBreadth(int b){ breadth = b; } void Cube::setHeight(int h){ height = h; } int main(){ Cube cube_obj1; cube_obj1.setLen(10.0); cube_obj1.setBreadth(10.0); cube_obj1.setHeight(10.0); cout << "The Volume : " << cube_obj1.getVolume(); }
Output
The Volume : 1000
// member function definition inside the class #include <iostream> using namespace std; class Student//class definition { // member variables int id; string name; public: // member function declared inside class // but defined outside void displayStudent(); void setDetails(int studID, string studName); }; // function definition outside the class // using scope resolutor :: void Student::displayStudent(){ cout << "Name: " << name << endl; cout << "ID: " << id << endl; } // function definition outside the class void Student::setDetails(int studID, string studName){ id = studID; name = studName; } int main() { Student s1; s1.setDetails(1, "Naman"); s1.displayStudent(); }
Output
Name: Naman ID: 1
Accessing private member function of a class
- When a member function is public we can access that member fun directly by using (.) membership operator anywhere
- Example cube_obj.Len will be valid if len is public
- However, a private member variable can not be accessed using dot(.) membership operator
- Example : cube_obj.breadth will be invalid if breadth private
Hence A private member function is accessed by only another public function of the same class within the body of the class
Example:-
#include <iostream> using namespace std; class Cube { // member variables double len; double breadth; public: double height; // member function declared and defined // inside class double getVolume(){ return len * breadth * height; } // setters : to set values declared and defined // inside class void setLen(int l){ len = l; } void setBreadth(int b){ breadth = b; } void setHeight(int h){ height = h; } // getter functions created to get values int getLen(){ return len; } int getBreadth(){ return breadth; } }; int main(){ Cube cube_obj1; cube_obj1.setLen(10.0); cube_obj1.setBreadth(10.0); cube_obj1.setHeight(10.0); cout << "The Volume : " << cube_obj1.getVolume() << endl; cout << "The height : " << cube_obj1.height << endl; // will be able to access private variables // using these public member functions (getters) cout << "The breadth : " << cube_obj1.getBreadth() << endl; cout << "The Length : " << cube_obj1.getLen() << endl; }
Output
The Volume : 1000 The height : 10 The breadth : 10 The Length : 10
Prime Course Trailer
Related Banners
Get PrepInsta Prime & get Access to all 200+ courses offered by PrepInsta in One Subscription
Get over 200+ course One Subscription
Courses like AI/ML, Cloud Computing, Ethical Hacking, C, C++, Java, Python, DSA (All Languages), Competitive Coding (All Languages), TCS, Infosys, Wipro, Amazon, DBMS, SQL and others
Login/Signup to comment